Element-Wise Division in Python NumPy
-
NumPy Element-Wise Division With
numpy.divide()
Function -
NumPy Element-Wise Division With the
/
Operator
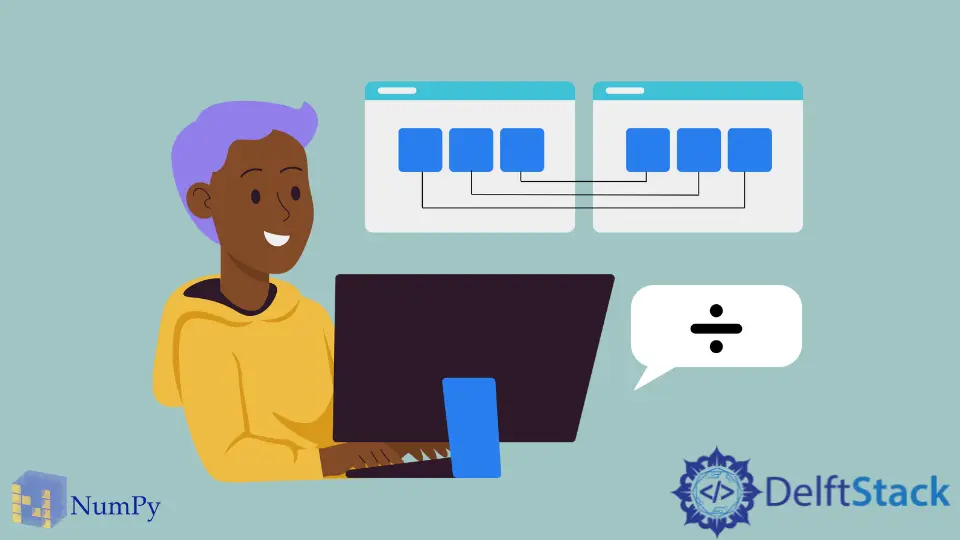
This tutorial will introduce the methods to carry out an element-wise division on NumPy arrays in Python.
NumPy Element-Wise Division With numpy.divide()
Function
If we have two arrays and want to divide each element of the first array with each element of the second array, we can use the numpy.divide()
function. The numpy.divide()
function performs element-wise division on NumPy arrays. The numpy.divide()
function takes the dividend array, the divisor array, and the output array as its arguments and stores the division’s results inside the output array. See the following code example.
import numpy as np
array1 = np.array([10, 20, 30])
array2 = np.array([2, 4, 6])
np.divide(array1, array2, array3)
print(array3)
Output:
[5. 5. 5.]
In the above code, we first created the two NumPy arrays, the dividend array array1
, and the divisor array array2
with the np.array()
function. We then divided the array1
by the array2
and stored the results inside the NumPy array array3
with the np.divide()
function.
NumPy Element-Wise Division With the /
Operator
We can also use the /
operator to carry out element-wise division on NumPy arrays in Python. The /
operator is a shorthand for the np.true_divide()
function in Python. We can use the /
operator to divide one array by another array and store the results inside a third array. See the following code example.
import numpy as np
array1 = np.array([10, 20, 30])
array2 = np.array([2, 4, 6])
array3 = array1 / array2
print(array3)
Output:
[5. 5. 5.]
We divided the array1
by the array2
and stored the results inside the NumPy array array3
with the /
operator.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn