Imaginary Numbers in NumPy Arrays
-
Use the
numpy.complex
Class to Store Imaginary Numbers in NumPy Arrays -
Use the
dtype
Parameter to Store Imaginary Numbers in NumPy Arrays
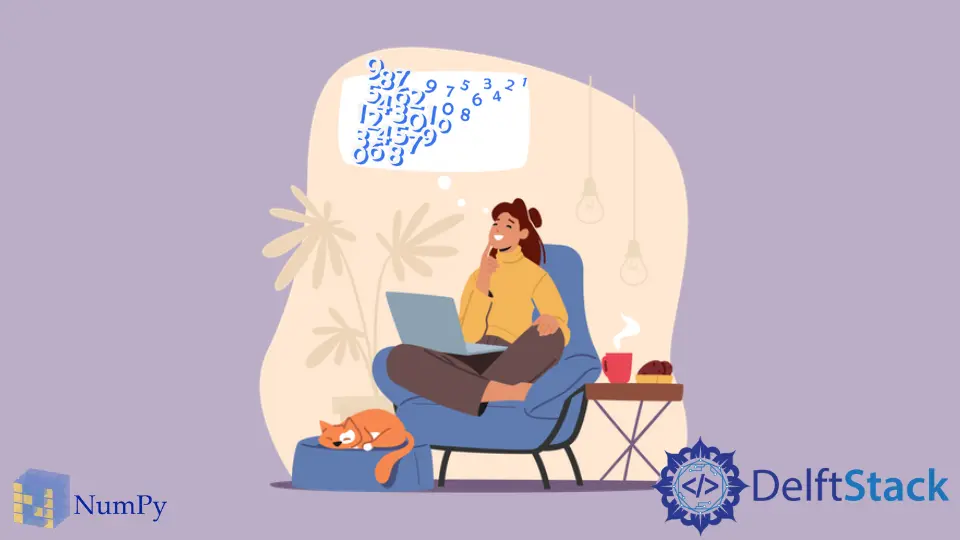
In Python, we can work with real numbers as well as imaginary numbers.
To represent a complex number, we simply add j
at the end. For example, the following string represents an imaginary number.
a = 5 + 2j
print(a, type(a))
Output:
(5+2j) <class 'complex'>
In this tutorial, we will learn how to deal with imaginary numbers in numpy arrays.
As discussed earlier, we can simply create a complex number by adding j
. We can broadcast this literal to an array using different functions like numpy.array()
, numpy.arange()
and more. See the code below for various examples of complex numbers in numpy arrays.
arr_1 = 1j * np.arange(5)
arr_2 = np.array([2 + 1j, 3 + 4j, 5 + 2j])
print(arr_1)
print(arr_2)
Output:
[0.+0.j 0.+1.j 0.+2.j 0.+3.j 0.+4.j]
[2.+1.j 3.+4.j 5.+2.j]
Use the numpy.complex
Class to Store Imaginary Numbers in NumPy Arrays
Another method of creating complex objects is using by using the complex
class provided by the numpy module. This returns a complex object which again can be stored in arrays as discussed in the previous method.
For example,
a = np.complex(1 + 1j)
c = a * np.arange(5)
print(c)
Output:
[0.+0.j 1.+1.j 2.+2.j 3.+3.j 4.+4.j]
Use the dtype
Parameter to Store Imaginary Numbers in NumPy Arrays
Another method of initiating imaginary numbers in arrays is by specifying the dtype
parameter in some numpy array functions. As we know, we can use the numpy.zeros()
and numpy.ones()
functions to create arrays of 0s and 1s, respectively. Here we can specify the dtype
parameter as complex
to get a resultant array with complex values.
The following code explains this.
z = np.ones(4, dtype=complex) * 2
print(z)
Output:
[2.+0.j 2.+0.j 2.+0.j 2.+0.j]
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn