How to Call REST API in Node.js
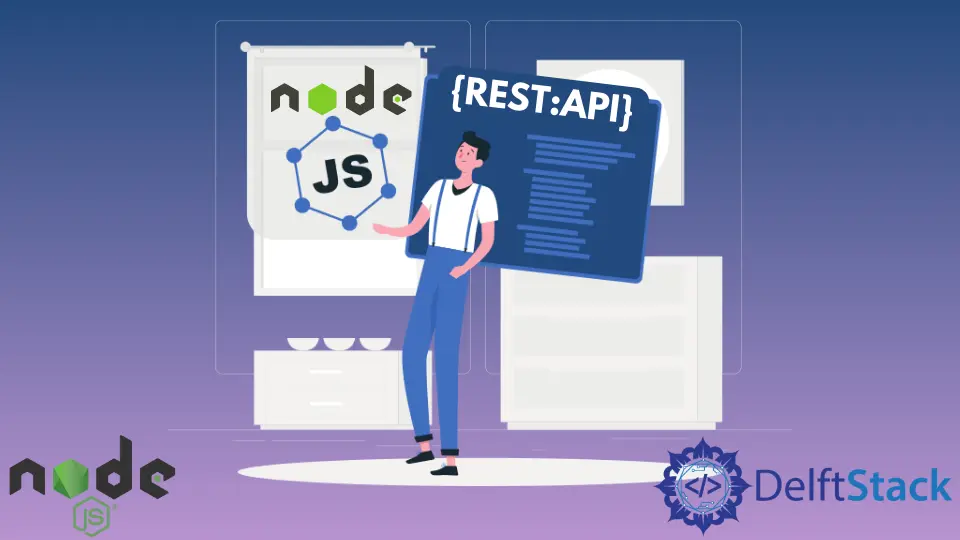
REST
stands for Representational State Transfer
. A REST
API or RESTful
API is an API or web API (Application Programming Interface) that conforms to the constraints/restrictions of the REST
architectural style and permits interaction with RESTful
web services.
In today’s post, we’ll learn how to make requests using third-party packages using Node.js.
Call REST
API in Node.js
The HTTP GET
method fetches resources from the server. For example, browsers use the HTTP GET
request method when fetching the TODO
data list from the server or fetching information of specific TODO
requests.
GET
and HEAD
requests do not change the server’s state.
GET
APIs are idempotent, which means making multiple identical requests always produce the same result every time until another API such as POST
or PUT
is made to the server, which changes the resource’s state on the server.
Axios
is a free third-party package, a Promise-based HTTP client for the browser and Node.js. It is available on NPM
.
You can send asynchronous HTTP requests to REST
endpoints using Axios
. Performing CRUD operations becomes an easy task using Axios
.
We can use it in Vanilla JavaScript or with a library like Node.js or Angular.
Install the Axios
library using this command $ npm i axios
.
A GET
request is created with the get
method.
const axios = require('axios');
async function getData() {
const res = await axios.get('https://jsonplaceholder.typicode.com/todos/1');
const data = res.data;
console.log(data);
}
getData();
In the example above, once the user runs the file, a GET
call is sent to the Node server with the specified URL (dummy in this post). If the server processes this data without interruption, it returns a successful message.
Based on the output of the server response, you can print the message on the console or notify the user with the appropriate message.
Output:
{ userId: 1, id: 1, title: 'delectus aut autem', completed: false }
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn