Transaction Log in MySQL
Preet Sanghavi
Jul 12, 2022
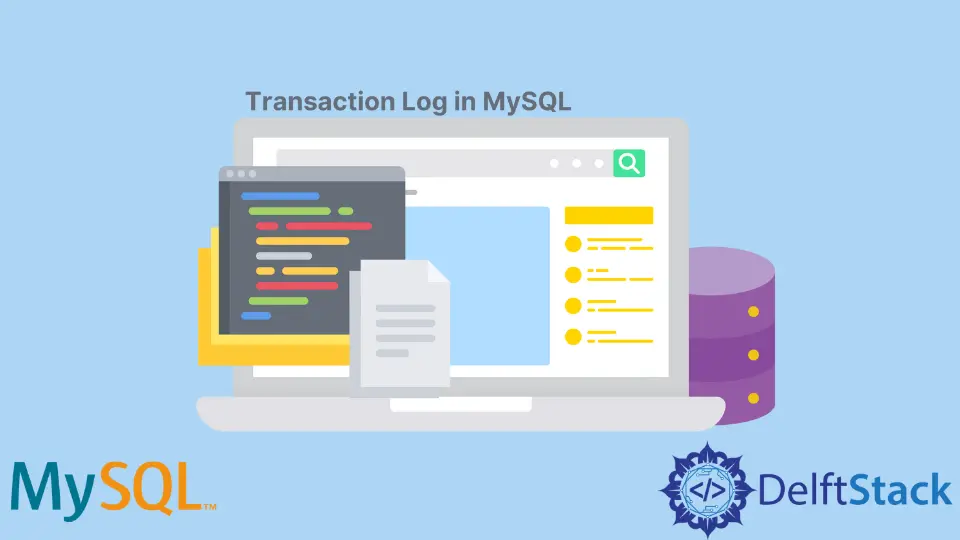
In this tutorial, we aim to explore how to access the transaction log in a MySQL database.
Access the Transaction Log in MySQL
A transaction log is nothing but a file containing all the information about recent and old transactions carried out on a particular database of a specific server in MySQL.
One must follow the following simple steps to track the transaction log file.
-
Ensure that you have MySQL installed on your local device. This can be understood with the help of the following query:
mysql --version` or `SHOW VARIABLES like '%version%';
-
Once you have checked the version’s relevance, go to the
MyComputer
icon on your device’s home screen. -
From there, route to the drive where the MySQL server is installed. By default, the server is installed on the
C
drive. -
Go to
Program Files
within theC
drive and route to the following location:C:\Program Files\MySQL\MySQL Server 5.0\data
-
This location would have a file named
%COMPUTERNAME%.log
, which would contain all the transaction information of your MySQL server.
Author: Preet Sanghavi