How to Encode Base64 in Node.js
- Understanding Base64 Encoding
- Encoding Strings to Base64
- Decoding Base64 to Strings
- Encoding and Decoding Files
- Decoding Base64 Files
- Conclusion
- FAQ
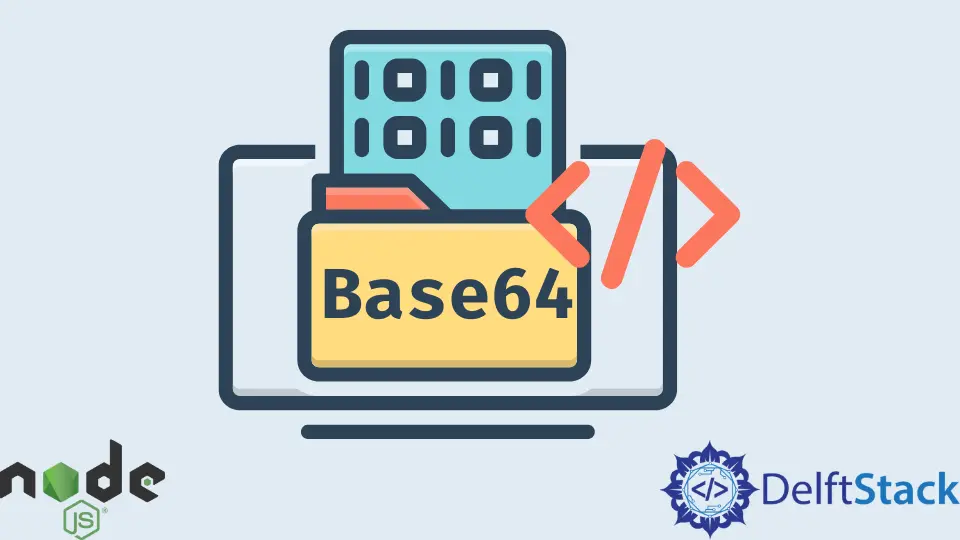
In this article, we’ll learn about how to convert strings or text into base64 in Node.js. Base64 encoding is a method used to encode binary data into an ASCII string format, making it easier to transmit over media that are designed to deal with textual data. This technique is particularly useful in web development, data storage, and when working with APIs. Whether you’re sending images, files, or simple text, knowing how to encode data in base64 can streamline your workflow. We’ll explore various methods to accomplish this in Node.js, complete with code examples that you can easily implement in your projects. Let’s dive in!
Understanding Base64 Encoding
Base64 encoding transforms binary data into a text format using a specific set of 64 characters. This encoding is commonly used in data transfer scenarios where binary data needs to be represented in a text format, such as in JSON or XML. In Node.js, the built-in Buffer
class provides an efficient way to handle base64 encoding and decoding.
When you encode a string in base64, it becomes a long string of text that can easily be transmitted or stored. The process involves taking three bytes of data and encoding them into four characters. This results in a larger output size, but the benefits of compatibility with text-based systems often outweigh this drawback.
Encoding Strings to Base64
To encode a string to base64 in Node.js, you can use the Buffer
class, which allows you to create a buffer from a string and then convert it to base64. Here’s how you can do it:
const str = "Hello, World!";
const buffer = Buffer.from(str, 'utf-8');
const base64Encoded = buffer.toString('base64');
console.log(base64Encoded);
Output:
SGVsbG8sIFdvcmxkIQ==
In this example, we start by defining a simple string, “Hello, World!”. We then create a buffer from this string using Buffer.from()
, specifying the encoding type as ‘utf-8’. After that, we call the toString()
method on the buffer and pass ‘base64’ as an argument to convert the buffer into a base64 encoded string. The output will be a string that represents the original data in base64 format.
Decoding Base64 to Strings
Decoding a base64 encoded string back to its original form is just as straightforward. You can use the same Buffer
class to achieve this. Here’s an example of how to decode a base64 string:
const base64Str = "SGVsbG8sIFdvcmxkIQ==";
const decodedBuffer = Buffer.from(base64Str, 'base64');
const decodedStr = decodedBuffer.toString('utf-8');
console.log(decodedStr);
Output:
Hello, World!
In this code snippet, we start with a base64 encoded string. Using Buffer.from()
, we create a buffer from this string, specifying ‘base64’ as the encoding type. We then convert the buffer back to a UTF-8 string using the toString()
method. This process allows us to retrieve the original string from its base64 representation.
Encoding and Decoding Files
Base64 encoding isn’t limited to strings; you can also encode files. This is particularly useful when you need to send files over a network or store them in a database. Here’s how you can encode a file in Node.js:
const fs = require('fs');
fs.readFile('example.txt', (err, data) => {
if (err) throw err;
const base64EncodedFile = data.toString('base64');
console.log(base64EncodedFile);
});
Output:
<base64-content-of-the-file>
In this example, we’re using the fs
module to read a file named ’example.txt’. The readFile
method reads the file asynchronously, and upon completion, we convert the file data to a base64 string using the toString('base64')
method. The output will be the base64 encoded content of the file, which can be used for various purposes like transmission or storage.
Decoding Base64 Files
Decoding a base64 encoded file back to its original binary format is equally simple. You can use the fs
module to write the decoded data back to a file. Here’s how to do it:
const fs = require('fs');
const base64Str = "<base64-content-of-the-file>";
const buffer = Buffer.from(base64Str, 'base64');
fs.writeFile('decoded_example.txt', buffer, (err) => {
if (err) throw err;
console.log('File has been decoded and saved!');
});
Output:
File has been decoded and saved!
In this snippet, we start with a base64 encoded string. We create a buffer from this string using Buffer.from()
, specifying ‘base64’ as the encoding type. Finally, we write the buffer to a new file called ‘decoded_example.txt’ using fs.writeFile()
. This process effectively restores the original file from its base64 representation.
Conclusion
Base64 encoding is a powerful tool in Node.js that simplifies the process of handling binary data. Whether you’re encoding strings, files, or other types of data, the Buffer
class provides a straightforward and efficient way to perform these tasks. By following the examples in this article, you can easily implement base64 encoding and decoding in your Node.js applications. This knowledge can significantly enhance your ability to work with data in various formats, making your applications more versatile and robust.
FAQ
-
What is Base64 encoding?
Base64 encoding is a method of converting binary data into a text format using a specific set of 64 characters, making it suitable for transmission over text-based systems. -
Why would I use Base64 encoding in Node.js?
Base64 encoding is useful for transmitting binary data in a text format, storing files in databases, and working with APIs that require text-based data. -
Can I encode images using Base64 in Node.js?
Yes, you can encode images and other binary files using Base64 in Node.js by reading the file data and converting it to a base64 string. -
How do I decode a Base64 string in Node.js?
You can decode a Base64 string by creating a buffer from the encoded string and then converting it back to its original format using thetoString()
method. -
Is Base64 encoding secure?
Base64 encoding is not a secure method of data encryption; it is simply a way to represent binary data in a text format. For secure data transmission, consider using encryption methods.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn