settimeout in Node JS
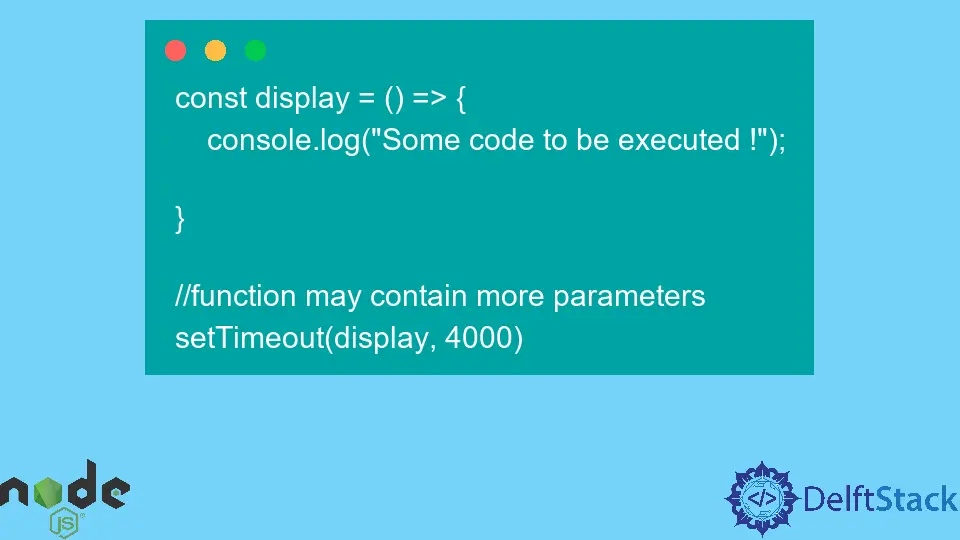
The setTimeOut()
function is an asynchronous function used to execute a piece of code after a specified amount of time.
It is one of the several ways that we can use to schedule code provided by the Node js API. This method is available both in the window object and on the server side.
However, its implementation on the server-side is different from that used in the window object.
Use the setTimeOut()
Function in NodeJS
The setTimeOut()
function is provided under the Timers
module. This module provides various methods that we can use to schedule functions to be called after some specified time.
Since the Timer object is a global object, we need not require()
anything in our code when working with the setTimeOut()
function.
The setTimeout()
function accepts several parameters this includes:
- The function is to be executed after the set time interval has elapsed.
- The time in Milliseconds that the timer will wait before executing the specified function.
- Additional optional arguments.
This function returns a positive integer
value used to identify the timer created by a call made to the function and is often referred to as a timeoutID
.
Here is the syntax of the setTimeOut()
function.
setTimeout(function [, delay, arg1, arg2, ...]);
A simple example of the setTimeOut()
function to pause execution between functions for a certain number of milliseconds.
const display =
() => {
console.log('Some code to be executed !');
}
// function may contain more parameters
setTimeout(display, 4000)
Output:
Some code to be executed !
The asynchronous nature of the setTimeOut()
is revealed when executing multiple functions that wait for each other. In the example below, the average wait time before the first function is executed is 6 seconds
.
Now in the meantime, the second function is executed. However, since the average wait time for the second function to execute is 4 seconds
, the last function is executed first, followed by the second function, and finally the first function.
setTimeout(() => {console.log('This is the first statement')}, 6000);
setTimeout(() => {console.log('This is the second statement')}, 4000);
setTimeout(() => {console.log('This is the final statement')}, 2000);
Output:
This is the final statement
This is the second statement
This is the first statement
Isaac Tony is a professional software developer and technical writer fascinated by Tech and productivity. He helps large technical organizations communicate their message clearly through writing.
LinkedIn