Node.js Heap Out of Memory
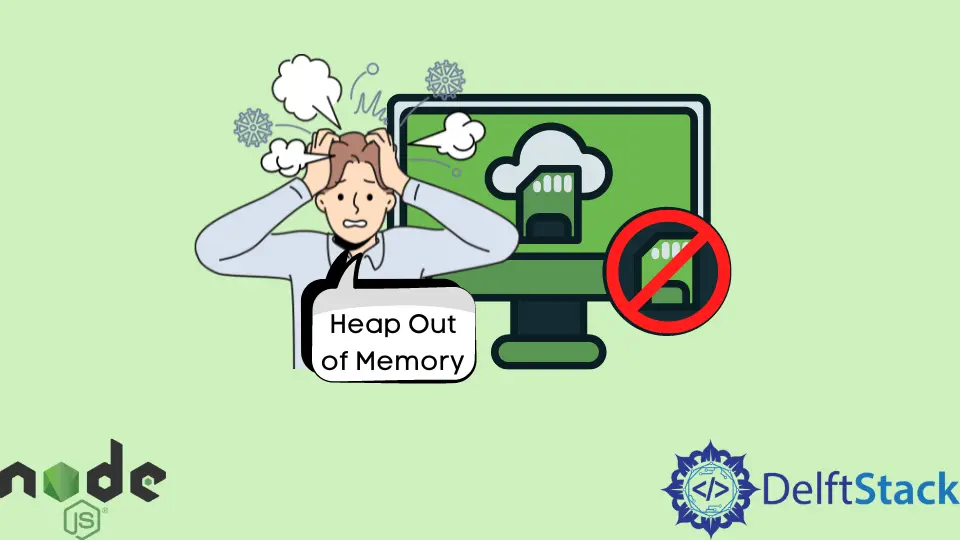
This article will help you solve the memory problem in JavaScript while using Node.js.
This error will happen when we load too much data at once. If you try to load a data set larger than available memory, you will run out of memory and get Fatal ERROR
.
Another big problem is the dreaded occurrence of memory leaks. Your application can normally fit in memory, but your memory usage will increase over time when a bug causes an allocation to hold.
A long-running application can take weeks or months, but eventually, the memory runs out, and your application stops running.
Memory restrictions are set by default in Node.js to prevent the program from consuming too much memory and crashing the entire system.
Depending on your system’s version and architecture, the results will vary (32bit or 64bit).
The quickest approach to solving this problem is increasing Node’s RAM limit. With Node.js v8, you can use the - max-old-space-size flag to set the limit in megabytes, as seen below:
node --max-old-space-size=4096 your_fileName.js
4 GB of memory is represented by the number 4096. You can set the limit to anything you like, but don’t use all of the available memory; otherwise, your system may crash.
Alternatively, you can set the flag in an environment variable, such as this:
NODE_OPTIONS="--max-old-space-size=4096" your_fileName.js
Changing the Node.js Memory Limits of the Environment
You must set the following variable in your environment’s configuration file if you wish to change Node.js’ memory limitations for your entire environment (.bashrc,.bash profile,.zshrc, and so forth).
Include the following line in your configuration file:
~/.bashrc
export NODE_OPTIONS=--max_old_space_size=4096
Use the npm install
to Heap Out of Memory
If you’re having trouble installing a package with npm or yarn, you can temporarily get around the memory limit by installing the package as follows:
node --max-old-space-size=4096 $(which npm) install -g nextawesomelib
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn