Truncated Incorrect Double Value in MySQL
- Understanding the Truncated Incorrect Double Value Error
- Method 1: Check Your Data Types
- Method 2: Clean Your Input Data
- Method 3: Use Proper SQL Syntax
- Conclusion
- FAQ
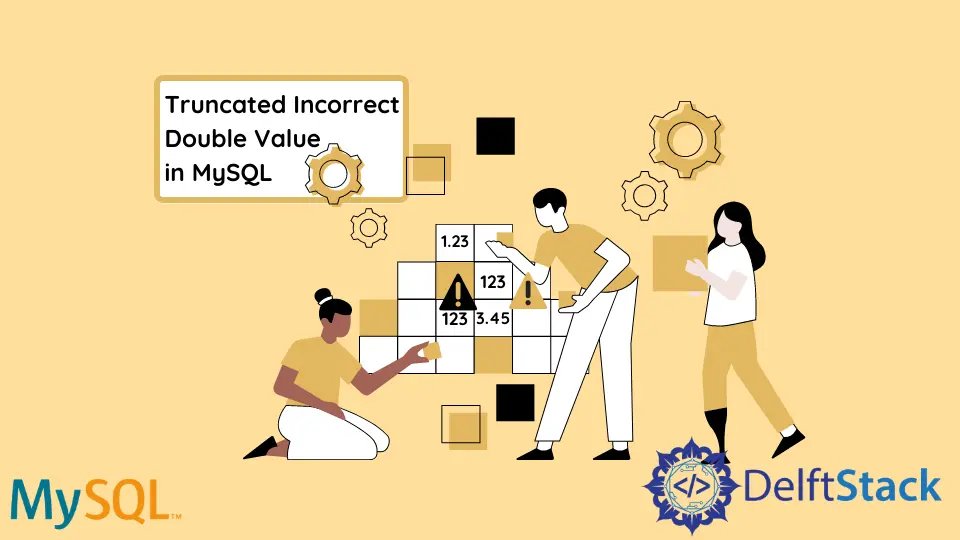
When working with MySQL databases, encountering errors is not uncommon. One of the more perplexing issues is the “truncated incorrect double value” error. This error typically arises when MySQL tries to convert a string or a number into a double type but fails due to incompatible formats or values. If you’ve ever faced this situation, you know how frustrating it can be, especially when you’re in the middle of a project.
In this tutorial, we will explore effective methods to fix the truncated incorrect double value error in MySQL, ensuring your database operations run smoothly. Let’s dive in and tackle this issue head-on!
Understanding the Truncated Incorrect Double Value Error
Before we jump into solutions, it’s essential to understand what causes this error. The “truncated incorrect double value” error occurs when MySQL encounters a string that it cannot convert into a double. This can happen for several reasons:
- The string contains non-numeric characters.
- The string is formatted incorrectly.
- You are trying to insert NULL or an empty value into a double column.
Identifying the root cause is crucial for applying the right fix.
Method 1: Check Your Data Types
One of the first steps in resolving the truncated incorrect double value error is to ensure that the data types in your MySQL table are correctly defined. If you are inserting data that does not match the expected data type, you will likely encounter this error.
To check your table structure, you can use the following SQL command:
DESCRIBE your_table_name;
This command will show you the data types of each column in your table. If you find that a column expected to hold double values is set to a different type, you can alter it using:
ALTER TABLE your_table_name MODIFY COLUMN column_name DOUBLE;
Ensure that the column is set to the correct type. After making these adjustments, try inserting your data again.
By confirming that your data types align with the values you are trying to insert, you can often resolve the truncated incorrect double value error. This step is crucial for maintaining data integrity and ensuring that your queries execute without issues.
Method 2: Clean Your Input Data
Another common reason for the truncated incorrect double value error is the presence of unexpected characters in your input data. If you are inserting data from user inputs or external sources, it’s essential to clean and validate this data before attempting to insert it into your database.
You can use the following Python code to clean your input data:
import re
def clean_input(value):
cleaned_value = re.sub(r'[^0-9.-]', '', value)
return float(cleaned_value) if cleaned_value else None
# Example of cleaning an input
input_value = "123.45abc"
cleaned_value = clean_input(input_value)
print(cleaned_value)
Output:
123.45
This code uses a regular expression to remove any non-numeric characters from the input string. If the cleaned string is empty, it returns None. Otherwise, it converts the cleaned string into a float. By implementing this cleaning process, you can ensure that only valid double values are inserted into your MySQL database, thus preventing the error from occurring.
Method 3: Use Proper SQL Syntax
Sometimes, the way you write your SQL queries can lead to the truncated incorrect double value error. If you’re using string concatenation or incorrectly formatted values in your SQL statements, it can result in this error.
Here’s how to properly format your SQL query in Python using parameterized queries:
import mysql.connector
def insert_value(value):
connection = mysql.connector.connect(
host='localhost',
user='your_username',
password='your_password',
database='your_database'
)
cursor = connection.cursor()
sql = "INSERT INTO your_table_name (column_name) VALUES (%s)"
cursor.execute(sql, (value,))
connection.commit()
cursor.close()
connection.close()
# Example of inserting a cleaned value
insert_value(cleaned_value)
Output:
Value inserted successfully
In this code snippet, we establish a connection to the MySQL database and prepare an SQL statement with a placeholder (%s) for the value. By using parameterized queries, we ensure that the input is properly escaped and formatted, which helps prevent the truncated incorrect double value error. This method is not only safer but also more efficient for inserting data into your database.
Conclusion
Fixing the truncated incorrect double value error in MySQL is crucial for maintaining the integrity of your database. By checking your data types, cleaning your input data, and using proper SQL syntax, you can effectively resolve this issue. Remember, the key to a smooth database experience is ensuring that your data is clean and correctly formatted. With these strategies in your toolkit, you can tackle any challenges that come your way in MySQL.
FAQ
-
what causes the truncated incorrect double value error in MySQL?
The error is caused when MySQL tries to convert a string or number into a double but encounters incompatible formats or values. -
how can I check the data types of my MySQL table?
You can use the DESCRIBE command followed by your table name to view the data types of each column. -
what is the best way to clean input data before inserting it into MySQL?
Use regular expressions to remove non-numeric characters and validate the data before insertion. -
why should I use parameterized queries in MySQL?
Parameterized queries help prevent SQL injection attacks and ensure that your input is properly formatted. -
can I fix the truncated incorrect double value error without changing my SQL queries?
Yes, by ensuring your data types are correct and cleaning your input data, you can often resolve the issue without modifying your queries.