Multithreading in Node.js
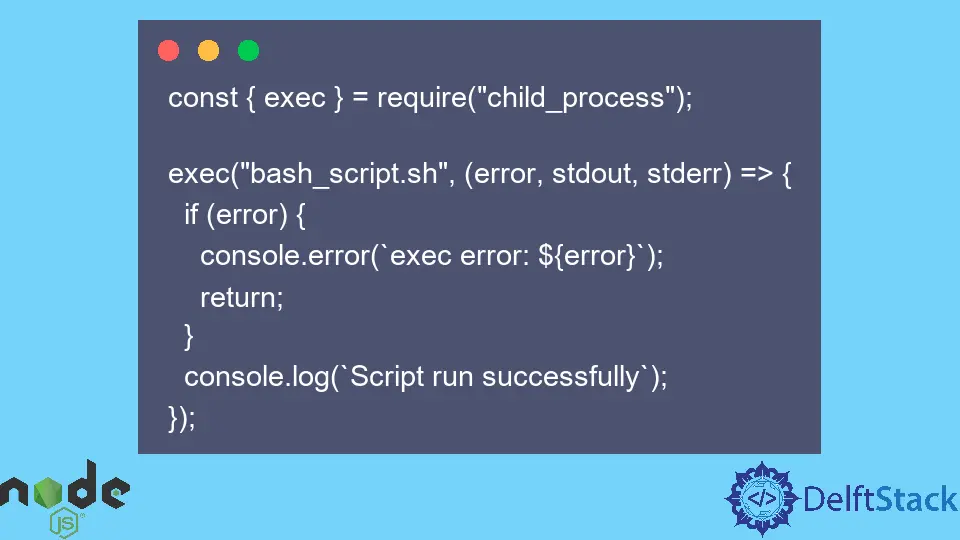
In Node.js, the term multithreading
doesn’t apply because Node.js is designed to be run in a single-threaded event loop. However, Node.js is built on top of the JavaScript language, which is single-threaded by default.
However, Node.js provides some ways to perform concurrent programming tasks. One way to do this is by using the child_process
module, which allows you to spawn new processes that can run concurrently with the main Node.js process.
Another way to perform concurrent tasks in Node.js is by using the async/await
syntax, which allows you to write asynchronous code that looks like it is synchronous. This can make it easier to write code that performs multiple tasks concurrently without having to explicitly spawn new processes or use callbacks.
For example, the following code uses async/await
to perform two tasks concurrently.
async function runTasks() {
const task1 = doTask1();
const task2 = doTask2();
await Promise.all([task1, task2]);
}
In this example, doTask1
and doTask2
are asynchronous functions that return Promises
. The await
operator is used to wait for both Promises
to resolve before continuing.
This allows the two tasks to be performed concurrently rather than sequentially. It’s worth noting that the async/await
syntax is built on top of the JavaScript Promise
type, which allows you to write asynchronous code in a way that is more like traditional synchronous code.
The child_process
module in Node.js provides an easy way to spawn new processes. It allows you to run external programs, such as Bash scripts or other executables, from within a Node.js program.
Implement Multithreading in Node.js
Here’s an example of using the child_process
module to run a Bash script.
In this example, the exec
function is used to execute a Bash script called bash_script.sh
. The function takes a callback that is called when the process finishes.
The callback receives three arguments: error
, stdout
, and stderr
. These arguments contain the error that occurred, the process’s standard output, and the process’s standard error, respectively.
The child_process
module provides several other functions for spawning processes, such as spawn
, fork
, and execFile
. Each of these functions has its own set of options and behavior, so you can choose the one that best fits your needs.
const {exec} = require('child_process');
exec('bash_script.sh', (error, stdout, stderr) => {
if (error) {
console.error(`exec error: ${error}`);
return;
}
console.log(`Script run successfully`);
});
Output:
stdout:
stderr:
Script run successfully
I am Waqar having 5+ years of software engineering experience. I have been in the industry as a javascript web and mobile developer for 3 years working with multiple frameworks such as nodejs, react js, react native, Ionic, and angular js. After which I Switched to flutter mobile development. I have 2 years of experience building android and ios apps with flutter. For the backend, I have experience with rest APIs, Aws, and firebase. I have also written articles related to problem-solving and best practices in C, C++, Javascript, C#, and power shell.
LinkedIn