How to Create Empty NumPy Array
-
Create an Empty NumPy Array With the
numpy.zeros()
Function -
Create an Empty NumPy Array With the
numpy.empty()
Function in Python
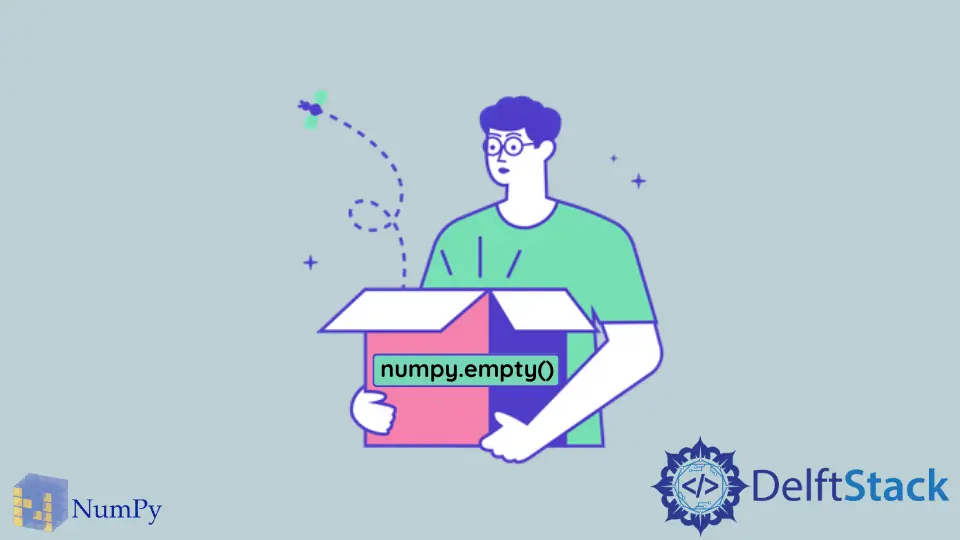
In this tutorial, we will discuss methods to create an empty NumPy array in Python.
Create an Empty NumPy Array With the numpy.zeros()
Function
The NumPy package is used to perform complex calculations on the array data structure in Python. Due to the Object-Oriented nature of Python, no variable can be truly empty. We can fill an array with zeros to call it empty in Python. The numpy.zeros()
function is used to fill an array with zeros. The following code example shows us how to create an empty array with the numpy.zeros()
function.
import numpy as np
a = np.zeros(shape=5)
print(a)
Output:
[0. 0. 0. 0. 0.]
In the above code, we created an empty array that contains five elements with the numpy.zeros()
function in Python. We specified the shape, i.e., the number of rows and columns of the array with the shape
parameter inside the numpy.zeros()
function.
Create an Empty NumPy Array With the numpy.empty()
Function in Python
We can also use the numpy.empty()
function to create an empty numpy array. Since nothing can be empty in Python, the array created by numpy.empty()
takes the existing values in the memory address allocated to it. The following code example shows us how to create an empty array with the numpy.empty()
function.
import numpy as np
a = np.empty(shape=5)
print(a)
Output:
[5.54125275e-302 0.00000000e+000 2.05226842e-289 8.73990206e+245 2.49224026e-306]
In the above code, we created an empty array that contains five elements with the numpy.empty()
function in Python. We specified the shape, i.e., the number of rows and columns of the array with the shape
parameter inside the numpy.empty()
function.
numpy.empty()
is much faster than numpy.zeros()
because it doesn’t set the array values to zero or other values. We need to set array elements to the desired values manually; otherwise, it can cause unexpected behavior.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn