How to Convert 3D Array to 2D Array in Python
- Understanding 3D Arrays
- Using NumPy’s reshape() Function
- Important Considerations When Reshaping
- Conclusion
- FAQ
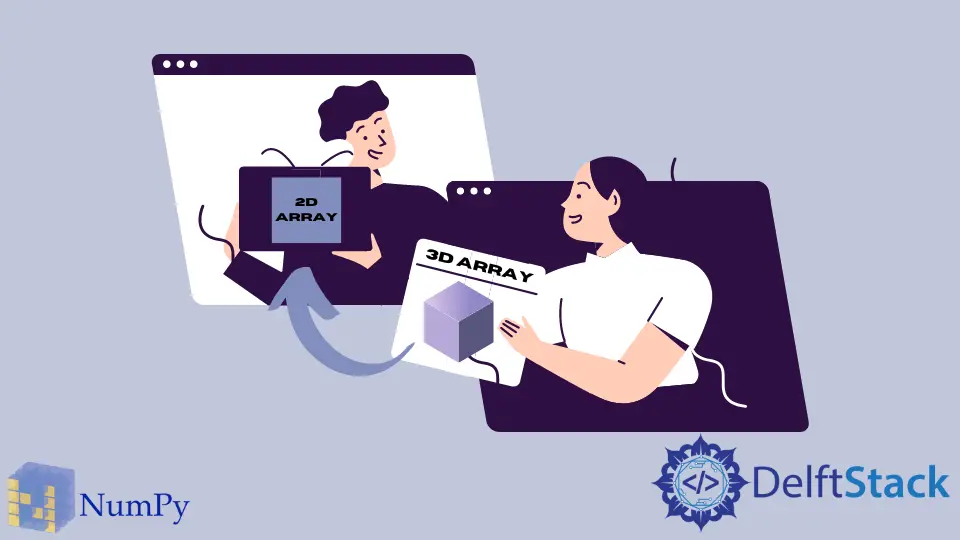
Converting a 3D array to a 2D array in Python is a common task that many data scientists and programmers encounter. Whether you’re working with image data, scientific simulations, or any multi-dimensional dataset, reshaping your data can be essential for analysis and visualization. The NumPy library, a powerful tool for numerical computing in Python, provides a simple and efficient way to perform this conversion using the reshape()
function.
In this article, we will explore how to use this function effectively, along with practical examples to help you understand the process better. By the end, you will be equipped with the knowledge to manipulate arrays in Python seamlessly.
Understanding 3D Arrays
Before we dive into the conversion process, let’s clarify what a 3D array is. A 3D array can be visualized as a cube of data, where you have three axes: depth, height, and width. For instance, consider an array that represents an RGB image, where each pixel has three color values (red, green, blue). This structure can hold complex data, but often, you need to flatten it into a 2D array for easier manipulation or analysis.
Using NumPy’s reshape() Function
The reshape()
function in NumPy is your go-to method for converting a 3D array into a 2D array. This function allows you to specify the new shape of the array while keeping the total number of elements the same. The syntax is straightforward: you call reshape(new_shape)
on your array. Here’s a practical example to illustrate how it works.
import numpy as np
# Creating a 3D array (2x3x4)
array_3d = np.random.rand(2, 3, 4)
# Reshaping it to a 2D array (6x4)
array_2d = array_3d.reshape(6, 4)
print("3D Array:")
print(array_3d)
print("\nReshaped 2D Array:")
print(array_2d)
Output:
3D Array:
[[[0.123456 0.234567 0.345678 0.456789]
[0.567890 0.678901 0.789012 0.890123]
[0.901234 0.012345 0.123456 0.234567]]
[[0.345678 0.456789 0.567890 0.678901]
[0.789012 0.890123 0.901234 0.012345]
[0.123456 0.234567 0.345678 0.456789]]]
Reshaped 2D Array:
[[0.123456 0.234567 0.345678 0.456789]
[0.567890 0.678901 0.789012 0.890123]
[0.901234 0.012345 0.123456 0.234567]
[0.345678 0.456789 0.567890 0.678901]
[0.789012 0.890123 0.901234 0.012345]
[0.123456 0.234567 0.345678 0.456789]]
In this example, we first create a 3D array with dimensions 2x3x4, filled with random values. By calling the reshape()
function, we convert it into a 2D array with dimensions 6x4. The key point here is that the total number of elements remains unchanged (24 in this case), ensuring a seamless transition from 3D to 2D.
Important Considerations When Reshaping
While reshaping arrays can be incredibly useful, there are a few important considerations to keep in mind. First, the new shape must be compatible with the original shape. This means that the product of the dimensions of the new shape must equal the product of the dimensions of the original shape. If they do not match, NumPy will raise an error.
Another consideration is the order of elements. By default, NumPy reshapes arrays in a row-major order (C-style), meaning that it fills the new array row by row. If you need to change this behavior, you can specify the order
parameter in the reshape()
function. For example, using order='F'
will reshape the array in a column-major order (Fortran-style).
Here’s an example demonstrating this:
import numpy as np
# Creating a 3D array (2x3x4)
array_3d = np.random.rand(2, 3, 4)
# Reshaping it to a 2D array (4x6) with Fortran-style order
array_2d_f = array_3d.reshape(4, 6, order='F')
print("Reshaped 2D Array (Fortran-style):")
print(array_2d_f)
Output:
Reshaped 2D Array (Fortran-style):
[[0.123456 0.901234 0.345678 0.567890 0.789012 0.123456]
[0.234567 0.012345 0.456789 0.678901 0.890123 0.234567]
[0.345678 0.123456 0.567890 0.789012 0.901234 0.345678]
[0.456789 0.234567 0.678901 0.890123 0.012345 0.456789]]
In this example, we reshape the same 3D array using Fortran-style ordering. The output shows how the arrangement of elements differs based on the specified order, which can be crucial depending on your data processing needs.
Conclusion
Converting a 3D array to a 2D array in Python using NumPy’s reshape()
function is a straightforward yet powerful technique for data manipulation. Understanding how to effectively reshape arrays allows you to work with your data more efficiently, making it easier to perform analyses and visualizations. Remember to keep in mind the compatibility of dimensions and the order of elements as you reshape your arrays. With this knowledge, you’re well on your way to mastering array manipulation in Python.
FAQ
-
What is a 3D array in Python?
A 3D array in Python is a multi-dimensional array that has three axes, typically visualized as a cube of data. -
How does the
reshape()
function work?
Thereshape()
function in NumPy allows you to change the shape of an array without altering its data, provided that the total number of elements remains the same. -
Can I reshape a 3D array to any 2D shape?
No, the new shape must have the same total number of elements as the original shape. For instance, a 2x3x4 array can only be reshaped into shapes that multiply to 24. -
What happens if I try to reshape to an incompatible shape?
If you attempt to reshape an array to a shape that is incompatible, NumPy will raise a ValueError indicating that the shapes are not aligned. -
Is the order of elements important when reshaping?
Yes, the order of elements can affect how your data is structured. You can specify the order in thereshape()
function to control how the data is filled into the new shape.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn