How to Multiply Array With Scalar in Python
-
Multiply Elements of an Array With a Scalar Using
*
in Python -
Multiply an Array With a Scalar Using the
numpy.multiply()
Function in Python
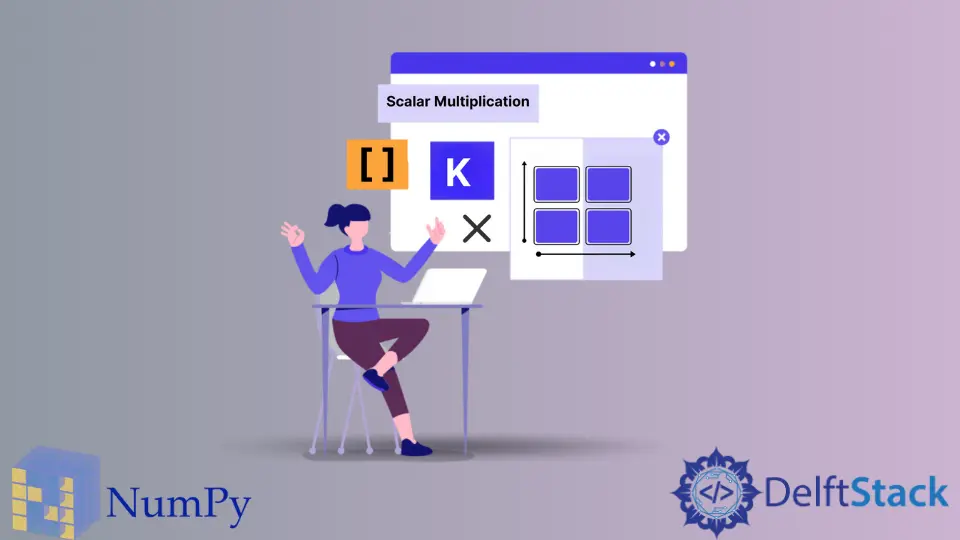
This tutorial will introduce methods to multiply elements of a NumPy array with a scalar in Python.
Multiply Elements of an Array With a Scalar Using *
in Python
In Python, it is very simple to multiply all the elements of a NumPy
array with a scalar. The *
operator in the NumPy
package can be used for this operation.
The following code example shows us how we can use the *
method to multiply all the elements of a NumPy
array with a scalar in Python.
import numpy
arr = numpy.array([1, 2, 3])
newarr = arr * 3
print(newarr)
Output:
[3 6 9]
In the above code, we first initialize a NumPy
array using the numpy.array()
function and then compute the product of that array with a scalar using the *
operator.
Multiply an Array With a Scalar Using the numpy.multiply()
Function in Python
We can multiply a NumPy array with a scalar using the numpy.multiply()
function. The numpy.multiply()
function gives us the product of two arrays. numpy.multiply()
returns an array which is the product of two arrays given in the arguments of the function.
The following code example shows us how to use the numpy.multiply()
function to multiply all the elements of a NumPy array with a scalar in Python.
import numpy
arr = numpy.array([1, 2, 3])
newarr = numpy.multiply(arr, 3)
print(newarr)
Output:
[3 6 9]
In the above code, we first initialize a NumPy
array using numpy.array()
function and then compute the product of that array with a scalar using the numpy.multiply()
function.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn