How to Resolve the Cannot Find Module Error in Node.js
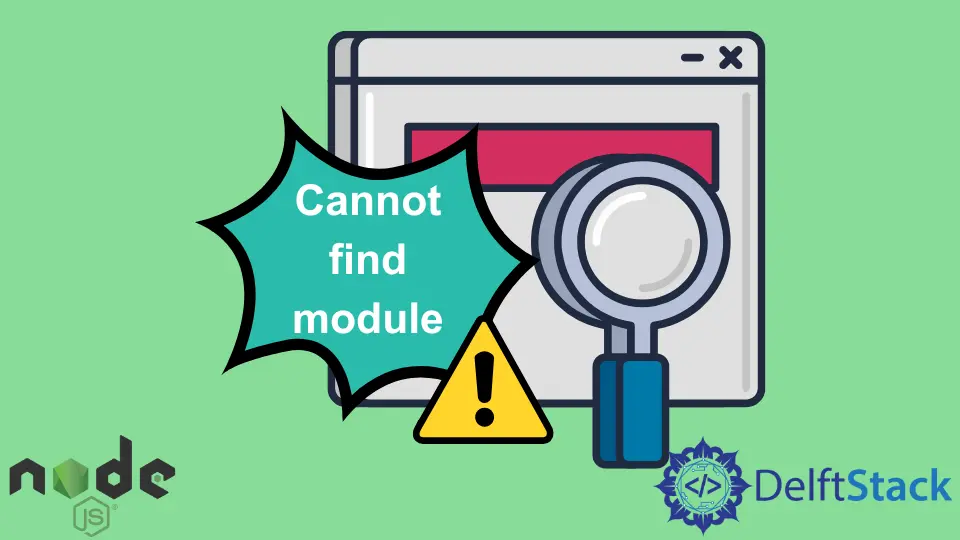
In today’s post, we will learn how to resolve the Cannot find module
error in Node.js.
the package.json
File
Before diving into the solution, we will first try understanding the package.json
file and why it is needed.
The package.json
file is any Node project’s root or heart. This file records all the important metadata information about a project that is required before publishing it in npm
.
It also defines the functional attributes of a project that uses npm
to install dependencies, run scripts, and determine the entry point to our package.
npm
is the world’s largest software registry. Open source developers on every continent use npm
to share and borrow packages, and many organizations also use npm
to manage private developments.
Resolve the Cannot find module
Error in Node.js
The Cannot find module
error occurs when the module is not installed globally. It’s a problem with the script or the npm
application.
Follow one of the solutions below to fix the problem:
-
Try to install the module in the local application folder first. Make sure you have the latest version of the module which you are trying to install by running the command:
$npm install module-name
-
Bind the existing global module to your local application. If the above solution doesn’t work, try binding a globally installed module to your application.
In your application, open the terminal and run the following command:
$npm link module
-
Delete the below files/folders from your local application.
- the
package-lock.json
file (not thepackage.json
file) - the
/node_modules
folder or run therm -rf node_modules
command in the terminal
- the
Once the above files/folders have been deleted, install the packages again by running:
$npm install
This will install all the packages with the latest version.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn