How to Split String in MySQL
- Using SUBSTRING_INDEX Function
- Using String Functions for Advanced Splitting
- Using JSON Functions for Splitting Strings
- Conclusion
- FAQ
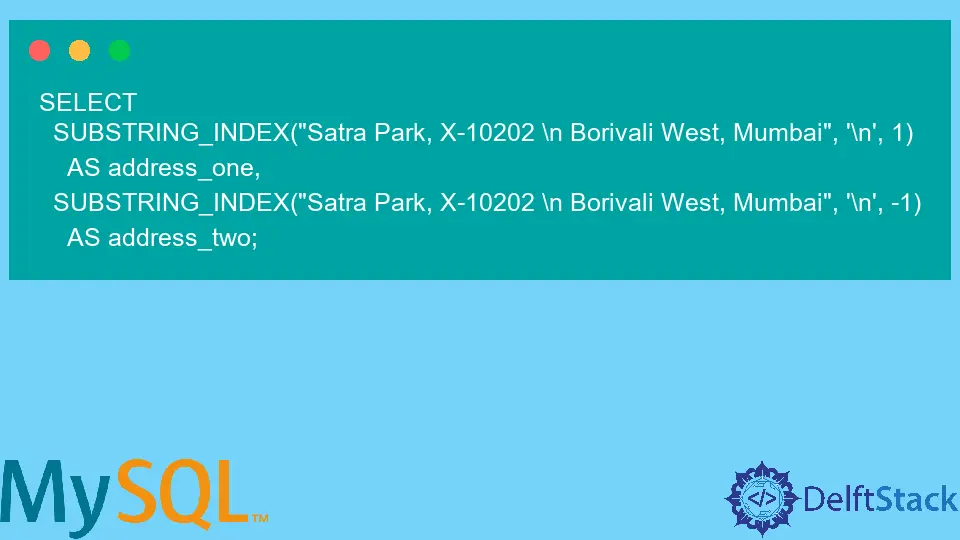
In the world of databases, string manipulation is often an essential skill, especially when working with MySQL. Whether you’re trying to extract specific pieces of information from a larger string or you need to format data for reporting, knowing how to split strings can save you a lot of time and effort.
In this tutorial, we’ll explore various methods to split a string in MySQL, including the use of built-in functions and advanced techniques. By the end of this article, you’ll have a solid understanding of how to manipulate strings effectively within your MySQL database, enabling you to handle data more efficiently.
Using SUBSTRING_INDEX Function
One of the most straightforward ways to split a string in MySQL is by using the SUBSTRING_INDEX
function. This function allows you to extract a substring from a string before or after a specified delimiter. It’s particularly useful when dealing with data formatted in a specific way, such as CSV strings.
Here’s how you can use SUBSTRING_INDEX
to split a string:
SELECT
SUBSTRING_INDEX('apple,banana,cherry', ',', 1) AS FirstFruit,
SUBSTRING_INDEX('apple,banana,cherry', ',', 2) AS FirstTwoFruits,
SUBSTRING_INDEX('apple,banana,cherry', ',', -1) AS LastFruit;
Output:
FirstFruit: apple
FirstTwoFruits: apple,banana
LastFruit: cherry
The SUBSTRING_INDEX
function takes three parameters: the string you want to split, the delimiter, and the index. A positive index returns the substring before the nth occurrence of the delimiter, while a negative index returns the substring after the last occurrence. This makes it incredibly versatile for various string manipulation tasks.
Using String Functions for Advanced Splitting
If you need more advanced string manipulation, you can combine several string functions in MySQL. For example, you might want to split a string into multiple parts and return them as separate rows. This can be achieved using a combination of SUBSTRING_INDEX
and a temporary table or a common table expression (CTE).
Here’s an example that demonstrates this:
CREATE TEMPORARY TABLE fruits AS
SELECT SUBSTRING_INDEX(SUBSTRING_INDEX('apple,banana,cherry', ',', numbers.n), ',', -1) AS fruit
FROM
(SELECT 1 AS n UNION ALL SELECT 2 UNION ALL SELECT 3 UNION ALL SELECT 4 UNION ALL SELECT 5) numbers
WHERE
numbers.n <= LENGTH('apple,banana,cherry') - LENGTH(REPLACE('apple,banana,cherry', ',', '')) + 1;
SELECT * FROM fruits;
Output:
fruit
apple
banana
cherry
In this example, we first create a temporary table that splits the string into separate rows. The inner query generates a series of numbers to indicate how many times we want to split the string. The outer query then uses these numbers to extract each fruit into its own row. This method is particularly useful when dealing with dynamic string lengths or when you want to analyze each part separately.
Using JSON Functions for Splitting Strings
With MySQL 5.7 and later, you can take advantage of JSON functions to split strings. This is especially useful if you are dealing with JSON data or if you want to leverage the flexibility of JSON arrays.
Here’s how to do it:
SET @json_string = '["apple","banana","cherry"]';
SELECT
JSON_UNQUOTE(JSON_EXTRACT(@json_string, '$[0]')) AS FirstFruit,
JSON_UNQUOTE(JSON_EXTRACT(@json_string, '$[1]')) AS SecondFruit,
JSON_UNQUOTE(JSON_EXTRACT(@json_string, '$[2]')) AS ThirdFruit;
Output:
FirstFruit: apple
SecondFruit: banana
ThirdFruit: cherry
In this example, we first define a JSON string representing an array of fruits. The JSON_EXTRACT
function is then used to retrieve individual elements from this array. The JSON_UNQUOTE
function is applied to remove the surrounding quotes from the output. This method is particularly powerful when working with JSON data, allowing for easy extraction of elements without complex string manipulation.
Conclusion
In summary, splitting strings in MySQL can be achieved through various methods, including the use of SUBSTRING_INDEX
, advanced string functions, and JSON functions. Each method has its unique advantages, depending on your specific use case. By mastering these techniques, you can enhance your data manipulation skills, making it easier to extract and analyze information from your MySQL database. Remember, the right approach often depends on the structure of your data and the desired outcome. Happy querying!
FAQ
-
What is the purpose of the SUBSTRING_INDEX function in MySQL?
The SUBSTRING_INDEX function is used to extract a substring from a string before or after a specified delimiter. -
Can I split a string into multiple rows in MySQL?
Yes, you can use a combination of string functions and a temporary table or CTE to split a string into multiple rows. -
How do JSON functions help in splitting strings in MySQL?
JSON functions allow you to work with JSON data and easily extract elements from JSON arrays, which can simplify string manipulation. -
Is there a limit to the number of splits I can perform in MySQL?
The limit depends on the length of the string and the structure of your query, but generally, MySQL can handle a significant number of splits. -
Are there performance considerations when splitting strings in MySQL?
Yes, complex queries involving string manipulation can impact performance, so it’s essential to optimize your queries and consider indexing where necessary.