How to Add Column in NumPy
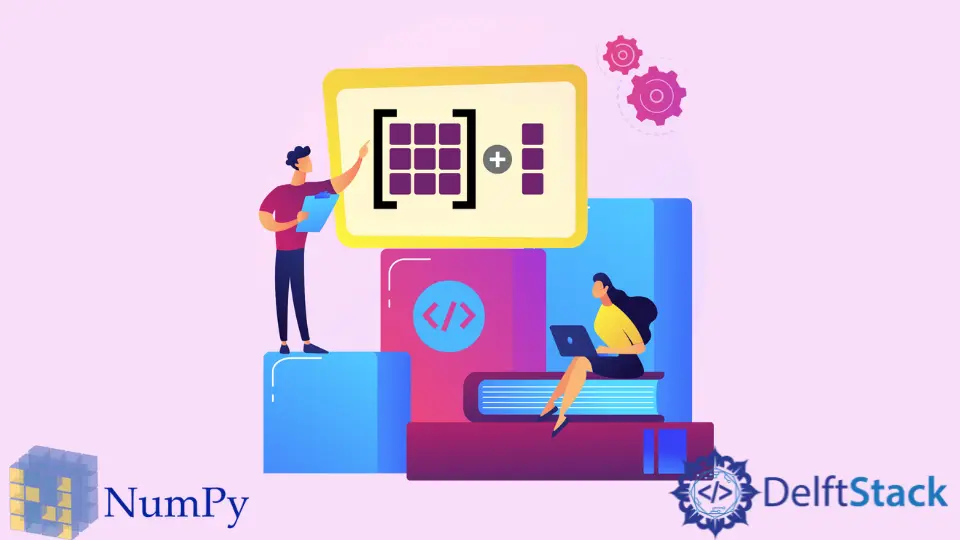
This tutorial will introduce the method to add a column to a NumPy array in Python.
Add Column to a NumPy Array With the numpy.append()
Function
The numpy.append()
function can be used to add an extra column to an existing numpy array. The numpy.append()
function takes three parameters, the pre-existing array, the new values to be added, and the axis
by which we want to append the new values to the pre-existing array. We can specify the axis
parameter to 1
if we want to add new columns to the pre-existing array. The numpy.append()
function returns a new array in which the new values are appended at the end of the pre-existing array. See the following code example.
import numpy as np
array = np.array([[1, 2], [3, 4], [5, 6]])
array2 = np.append(array, [[1], [1], [1]], axis=1)
array2
Output:
[[1 2 1]
[3 4 1]
[5 6 1]]
In the above code, we first initialized our first array array
with the numpy.array()
function in Python. We then initialized a new array, array2
, containing an extra column, with the numpy.append()
function in Python.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn