How to Encode URL in Node.js
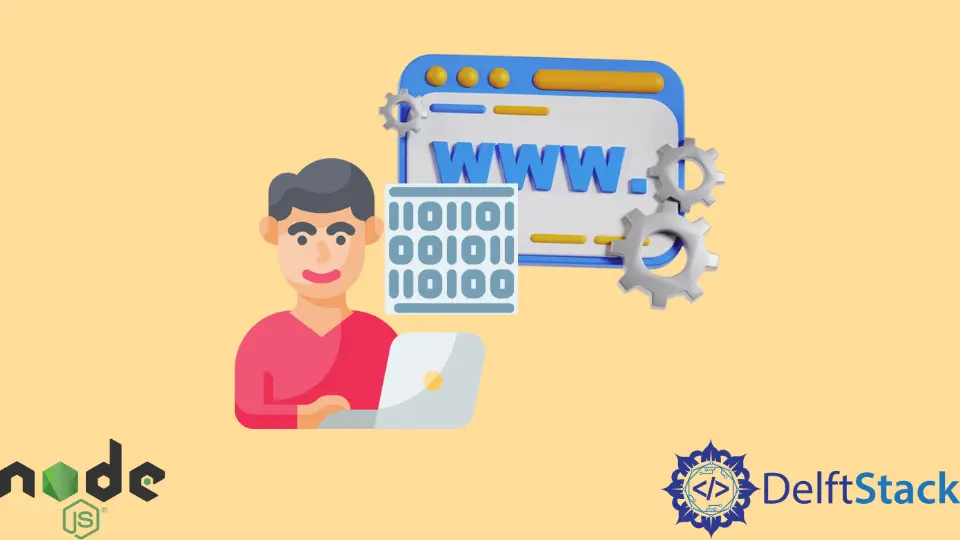
In this article, we’ll learn about how to do URL encoding in Node.js.
URI Encoding in Node.js
JavaScript offers an integrated URI encoding feature called encodeURIComponent()
. This selection encodes a URI by changing every example of tremendous characters with one, two, three, or as many as four break-out sequences representing the UTF-8
encoding of the individual.
You can use this function in any client-side or server-side application, including Node.js.
Syntax:
encodeURIComponent(uriComponent)
The uriComponent
is an obligatory parameter that can be a number, string, Boolean, undefined, null, or any object.
Before encoding, the uriComponent
receives transformed into a string. A new string is returned as an output representing the provided uriComponent
encoded as a URI element.
Use encodeURIComponent()
on person-entered fields from forms submitted to the server via query string parameters. This eliminates encodings and symbols accidentally generated during data entry for individual HTML
elements or other characters that need to be encoded/decoded.
The sole difference between encodeURI
and encodeURIComponent
is that encodeURIComponent
encodes the complete string while encodeURI
ignores the protocol prefix ("http://"
) and domain name.
Code Example:
console.log(encodeURIComponent(
'select * from Users where email = "example@domain.com"'));
console.log(encodeURIComponent('http://exampleDomain.com/?param1=hello world'));
console.log(
encodeURI('select * from Users where email = "example@domain.com"'));
console.log(encodeURI('http://exampleDomain.com/?param1=hello world'));
In the example above, the MySQL database query, and the URL query is encoded using the encodeURIComponent()
and encodeURI()
function. The main difference between encodeURIComponent()
and encodeURI()
is that the HTTP domain is encoded in the prior and later only the query parameters, and the =
and @
characters are not encoded in encodeURI()
.
Attempt to execute the above code in any compiler that supports Node.js. It’s going to display the beneath result:
Output:
select%20*%20from%20Users%20where%20email%20%3D%20%22example%40domain.com%22
http%3A%2F%2FexampleDomain.com%2F%3Fparam1%3Dhello%20world
select%20*%20from%20Users%20where%20email%20=%20%22example@domain.com%22
http://exampleDomain.com/?param1=hello%20world
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn