How to Transpose a 1D Array in NumPy
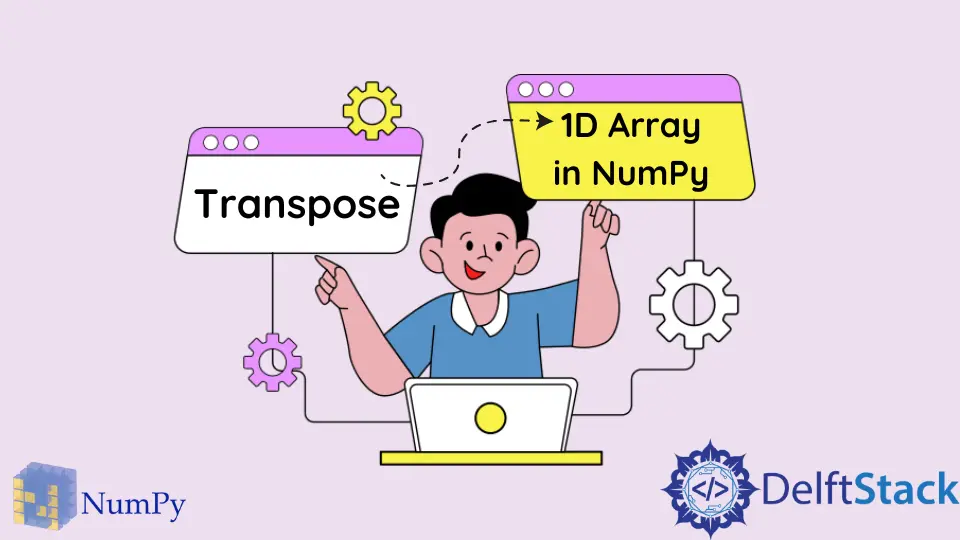
Arrays and matrices form the core of this Python library. Transposes of these arrays and matrices play a critical role in some subjects, such as machine learning. In NumPy, it’s straightforward to calculate the transpose of an array or a matrix.
Transpose a 1D array in NumPy
To transpose an array or matrix in NumPy, we have to use the T
attribute that stores the transposed array or matrix.
T
attribute is exclusive to NumPy arrays, that is, ndarray
only. This attribute is invalid for Python lists.
Theoretically, it’s possible to transpose a 1D array, but technically, or more precisely, in terms of programming languages, it is not possible to transpose a 1D array.
Don’t get me wrong with this statement. It is just that transposing a 1D array is a bit different in Python or any other programming language. It all boils down to how arrays are represented in programming languages.
A 1D array is simply a row of a matrix. If we have to transpose this array, technically matrix, we must convert this 1D matrix to a 2D matrix. And then transpose the 2-D matrix using designated functions.
Refer to the following code for a better explanation.
import numpy as np
a = [1, 2, 3, 4, 5]
b = np.array(a)
c = np.array([a])
print(b)
print(c)
print(b.shape)
print(c.shape)
print(b.T)
print(c.T)
Output:
[1 2 3 4 5]
[[1 2 3 4 5]]
(5,)
(1, 5)
[1 2 3 4 5]
[[1]
[2]
[3]
[4]
[5]]
First, we form two NumPy arrays, b
is 1D and c
is 2D, using the np.array()
method and a Python list. To convert the list to a 2D matrix, we wrap it around by []
brackets. Then we print the NumPy arrays and their respective shapes.
But the most important thing to note is that the transpose of the 1D array is the same as the array itself, but the transpose of the 2D array is wholly changed. And the results are pretty obvious.
The non-transposed 2D array has an array within it with five elements representing a row of the matrix. When transposed, there were five arrays inside the 2D array representing five rows of the transposed matrix with one element in each row. And that’s how transposing works!
Whereas, in the case of the 1D array, the same array was returned because the transposed array of [1 2 3 4 5]
in Python looks like this [[1] [2] [3] [4] [5]]
. And this result requires our original array to be 2D instead of 1D.