How to Normalize a Vector in Python
- Use the Mathematical Formula to Normalize a Vector in Python
-
Use the
numpy.linalg.norm()
Function to Normalize a Vector in Python -
Use the
sklearn.preprocessing.normalize()
Function to Normalize a Vector in Python
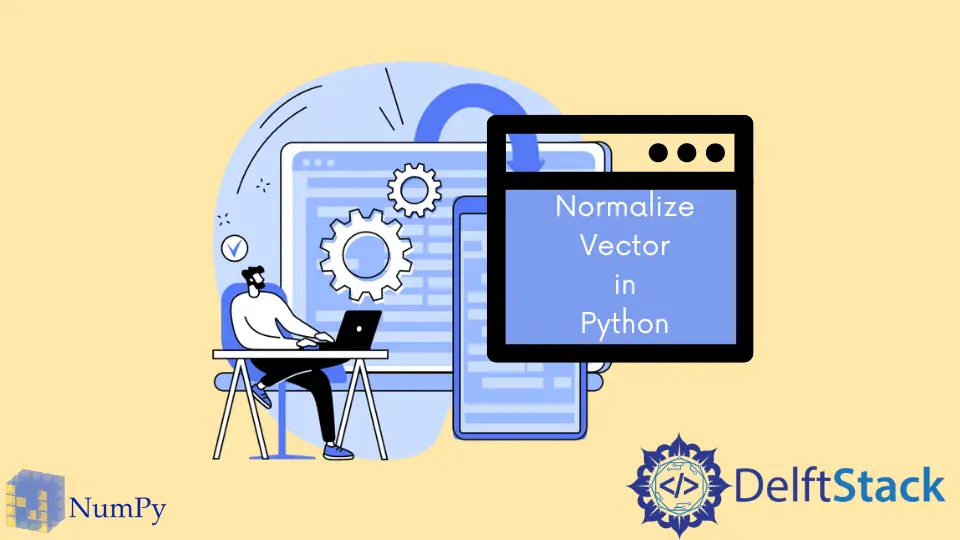
A prevalent notion in the world of machine learning is to normalize a vector or dataset before passing it to the algorithm.
When we talk about normalizing a vector, we say that its vector magnitude is 1, as a unit vector.
In this tutorial, we will convert a numpy array to a unit vector.
Use the Mathematical Formula to Normalize a Vector in Python
In this method, we will compute the vector norm of an array using the mathematical formula. When we divide the array with this norm vector, we get the normalized vector. The following code implements this.
import numpy as np
v = np.random.rand(10)
normalized_v = v / np.sqrt(np.sum(v ** 2))
print(normalized_v)
Output:
[0.10366807 0.05821296 0.11852538 0.42957961 0.27653372 0.36389277
0.47575824 0.32059888 0.2721495 0.41856126]
Note that this method will return some error if the length of the vector is 0.
Use the numpy.linalg.norm()
Function to Normalize a Vector in Python
The NumPy
module in Python has the linalg.norm()
function that can return the array’s vector norm. Then we divide the array with this norm vector to get the normalized vector. For example, in the code below, we will create a random array and find its normalized form using this method.
import numpy as np
v = np.random.rand(10)
normalized_v = v / np.linalg.norm(v)
print(normalized_v)
Output:
[0.10881785 0.32038649 0.51652046 0.05670539 0.12873248 0.52460815
0.32929967 0.32699446 0.0753471 0.32043046]
Use the sklearn.preprocessing.normalize()
Function to Normalize a Vector in Python
The sklearn
module has efficient methods available for data preprocessing and other machine learning tools. The normalize()
function in this library is usually used with 2-D matrices and provides the option of L1 and L2 normalization. The code below will use this function with a 1-D array and find its normalized form.
import numpy as np
from sklearn.preprocessing import normalize
v = np.random.rand(10)
normalized_v = normalize(v[:, np.newaxis], axis=0).ravel()
print(normalized_v)
Output:
[0.19361438 0.36752554 0.26904722 0.10672546 0.32089067 0.48359538
0.01824837 0.47591181 0.26439268 0.33180998]
The ravel()
method used in the above method is used to flatten a multi-dimensional array in Python.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn