NumPy Meshgrid 3D
- Understanding the Basics of NumPy Meshgrid
- Method 1: Creating a Basic 3D Meshgrid
- Method 2: Customizing the 3D Meshgrid
- Method 3: Using the Meshgrid for Function Evaluation
- Conclusion
- FAQ
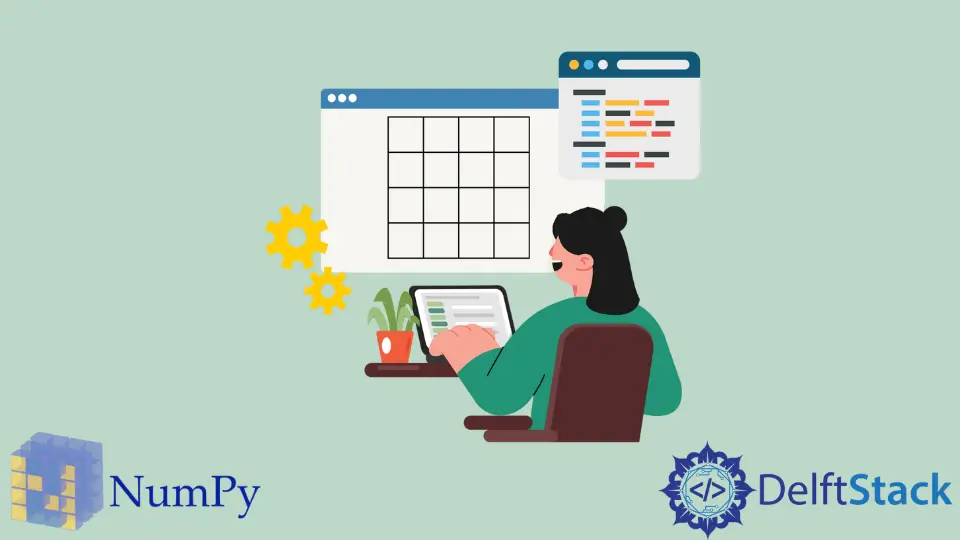
Creating a three-dimensional meshgrid is an essential skill for anyone working with numerical simulations, data visualizations, or scientific computing in Python. The NumPy library, a cornerstone of the Python ecosystem, provides powerful tools to generate meshgrids that can be utilized in various applications, including 3D plotting and surface generation.
In this tutorial, we will delve into the process of creating a 3D meshgrid using NumPy. Whether you are a beginner or looking to refresh your knowledge, this guide will provide you with clear examples and explanations to help you master this technique.
Understanding the Basics of NumPy Meshgrid
Before we dive into the code, let’s clarify what a meshgrid is. A meshgrid is a grid of coordinates that allows for the evaluation of functions over a multi-dimensional space. In 3D, this means generating a grid that spans three axes: X, Y, and Z. NumPy’s meshgrid
function is designed to create these grids efficiently, enabling you to work with multi-dimensional data seamlessly.
To illustrate how to create a 3D meshgrid, we will use NumPy’s meshgrid
function combined with a range of values for each axis. Let’s get started with the first method.
Method 1: Creating a Basic 3D Meshgrid
In this method, we will use NumPy’s meshgrid
function to create a basic 3D meshgrid. We will define a range of values for each axis and then generate the meshgrid.
import numpy as np
x = np.linspace(-5, 5, 10)
y = np.linspace(-5, 5, 10)
z = np.linspace(-5, 5, 10)
X, Y, Z = np.meshgrid(x, y, z)
print("X grid:")
print(X)
print("\nY grid:")
print(Y)
print("\nZ grid:")
print(Z)
Output:
X grid:
[[[-5. -5. -5. ... -5. -5. -5.]
[-5. -5. -5. ... -5. -5. -5.]
...
[ 5. 5. 5. ... 5. 5. 5.]]
[[-5. -5. -5. ... -5. -5. -5.]
[-5. -5. -5. ... -5. -5. -5.]
...
[ 5. 5. 5. ... 5. 5. 5.]]
...
[[-5. -5. -5. ... -5. -5. -5.]
[-5. -5. -5. ... -5. -5. -5.]
...
[ 5. 5. 5. ... 5. 5. 5.]]]
Y grid:
[[[-5. -5. -5. ... -5. -5. -5.]
[-5. -5. -5. ... -5. -5. -5.]
...
[ 5. 5. 5. ... 5. 5. 5.]]
[[-5. -5. -5. ... -5. -5. -5.]
[-5. -5. -5. ... -5. -5. -5.]
...
[ 5. 5. 5. ... 5. 5. 5.]]
...
[[-5. -5. -5. ... -5. -5. -5.]
[-5. -5. -5. ... -5. -5. -5.]
...
[ 5. 5. 5. ... 5. 5. 5.]]]
Z grid:
[[[-5. -5. -5. ... -5. -5. -5.]
[-5. -5. -5. ... -5. -5. -5.]
...
[ 5. 5. 5. ... 5. 5. 5.]]
[[-5. -5. -5. ... -5. -5. -5.]
[-5. -5. -5. ... -5. -5. -5.]
...
[ 5. 5. 5. ... 5. 5. 5.]]
...
[[-5. -5. -5. ... -5. -5. -5.]
[-5. -5. -5. ... -5. -5. -5.]
...
[ 5. 5. 5. ... 5. 5. 5.]]]
In this example, we first import NumPy and define three ranges of values for the X, Y, and Z axes using np.linspace
. The meshgrid
function then takes these ranges and generates three 3D arrays: X
, Y
, and Z
. Each of these arrays corresponds to the coordinates of the grid points in 3D space. The output shows the X, Y, and Z grids, which can be used for further calculations or visualizations.
Method 2: Customizing the 3D Meshgrid
In this method, we will explore how to customize our meshgrid by changing the resolution and the ranges of the axes. This can be useful for specific applications where you need finer control over the grid points.
import numpy as np
x = np.linspace(-10, 10, 20)
y = np.linspace(-10, 10, 20)
z = np.linspace(-10, 10, 20)
X, Y, Z = np.meshgrid(x, y, z)
print("X grid with customized range:")
print(X)
print("\nY grid with customized range:")
print(Y)
print("\nZ grid with customized range:")
print(Z)
Output:
X grid with customized range:
[[[-10. -10. -10. ... -10. -10. -10.]
[-10. -10. -10. ... -10. -10. -10.]
...
[ 10. 10. 10. ... 10. 10. 10.]]
[[-10. -10. -10. ... -10. -10. -10.]
[-10. -10. -10. ... -10. -10. -10.]
...
[ 10. 10. 10. ... 10. 10. 10.]]
...
[[-10. -10. -10. ... -10. -10. -10.]
[-10. -10. -10. ... -10. -10. -10.]
...
[ 10. 10. 10. ... 10. 10. 10.]]
Y grid with customized range:
[[[-10. -10. -10. ... -10. -10. -10.]
[-10. -10. -10. ... -10. -10. -10.]
...
[ 10. 10. 10. ... 10. 10. 10.]]
[[-10. -10. -10. ... -10. -10. -10.]
[-10. -10. -10. ... -10. -10. -10.]
...
[ 10. 10. 10. ... 10. 10. 10.]]
...
[[-10. -10. -10. ... -10. -10. -10.]
[-10. -10. -10. ... -10. -10. -10.]
...
[ 10. 10. 10. ... 10. 10. 10.]]
Z grid with customized range:
[[[-10. -10. -10. ... -10. -10. -10.]
[-10. -10. -10. ... -10. -10. -10.]
...
[ 10. 10. 10. ... 10. 10. 10.]]
[[-10. -10. -10. ... -10. -10. -10.]
[-10. -10. -10. ... -10. -10. -10.]
...
[ 10. 10. 10. ... 10. 10. 10.]]
...
[[-10. -10. -10. ... -10. -10. -10.]
[-10. -10. -10. ... -10. -10. -10.]
...
[ 10. 10. 10. ... 10. 10. 10.]]]
In this example, we modified the range of values for each axis to span from -10 to 10, while also increasing the number of points to 20. This results in a finer meshgrid, providing more detailed coordinates for 3D visualizations. The output reveals the updated grids, which can be particularly beneficial when you require a more precise representation of a function or surface in three dimensions.
Method 3: Using the Meshgrid for Function Evaluation
Now that we have our meshgrid, let’s see how we can use it to evaluate a mathematical function in 3D space. This is particularly useful in visualizing surfaces, such as a sine wave or a paraboloid.
import numpy as np
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
x = np.linspace(-5, 5, 100)
y = np.linspace(-5, 5, 100)
X, Y = np.meshgrid(x, y)
Z = np.sin(np.sqrt(X**2 + Y**2))
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
ax.plot_surface(X, Y, Z, cmap='viridis')
plt.show()
In this code, we first create a meshgrid for the X and Y axes using a finer range of values. We then compute the Z values by applying the sine function to the square root of the sum of squares of X and Y. The plot_surface
function from Matplotlib is then used to visualize the resulting surface in 3D. The output is a beautiful sine wave pattern that showcases the capabilities of using meshgrids for function evaluation.
Conclusion
In this tutorial, we’ve explored how to create and utilize 3D meshgrids using NumPy in Python. From generating basic grids to customizing them and evaluating functions, these techniques are invaluable for anyone working in scientific computing or data visualization. By mastering NumPy’s meshgrid function, you can significantly enhance your ability to analyze and visualize complex data in three dimensions.
FAQ
-
what is a meshgrid in NumPy?
A meshgrid in NumPy is a grid of coordinates that allows for the evaluation of functions over multiple dimensions, typically used in 2D and 3D plotting. -
how do I create a 3D meshgrid in Python?
You can create a 3D meshgrid in Python using NumPy’s meshgrid function by defining ranges for the X, Y, and Z axes. -
can I customize the resolution of a meshgrid?
Yes, you can customize the resolution of a meshgrid by adjusting the number of points in thelinspace
function for each axis.
-
how can I visualize a 3D meshgrid?
You can visualize a 3D meshgrid using libraries like Matplotlib, which provides functions to plot surfaces and scatter points in three dimensions. -
what are some applications of 3D meshgrids?
Applications of 3D meshgrids include scientific simulations, data visualization, surface plotting, and evaluating mathematical functions.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn