How to Get NumPy Array Length
-
Get Length of a NumPy Array With the
numpy.size
Property in Python -
Get Length of a NumPy Array With the
numpy.shape
Function in Python
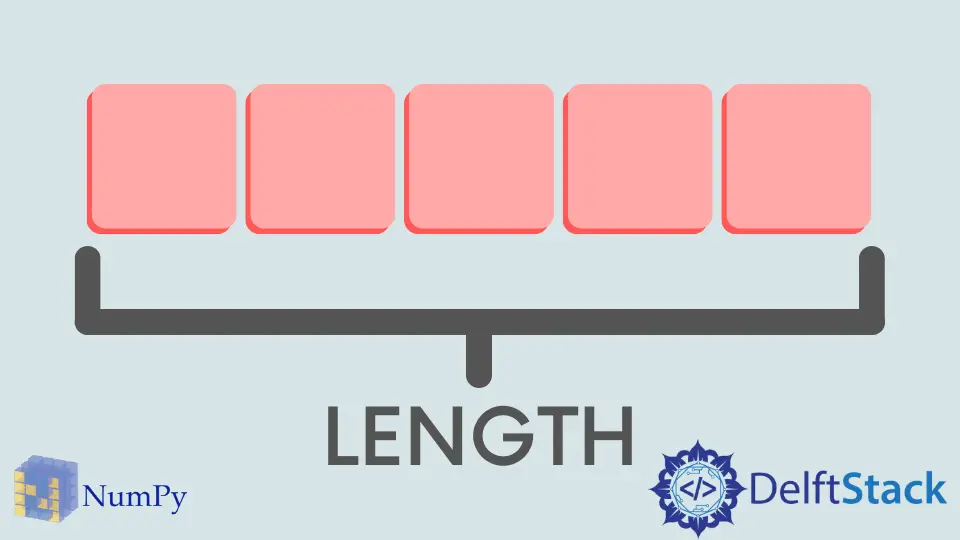
This tutorial will discuss the methods to get the length of a NumPy array.
Get Length of a NumPy Array With the numpy.size
Property in Python
The numpy.size
property gets the total number of elements in a NumPy array. We can use this property to accurately find the number of elements in a NumPy array in Python. See the following code example.
import numpy as np
array = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9])
print(array.size)
Output:
9
In the above code, we get the number of elements in the array array
with the numpy.size
property in Python. This method works great with one-dimensional arrays. It does not consider the multi-dimensional arrays; it only gives us the total number of elements in an array. It is shown in the code example below.
import numpy as np
array = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
print(array.size)
Output:
9
In the above code, we get the number of elements in the multi-dimensional array array
with the numpy.size
property in Python. It also gives us the value 9
because the total number of elements is the same as in the previous example. This is the reason why this method is not suitable for multi-dimensional arrays.
Get Length of a NumPy Array With the numpy.shape
Function in Python
If we also want to know the number of elements in each dimension of the NumPy array, we have to use the numpy.shape
function in Python. The numpy.shape
function returns a tuple in the form of (x, y)
, where x
is the number of rows in the array and y
is the number of columns in the array. We can find the total number of elements in the array like we have done in the previous section by multiplying both x
and y
with each other. See the following code example.
import numpy as np
array = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
print(array.shape)
Output:
(3, 3)
In the above code, we get the length of the multi-dimensional array array
with the numpy.shape
function in Python. We can now find the total number of elements by multiplying the values in the tuple with each other. This method is preferred over the previous method because it gives us the number of rows and columns.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn