Covariance in Python NumPy
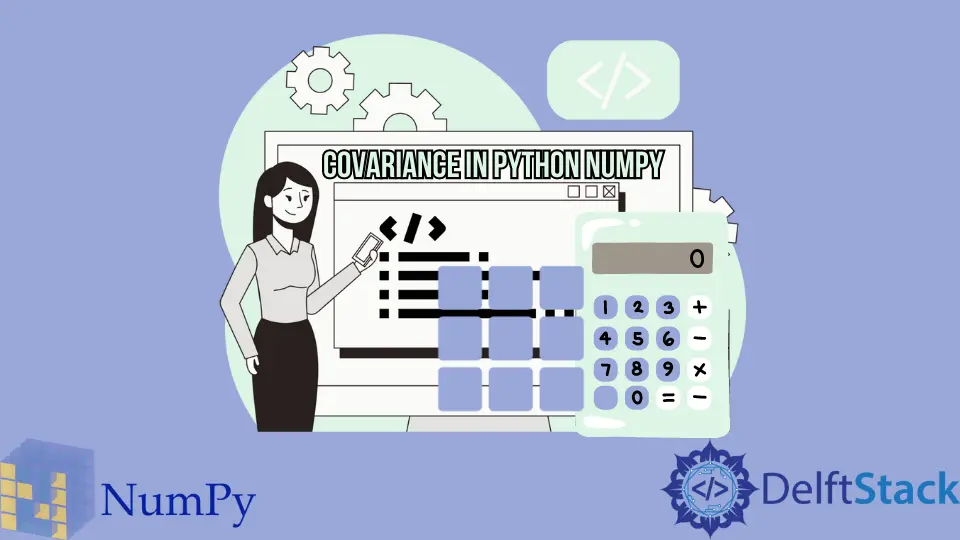
This tutorial will introduce the method to calculate the covariance between two NumPy arrays in Python.
Covariance With the numpy.cov()
Function
In statistics, covariance is the measure of change in one variable with the change in the other variable. Covariance tells us how much one variable changes if another variable is changed. We can calculate the covariance between two NumPy arrays with the numpy.cov(a1, a2)
function in Python.
Here, a1
represents a collection of values of the first variable, and a2
represents a collection of values of the second variable. The numpy.cov()
function returns a 2D array in which the value at index [0][0]
is the covariance between a1
and a1
, the value at index [0][1]
is the covariance between a1
and a2
, the value at index [1][0]
is the covariance between a2
and a1
, and the value at index [1][1]
is the covariance between a2
and a2
. See the following code example.
import numpy as np
array1 = np.array([1, 2, 3])
array2 = np.array([2, 4, 5])
covariance = np.cov(array1, array2)[0][1]
print(covariance)
Output:
1.5
We first created the two NumPy arrays array1
and array2
with the np.array()
function. Then we calculated the covariance with the np.cov(array1, array2)[0][1]
and saved the result in the covariance
variable. In the end, we printed the value inside the covariance
variable.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn