How to Convert List to NumPy Array in Python
- Understanding NumPy Arrays
- Method 1: Using the np.array() Function
- Method 2: Converting a List of Lists to a 2D NumPy Array
- Method 3: Specifying the Data Type
- Conclusion
- FAQ
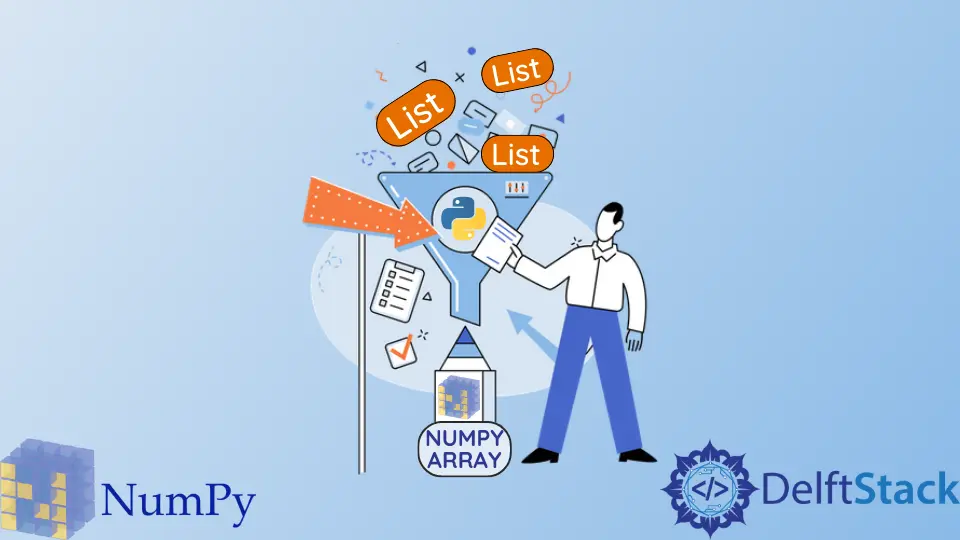
Converting a list to a NumPy array in Python is a common task that many data scientists and programmers encounter. Whether you’re working with numerical data for machine learning, scientific computing, or data analysis, understanding how to manipulate data structures effectively is crucial. NumPy arrays offer significant advantages over Python lists, including enhanced performance for mathematical operations and the ability to perform element-wise computations.
In this tutorial, we’ll guide you through the process of converting a list to a NumPy array. By the end, you’ll be equipped with the knowledge to handle data more efficiently in your Python projects.
Understanding NumPy Arrays
Before diving into the conversion process, it’s essential to understand what NumPy arrays are and why they are so widely used. NumPy, short for Numerical Python, is a powerful library that provides support for large, multi-dimensional arrays and matrices. It also includes a collection of mathematical functions to operate on these arrays.
One significant advantage of using NumPy arrays over Python lists is their ability to perform operations on entire arrays at once, rather than needing to loop through individual elements. This capability leads to cleaner code and faster execution times, especially when working with large datasets. Additionally, NumPy arrays require less memory than Python lists, making them more efficient for numerical computations.
To get started with converting a list to a NumPy array, you’ll first need to install the NumPy library if you haven’t already. You can do this using pip:
pip install numpy
Once you have NumPy installed, you can easily convert lists to arrays using the methods outlined below.
Method 1: Using the np.array() Function
The simplest and most straightforward way to convert a list to a NumPy array is by using the np.array()
function. This function takes a list (or any array-like structure) as an argument and returns a NumPy array.
Here’s how you can do it:
import numpy as np
my_list = [1, 2, 3, 4, 5]
my_array = np.array(my_list)
print(my_array)
Output:
[1 2 3 4 5]
In this example, we first import the NumPy library as np
. We then define a simple list called my_list
containing integers. By passing my_list
to the np.array()
function, we create a NumPy array called my_array
. Finally, we print the array to see the result. The output shows that the list has been successfully converted into a NumPy array.
The np.array()
function is versatile and can handle nested lists as well, converting them into multi-dimensional arrays. This feature is particularly useful when dealing with matrices or higher-dimensional data structures.
Method 2: Converting a List of Lists to a 2D NumPy Array
When dealing with more complex data, such as a list of lists, you can still use the np.array()
function to create a 2D NumPy array. This method is particularly useful for representing matrices or tabular data.
Here’s an example:
import numpy as np
my_list_of_lists = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
my_2d_array = np.array(my_list_of_lists)
print(my_2d_array)
Output:
[[1 2 3]
[4 5 6]
[7 8 9]]
In this example, we define a list of lists called my_list_of_lists
. Each inner list represents a row in a 2D array. By passing this list to the np.array()
function, we create a NumPy array called my_2d_array
. The printed output shows the 2D structure, demonstrating that the conversion was successful.
This method allows you to work with complex datasets easily. You can perform various operations on the 2D array, such as matrix multiplication, reshaping, and slicing, making it a powerful tool for data manipulation.
Method 3: Specifying the Data Type
Sometimes, you may want to specify the data type of the NumPy array explicitly. This can be crucial when working with mixed data types or when you want to optimize memory usage. The np.array()
function allows you to set the desired data type using the dtype
parameter.
Here’s an example:
import numpy as np
my_list = [1, 2, 3, 4, 5]
my_array = np.array(my_list, dtype=float)
print(my_array)
Output:
[1. 2. 3. 4. 5.]
In this code snippet, we create a list of integers and then convert it to a NumPy array with a specified data type of float
. This means that all elements in the resulting array will be of type float, even though they were originally integers. The output confirms this, showing that the integers have been converted to floating-point numbers.
Specifying the data type can be particularly helpful when you need to ensure that calculations are performed with the correct precision or when you want to save memory by using a smaller data type. NumPy supports various data types, including integers, floats, and complex numbers, allowing for flexibility in your data handling.
Conclusion
Converting a list to a NumPy array in Python is a straightforward process that can significantly enhance your data manipulation capabilities. By utilizing the np.array()
function, you can easily transform both one-dimensional and two-dimensional lists into NumPy arrays. Additionally, specifying the data type allows for greater control over memory usage and computational precision. Whether you’re working on data analysis, machine learning, or scientific computing, mastering this conversion will empower you to handle data more effectively in your Python projects.
FAQ
-
How do I install NumPy?
You can install NumPy using the commandpip install numpy
in your terminal or command prompt. -
Can I convert a nested list to a NumPy array?
Yes, you can convert a nested list (a list of lists) to a 2D NumPy array using thenp.array()
function. -
What is the advantage of using NumPy arrays over Python lists?
NumPy arrays are more efficient for numerical computations, require less memory, and allow for element-wise operations, making them ideal for data manipulation. -
Can I specify the data type of a NumPy array?
Yes, you can specify the data type using thedtype
parameter in thenp.array()
function. -
Is it possible to convert a list of mixed data types into a NumPy array?
Yes, NumPy can handle mixed data types, but it will convert them to a common data type based on the highest precision.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn