How to Convert Float Array to Int Array in NumPy
-
Convert a 2D Array From Float to Int Using
ndarray.astype()
in NumPy -
Convert a 2D Array From Float to Int Using
ndarray.asarray()
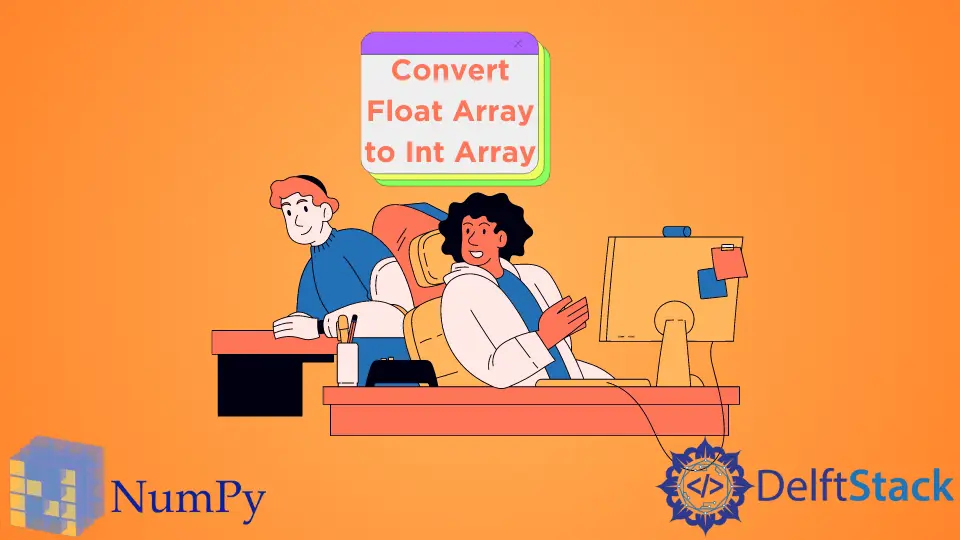
Often, we have to convert float values to integer values for a variety of use cases. Similar is the case with Python arrays and NumPy arrays.
Using some functions from NumPy
, we can easily convert 2D float NumPy arrays into 2D integer NumPy arrays.
In this article, we will talk about two such methods, ndarray.astype()
and numpy.asarray()
.
Convert a 2D Array From Float to Int Using ndarray.astype()
in NumPy
NumPy arrays are of type ndarray
. These objects have in-built functions, and one such function is astype()
. This function is used to create a copy of a NumPy array of a specific type. This method accepts five arguments, namely, dtype
, order
, casting
, subok
, and copy
. dtype
refers to the data type of the copied array. order
is an optional argument and it controls the memory layout of the resulting array. All the other options are optional.
To learn more about the other parameters of this function, refer to the official documentation of this function here
Refer to the following code to understand this function better.
import numpy as np
myArray = np.array(
[[1.0, 2.5, 3.234, 5.99, 99.99999], [0.3, -23.543, 32.9999, 33.0000001, -0.000001]]
)
myArray = myArray.astype(int)
print(myArray)
Output:
[[ 1 2 3 5 99]
[ 0 -23 32 33 0]]
Convert a 2D Array From Float to Int Using ndarray.asarray()
Secondly, we can use the asarray()
function. This function accepts four arguments, a
, dtype
, order
, and like
.
a
refers to the input array that has to be converted.dtype
refers to the data type to which the array has to be converted. Interestingly,dtype
is an optional argument, and its default value is inferred from the input itself.order
andlike
are also other optional arguments.order
refers to the output array’s memory layout.
To learn more about the arguments of this function, refer to the official documentation of this function here
import numpy as np
myArray = np.array([[1.923, 2.34, 23.134], [-24.000001, 0.000001, -0.000223]])
myArray = np.asarray(myArray, dtype=int)
print(myArray)
Output:
[[ 1 2 23]
[-24 0 0]]
In the above code, the data type is mention as int
, and the output array is also an integer NumPy array.