How to Fill Array With Value in NumPy
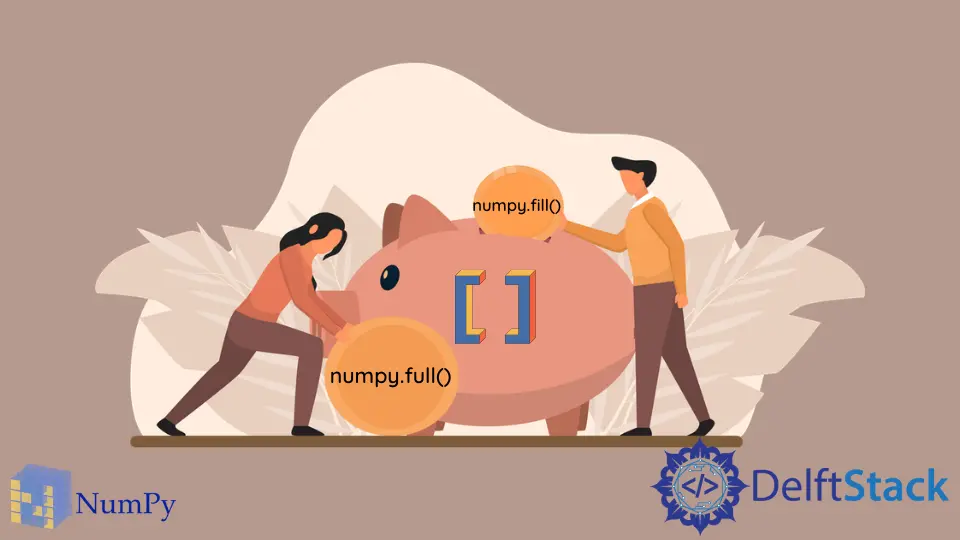
When working with numerical data in Python, NumPy is an essential library that provides powerful tools for handling arrays. One common task is filling an array with a specific value. Whether you need to initialize an array for calculations or simply want to reset values, knowing how to do this efficiently is crucial.
In this article, we will explore three primary methods for filling a NumPy array with a certain value: using the numpy.full()
function, the numpy.fill()
method, and a simple for loop. Each method has its own use cases and advantages, so let’s dive in and understand how to implement them effectively.
Using numpy.full() Function
The numpy.full()
function is one of the most straightforward ways to create an array filled with a specific value. This function takes two main arguments: the shape of the array and the fill value. It returns a new array with the specified shape, filled entirely with the given value.
Here’s how you can use numpy.full()
:
import numpy as np
array_shape = (3, 4)
fill_value = 7
filled_array = np.full(array_shape, fill_value)
print(filled_array)
Output:
[[7 7 7 7]
[7 7 7 7]
[7 7 7 7]]
In this example, we first import the NumPy library and define the shape of our array as a tuple (3, 4)
, meaning we want a 3x4 array. We set the fill_value
to 7. The np.full()
function then creates a new array of the specified shape, filled entirely with the value 7. This method is highly efficient and is particularly useful when you need to create arrays of a fixed size with a default value.
Using numpy.fill() Method
Another method to fill an existing NumPy array with a specific value is by using the numpy.fill()
method. Unlike numpy.full()
, which creates a new array, numpy.fill()
modifies an existing array in place. This can be particularly useful when you want to reset the values of an array without creating a new one.
Here’s how to use the numpy.fill()
method:
import numpy as np
existing_array = np.array([[1, 2, 3], [4, 5, 6]])
fill_value = 0
existing_array.fill(fill_value)
print(existing_array)
Output:
[[0 0 0]
[0 0 0]]
In this example, we start with an existing array containing some values. We then use the fill()
method to set all the elements of this array to 0. This method is efficient and memory-friendly, especially when dealing with large datasets. Since it modifies the array in place, it avoids the overhead of creating a new array, which can be beneficial in performance-sensitive applications.
Using a for
Loop
While the previous methods are more efficient and straightforward, sometimes you might want to fill an array using a for loop. This approach gives you more control and flexibility, especially if you need to apply more complex logic while filling the array.
Here’s an example of how to fill an array using a for loop:
import numpy as np
array_shape = (2, 3)
fill_value = 5
filled_array = np.empty(array_shape)
for i in range(array_shape[0]):
for j in range(array_shape[1]):
filled_array[i][j] = fill_value
print(filled_array)
Output:
[[5. 5. 5.]
[5. 5. 5.]]
In this example, we first create an empty array with the desired shape using np.empty()
. Then, we use nested for loops to iterate through each element of the array and assign the fill_value
of 5. This method is more flexible, allowing you to implement complex filling logic if needed, but it’s generally less efficient than the previous methods, especially for large arrays.
Conclusion
Filling a NumPy array with a specific value is a fundamental operation that can be accomplished in several ways. The numpy.full()
function is ideal for creating new arrays, while the numpy.fill()
method is perfect for modifying existing ones. If you need more control or wish to implement custom logic, using a for loop is also an option. Understanding these methods will enhance your ability to manipulate arrays effectively in your numerical computations.
FAQ
-
What is the difference between numpy.full() and numpy.fill()?
numpy.full() creates a new array filled with a specified value, while numpy.fill() modifies an existing array in place. -
Can I fill a multi-dimensional array using these methods?
Yes, both numpy.full() and numpy.fill() can be used to fill multi-dimensional arrays with a specified value. -
Is using a for loop to fill an array efficient?
While using a for loop provides flexibility, it is generally less efficient than using numpy.full() or numpy.fill() for large arrays. -
How do I fill an array with random values?
You can use numpy.random.rand() or numpy.random.randint() to fill an array with random values instead of a fixed value. -
Are there any performance considerations when filling arrays?
Yes, using numpy methods like full() and fill() is generally more efficient than using a for loop, especially for large datasets.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn