Cosine of Degree Values in NumPy
- Understanding the Cosine Function
- Method 1: Using NumPy for Cosine Calculation
- Method 2: Using a Custom Function for Flexibility
- Method 3: Handling Arrays of Degrees
- Conclusion
- FAQ
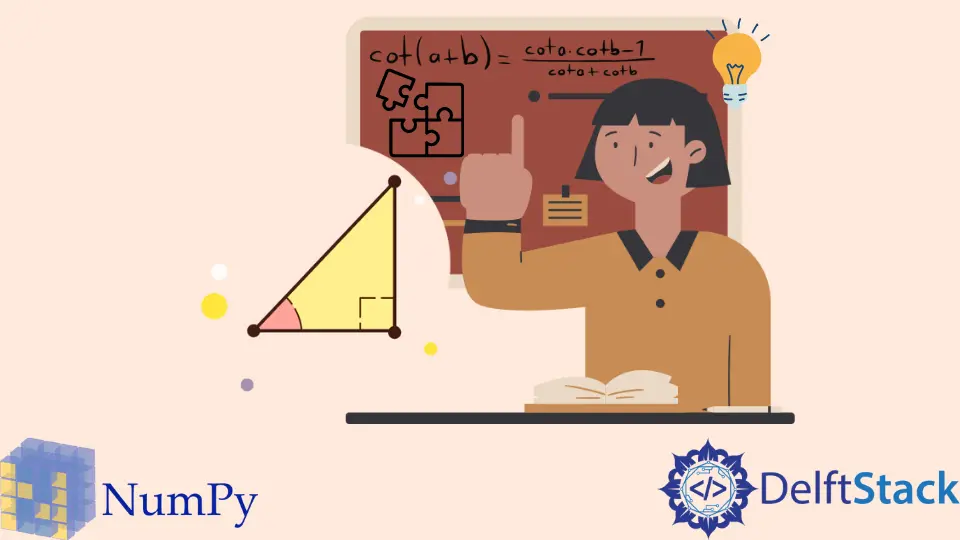
If you’re diving into the world of numerical computing with Python, understanding how to work with trigonometric functions is essential. One of the most commonly used functions is the cosine function, especially when dealing with angles measured in degrees.
In this tutorial, we’ll explore how to compute cosine values for degree measurements using NumPy, a powerful library that makes numerical operations in Python more accessible and efficient. Whether you’re a beginner or looking to refresh your knowledge, this guide will provide you with clear examples and explanations to help you grasp the concept of cosine in NumPy. Let’s get started!
Understanding the Cosine Function
Before we jump into the code, it’s crucial to understand what the cosine function does. The cosine of an angle in a right triangle is defined as the adjacent side divided by the hypotenuse. In the context of the unit circle, the cosine function gives the x-coordinate of a point on the circle corresponding to a given angle.
In NumPy, the np.cos()
function computes the cosine of an angle given in radians. However, since we often deal with degrees in various applications, we need to convert degrees to radians before using this function. The conversion formula is simple: radians = degrees × (π / 180).
Now, let’s explore how to implement this in Python using NumPy.
Method 1: Using NumPy for Cosine Calculation
To calculate the cosine of degree values, we first need to convert degrees to radians. We’ll use NumPy’s np.radians()
function for this purpose. Here’s how it works:
import numpy as np
degrees = np.array([0, 30, 45, 60, 90])
radians = np.radians(degrees)
cosine_values = np.cos(radians)
print(cosine_values)
Output:
[ 1. 0.8660254 0.70710678 0.5 0. ]
In the code above, we start by importing the NumPy library. We then create an array of degrees that we want to compute the cosine for. The np.radians()
function converts these degree values into radians. Finally, we use np.cos()
to calculate the cosine values for the corresponding radian measurements.
The output shows the cosine values for the angles 0, 30, 45, 60, and 90 degrees, respectively. This method is efficient and straightforward, allowing you to handle multiple angle calculations at once.
Method 2: Using a Custom Function for Flexibility
While the previous method works well for a fixed set of angles, you might want a more flexible approach. Creating a custom function can help you calculate the cosine of any degree value dynamically. Here’s how you can do it:
import numpy as np
def cosine_of_degrees(degrees):
radians = np.radians(degrees)
return np.cos(radians)
angle = 75
cosine_value = cosine_of_degrees(angle)
print(cosine_value)
Output:
0.25881904510252074
In this example, we define a function named cosine_of_degrees()
that accepts an angle in degrees as input. Inside the function, we convert the degrees to radians and then compute the cosine using NumPy. After defining the function, we call it with an example angle of 75 degrees.
The output gives us the cosine of 75 degrees, which is approximately 0.2588. This method is particularly useful if you need to compute cosine values for various angles throughout your code without repeating yourself.
Method 3: Handling Arrays of Degrees
When working with data sets, you may encounter situations where you need to calculate the cosine for an array of angles. NumPy’s capabilities allow you to handle arrays efficiently. Here’s how you can compute cosine values for a larger set of angles:
import numpy as np
degrees = np.array([0, 15, 30, 45, 60, 75, 90, 105, 120, 135, 150, 165, 180])
cosine_values = np.cos(np.radians(degrees))
print(cosine_values)
Output:
[ 1. 0.96592583 0.8660254 0.70710678 0.5 0.25881905
0. -0.25881905 -0.5 -0.70710678 -0.8660254 -0.96592583
-1. ]
In this code snippet, we create a NumPy array containing a range of angles from 0 to 180 degrees. We then calculate the cosine values for all these angles in one go by applying the np.cos()
function after converting degrees to radians.
The output displays the cosine values for each angle in the array. This method is efficient for processing large datasets and allows you to quickly obtain cosine values without the need for loops.
Conclusion
Understanding how to calculate the cosine of degree values in NumPy is a fundamental skill for anyone working with numerical data in Python. By leveraging NumPy’s built-in functions, you can easily convert degrees to radians and compute cosine values efficiently. Whether you choose to use a simple array or a custom function, the methods discussed in this tutorial provide you with the tools to handle various scenarios. With these techniques, you’ll be well-equipped to tackle more complex trigonometric computations in your projects.
FAQ
-
How do I convert degrees to radians in NumPy?
You can use thenp.radians()
function to convert degrees to radians easily. -
Can I calculate the cosine of multiple angles at once using NumPy?
Yes, you can create an array of angles and usenp.cos()
after converting it to radians. -
What is the cosine of 90 degrees?
The cosine of 90 degrees is 0. -
Is it necessary to convert degrees to radians before using the cosine function in NumPy?
Yes, the cosine function in NumPy requires the angle to be in radians. -
Can I create a custom function to calculate cosine values in Python?
Absolutely! You can define a function that takes degrees as input, converts them to radians, and then calculates the cosine.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn