How to Get Column of NumPy Array
- Method 1: Basic Slicing
-
Method 2: Using the
np.take
Function - Method 3: Boolean Indexing
- Conclusion
- FAQ
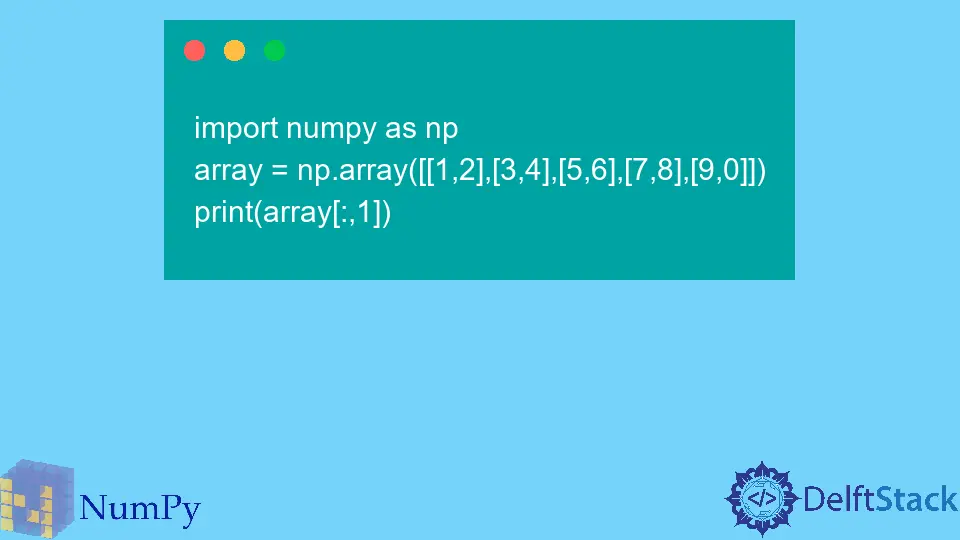
NumPy is an essential library for anyone working with numerical data in Python. If you’re dealing with multidimensional arrays, you might often need to extract specific columns for analysis or manipulation. Fortunately, NumPy offers a straightforward way to do this using basic slicing methods.
In this article, we will explore different techniques to retrieve a column from a NumPy array, complete with clear examples and explanations. Whether you’re a beginner or an experienced programmer, understanding how to slice arrays effectively will enhance your data manipulation skills. Let’s dive into the world of NumPy and learn how to get that column you need!
Once you have NumPy imported, you can create arrays in various dimensions. For example, a 2D array can be created like this:
data = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
Output:
[[1 2 3]
[4 5 6]
[7 8 9]]
This array consists of three rows and three columns, and it serves as a perfect example for our column extraction techniques.
Method 1: Basic Slicing
The most straightforward way to extract a column from a NumPy array is through basic slicing. This method allows you to specify the row and column indices you want to retrieve. In a 2D array, you can access a specific column by using the syntax array[:, column_index]
. Here’s how it works:
column_1 = data[:, 0]
Output:
[1 4 7]
In this example, we are retrieving the first column of the data
array. The :
operator indicates that we want all rows, while 0
specifies that we want the first column (remember that indexing starts at zero in Python). The resulting output is a one-dimensional array containing the elements of the first column.
This method is not only simple but also efficient, making it a go-to choice for many data manipulation tasks. You can easily adjust the column_index
to extract any other column you need. For instance, to get the second column, you would use data[:, 1]
, and to get the third, data[:, 2]
.
Method 2: Using the np.take
Function
Another method to extract a column from a NumPy array is by utilizing the np.take
function. This function allows you to specify the axis along which you want to extract elements. It can be particularly useful when you want to retrieve multiple columns at once. Here’s how to use np.take
to get a single column:
column_2 = np.take(data, 1, axis=1)
Output:
[2 5 8]
In this code snippet, we use np.take
to extract the second column (index 1) from the data
array. The axis=1
parameter indicates that we are working along the columns. The result is a one-dimensional array containing the elements from the second column.
What makes np.take
advantageous is its flexibility. You can easily modify it to extract multiple columns by passing a list of indices. For example, if you wanted to extract both the first and second columns, you could do something like this:
columns_1_and_2 = np.take(data, [0, 1], axis=1)
Output:
[[1 2]
[4 5]
[7 8]]
This would give you a 2D array containing the specified columns, making it a versatile option for more complex data extraction needs.
Method 3: Boolean Indexing
Boolean indexing is another powerful method to extract columns from a NumPy array. This technique allows you to create a mask based on conditions, which can then be used to filter the data. While this method is often used for row selection, you can also apply it to columns. Here’s how to use boolean indexing to extract a specific column:
mask = np.array([True, False, False])
column_3 = data[mask, 2]
Output:
[3 6 9]
In this example, we first create a boolean mask that indicates which rows we want to select. The mask [True, False, False]
means we only want the first row. By using this mask along with the column index 2
, we retrieve the third column of the data
array.
While boolean indexing might seem a bit more complex than the previous methods, it offers great flexibility. You can create more intricate conditions based on your dataset, allowing you to extract data that meets specific criteria. For instance, if you wanted to extract values from the third column where the corresponding values in the first column are greater than 2, you could do something like this:
mask = data[:, 0] > 2
column_filtered = data[mask, 2]
Output:
[6 9]
This results in a filtered array containing values from the third column, but only for rows where the first column’s value is greater than 2.
Conclusion
Extracting columns from a NumPy array is a fundamental skill for anyone working with data in Python. Whether you choose basic slicing, the np.take
function, or boolean indexing, each method offers unique advantages. By mastering these techniques, you can efficiently manipulate your datasets and perform analyses tailored to your needs. As you continue to work with NumPy, you’ll find that these skills will significantly enhance your data processing capabilities.
FAQ
-
How do I extract multiple columns from a NumPy array?
You can use thenp.take
function and pass a list of column indices, or you can slice the array using the syntaxarray[:, [col_index_1, col_index_2]]
. -
What is the difference between basic slicing and boolean indexing?
Basic slicing allows you to access specific rows and columns directly using their indices, while boolean indexing uses a mask to filter rows based on conditions.
-
Can I extract a column from a 3D NumPy array?
Yes, you can extract a column from a 3D array by specifying the appropriate indices for the dimensions you want to access. -
Is it possible to extract a column without using NumPy?
While you can use Python lists to achieve similar results, NumPy is optimized for performance and should be preferred for large datasets. -
What are some common applications of extracting columns from NumPy arrays?
Common applications include data analysis, machine learning preprocessing, and statistical computations.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn