How to Execute Shell Script in Node.js
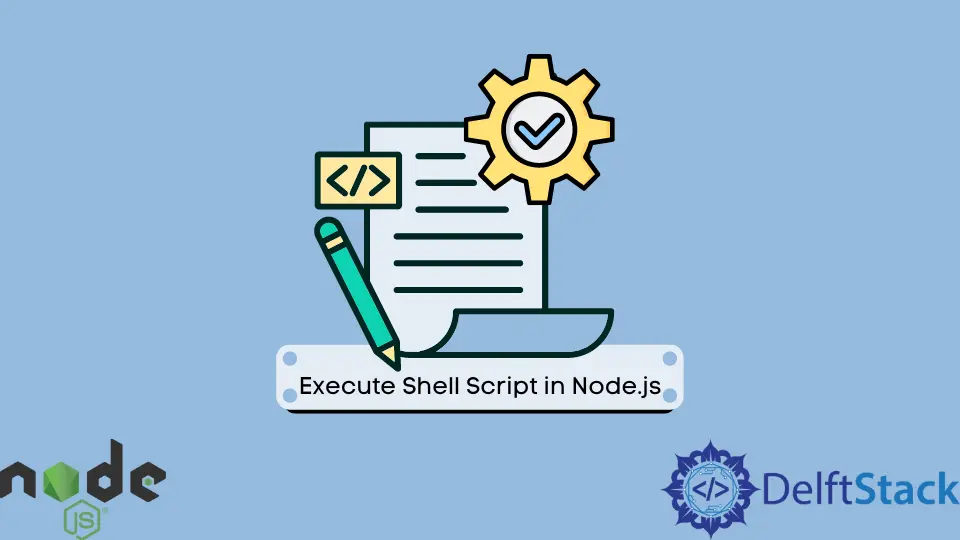
In this short article, we’ll learn how to execute the shell script using Node.js.
Use the shelljs
Module to Execute Shell Script in Node.js
On top of Node.js API, the ShellJS is a portable implementation of Unix shell commands. You could use it to remove your shell script dependency on Unix while keeping your instructions acquainted and powerful.
You can additionally install it globally to run it from outside Node tasks. You can discover more information about the ShellJS documentation for shelljs
.
Syntax:
exec(command [, options] [, callback])
The following are different options for Node.js child_process.exec()
.
async
: async meansAsynchronous
execution. It’ll be set totrue
if the callback is provided regardless of the value passed. The default value isfalse
.silent
: Don’t write program output to console. The default value isfalse
.encoding
: Encoding of the characters to be used. It affects the values returned to stdout and stderr and what is written tostdout
andstderr
when not in silent mode. The default value isutf8
.
We will focus on how to execute a shell script in Node.js using the shelljs
module.
First, install the shelljs
library using the following command.
$ npm i shelljs
The command below will check the version of npm
.
const shell = require('shelljs')
shell.exec('npm --version')
Instead of a command, you can also specify the path of your shell file.
Output:
8.1.2
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn