How to Add Dimension to NumPy Array
-
Add Dimension to NumPy Array With the
numpy.expand_dims()
Function -
Add Dimension to NumPy Array With the
numpy.newaxis
Function in Python
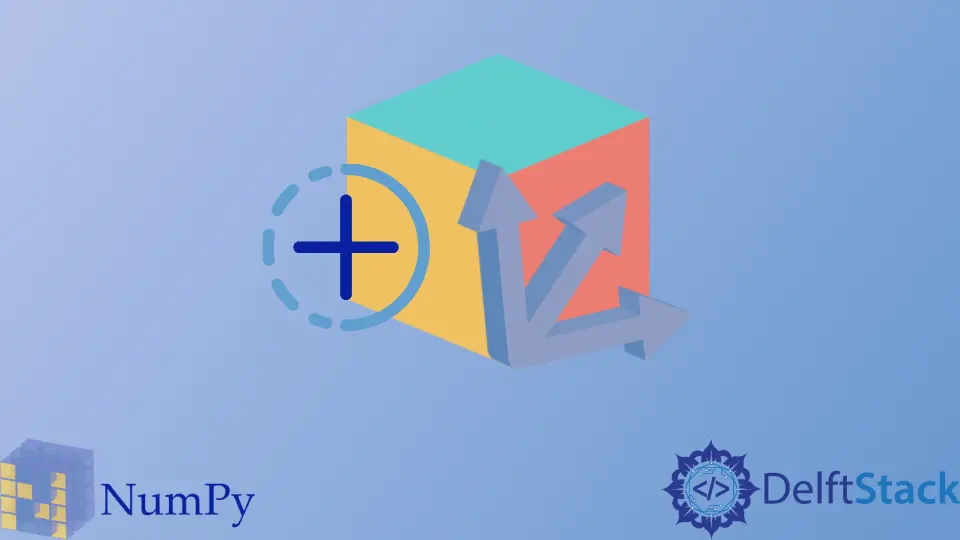
This tutorial will introduce the methods to add a new dimension to a NumPy array in Python.
Add Dimension to NumPy Array With the numpy.expand_dims()
Function
The numpy.expand_dims()
function adds a new dimension to a NumPy array. It takes the array to be expanded and the new axis as arguments and returns a new array with extra dimensions. We can specify the axis to be expanded inside the axis
parameter of the numpy.expand_dims()
function. See the following code example.
import numpy as np
array = np.array([1, 2, 3])
print(array.shape)
array = np.expand_dims(array, axis=0)
print(array.shape)
array = np.append(array, [[4, 5, 6]], axis=0)
print(array)
Output:
(3,)
(1, 3)
[[1 2 3]
[4 5 6]]
In the above code, we first created a 1D array array
with the np.array()
function and printed the shape of the array
with the array.shape
property. We then converted the array
to a 2D array with the np.expand_dims(array, axis=0)
function and printed the new shape of the array
with the array.shape
property. In the end, we appended new elements to the array
with the np.append()
function and printed the elements of the array
.
Add Dimension to NumPy Array With the numpy.newaxis
Function in Python
The previous approach does the job and works fine for now. The only problem is that the previous method has been deprecated and will probably not work with Python’s newer versions in the future. The numpy.newaxis
method can also be used to achieve the same goal as the previous method but with even lesser code and complexity. With this method, we also don’t have to worry about not being supported in Python’s later versions. The numpy.newaxis
method adds a new dimension to our array in Python.
import numpy as np
array = np.array([1, 2, 3])
print(array.shape)
array = array[np.newaxis]
print(array.shape)
array = np.append(array, [[4, 5, 6]], axis=0)
print(array)
Output:
(3,)
(1, 3)
[[1 2 3]
[4 5 6]]
We converted the array
to a 2D array with the array[np.newaxis]
method and printed the new shape of the array
with the array.shape
property. In the end, we appended new elements to the array
with the np.append()
function and printed the elements of the array
.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn