How to Calculate Absolute Value in NumPy
-
Calculate Absolute Value With the
abs()
Function in Python -
Calculate Absolute Value With the
numpy.absolute()
Function -
Calculate Absolute Value With the
numpy.abs()
Function
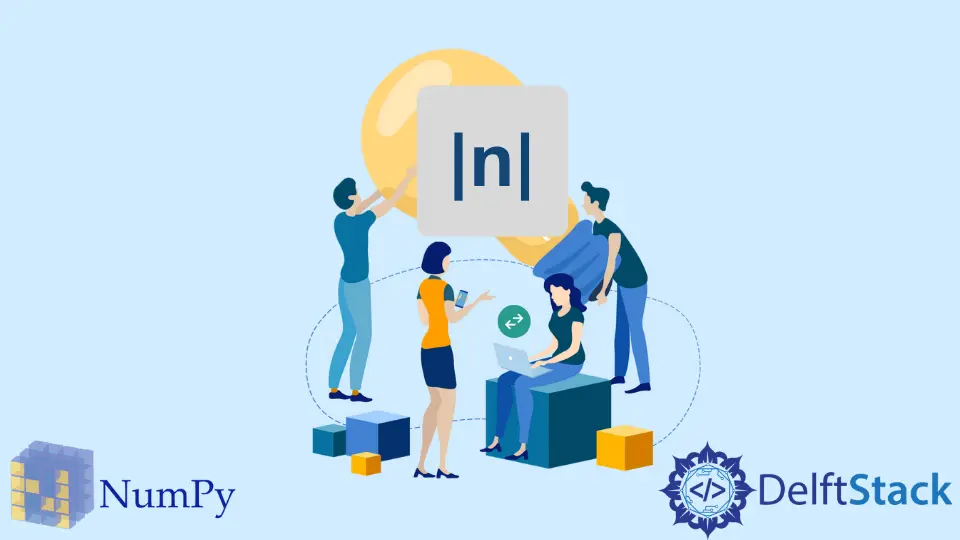
This tutorial will introduce the methods to calculate the absolute value in Python.
Calculate Absolute Value With the abs()
Function in Python
The absolute value of a number is calculated by taking the square root of its square. The abs()
function is a built-in function that can be used to find the absolute value of a number in Python. The following code example shows us how we can use the abs()
function to find the absolute value in Python.
import numpy as np
x1 = np.array([-1, -2])
print(abs(x1))
Output:
[1, 2]
In the above code, We calculated the absolute value of the variable x1
with the abs()
function in Python.
Calculate Absolute Value With the numpy.absolute()
Function
We can also use the numpy.absolute()
function inside the NumPy
package to calculate the absolute value. See the following code example.
import numpy as np
x1 = np.array([-1, -2])
print(np.absolute(x1))
Output:
[1, 2]
Calculate Absolute Value With the numpy.abs()
Function
There is also a abs()
function inside the NumPy
package just like the abs()
function in core-python that can be used to calculate the absolute value. The following code example shows us how we can calculate the absolute value of a numpy array with the numpy.abs()
function in Python.
import numpy as np
x1 = np.array([-1, -2])
print(np.abs(x1))
Output:
[1, 2]
All the functions discussed above are exactly the same and do the same thing. There are no differences in any of the methods discussed above. We can use any of the above-mentioned methods to find the absolute value of a number in Python.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn