How to Find the First Index of Element in NumPy Array
-
Use the
where()
Function to Find the First Index of an Element in a NumPy Array -
Use the
nonzero()
Function to Find the First Index of an Element in a NumPy Array -
Use the
argmax()
Function to Find the First Index of an Element in a NumPy Array -
Use the
index()
Function to Find the First Index of an Element in a NumPy Array
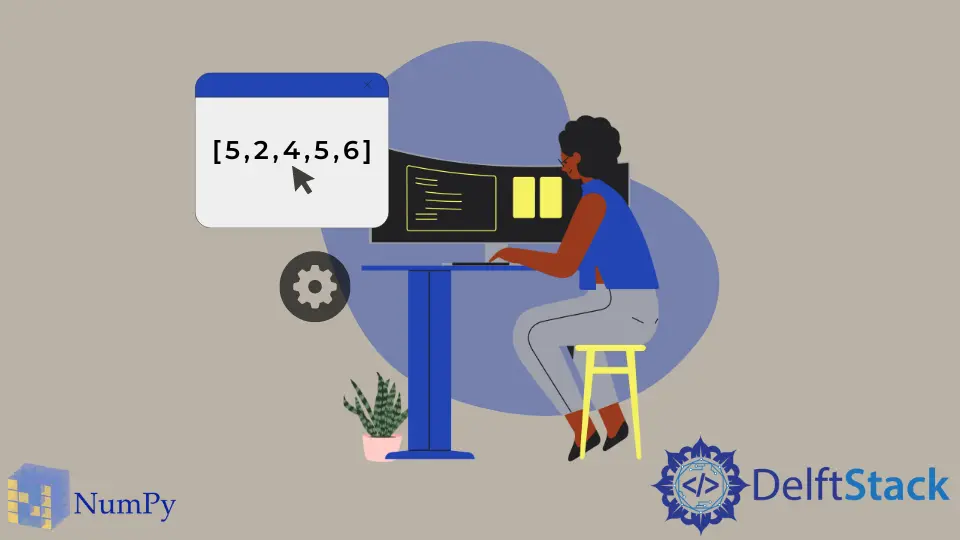
In this tutorial, we will discuss how to find the first index of an element in a numpy array.
Use the where()
Function to Find the First Index of an Element in a NumPy Array
The where()
function from the numpy module is used to return an array that contains the indices of elements that satisfy some conditions. The condition is specified within the function.
We can use it to find the first index of a specific value in an array, as shown below.
a = np.array([7, 8, 9, 5, 2, 1, 5, 6, 1])
print(np.where(a == 1)[0][0])
Output:
5
Use the nonzero()
Function to Find the First Index of an Element in a NumPy Array
The nonzero()
function returns the indices of all the non-zero elements in a numpy array. It returns tuples of multiple arrays for a multi-dimensional array.
Similar to the where()
function, we can specify the condition also so it can also return the position of a specific element.
For example,
a = np.array([7, 8, 9, 5, 2, 1, 5, 6, 1])
print(np.nonzero(a == 1)[0][0])
Output:
5
For the most basic purposes, the where()
and nonzero()
functions seem similar. The difference arises with the where()
function when you wish to pick elements of from array a
if some condition is True
and from array b
when that condition is False
.
Use the argmax()
Function to Find the First Index of an Element in a NumPy Array
The numpy.argmax()
function finds the index of the maximum element in an array. We can specify the equality condition in the function and find the index of the required element also.
For example,
a = np.array([7, 8, 9, 5, 2, 1, 5, 6, 1])
print(np.argmax(a == 1))
Output:
5
Use the index()
Function to Find the First Index of an Element in a NumPy Array
In this method, we will first convert the array to a list using the tolist()
function. Then we will use the index()
function, which will return the position of the specified element.
The following code implements this.
a = np.array([7, 8, 9, 5, 2, 1, 5, 6, 1])
print(a.tolist().index(1))
Output:
5
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn