MySQLi count() Function
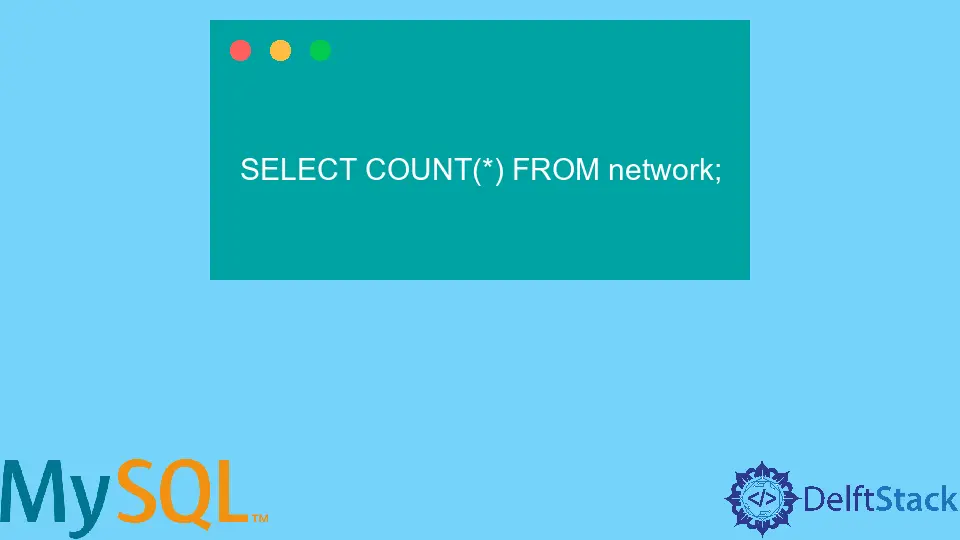
This is a special function in PHP MySQLi that counts and returns the number of rows present in a result set.
MySQLi Count()
Rows
Suppose we have the following data set in a table named network
.
We want to count the number of rows in the table. Use the following query.
SELECT COUNT(*) FROM network;
The above query returned the number of rows in the network
table.
Let’s say we want to count the rows that have any value in the managerid
column. For that reason, the following query will be used.
SELECT COUNT(managerid) FROM network;
As expected, we got the number of rows with some value in the managerid
column. Another point to ponder here is that we got 4
rows instead of 5
because it does not count rows with a NULL
value.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn