How to Select All Tables in MySQL
- Query Information Schema to Select All Tables in a MySQL Database
-
Use the
SHOW TABLES
Statement to Select All Tables in a MySQL Database -
Use the
mysqlshow
Client to Select All Tables in a MySQL Database - Conclusion
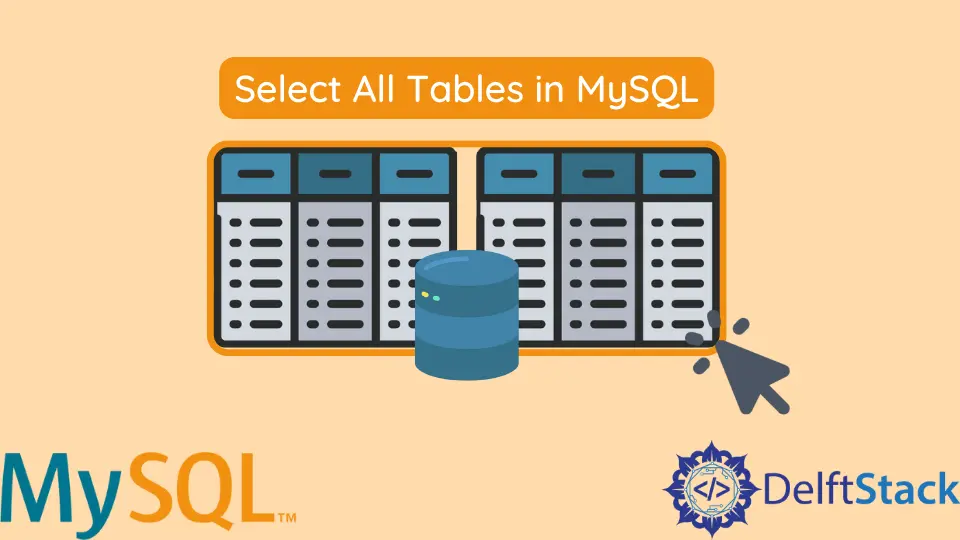
Effectively managing and analyzing database structures is a fundamental aspect of working with MySQL, a popular relational database management system. For businesses, organizations, and data professionals, the ability to select and understand all tables within a MySQL database is crucial for tasks ranging from onboarding new team members to conducting comprehensive data analyses.
In this guide, we will explore various methods and techniques to seamlessly retrieve the names of all tables within a MySQL database. Whether you’re a seasoned database administrator or a novice data analyst, this comprehensive tutorial will provide you with the insights and practical steps needed to navigate and retrieve valuable information from your MySQL databases.
Query Information Schema to Select All Tables in a MySQL Database
One powerful method to retrieve a list of all tables within a MySQL database involves querying the Information Schema.
The Information Schema in MySQL is a virtual database that contains metadata about the server, databases, tables, columns, and more. By querying the Information Schema, you can retrieve valuable information about the structure and properties of your databases and their components.
The general syntax for querying Information Schema to select all tables is as follows:
SELECT table_name
FROM information_schema.tables
WHERE table_schema = 'your_database_name';
Replace your_database_name
with the actual name of your MySQL database. This query filters the tables based on the specified database, extracting relevant information from the information_schema.tables
view.
Code Example 1: Basic Query
-- Selecting all tables from a specific database
SELECT table_name
FROM information_schema.tables
WHERE table_schema = 'library_db';
In this basic query, we aim to retrieve a list of all tables within a specific MySQL database.
The SELECT table_name FROM information_schema.tables WHERE table_schema = 'library_db';
statement is crafted to fetch the names of tables stored in the library_db
database. The information_schema.tables
view provides metadata about various tables across databases.
The table_schema
condition ensures that only tables from the specified database are included in the result set.
Output:
+---------------------------------------+
| TABLE_NAME |
+---------------------------------------+
| authors |
| publishers |
| books |
+---------------------------------------+
This straightforward query is foundational for understanding the core syntax needed to select all tables within a database.
Code Example 2: Using a Variable
-- Using a variable for the database name
SET @database_name = 'library_db';
SELECT table_name
FROM information_schema.tables
WHERE table_schema = @database_name;
In this example, we introduce the concept of using a variable to store the database name, enhancing the query’s flexibility.
We begin by setting a variable with SET @database_name = 'library_db';
, assigning the desired database name to @database_name
. Subsequently, the SELECT table_name FROM information_schema.tables WHERE table_schema = @database_name;
statement utilizes the variable in the WHERE
clause, allowing for dynamic database selection.
Output:
+---------------------------------------+
| TABLE_NAME |
+---------------------------------------+
| authors |
| publishers |
| books |
+---------------------------------------+
This technique proves useful when the database name needs to be reused in multiple queries, offering a more adaptable and efficient approach.
Code Example 3: Retrieving Table Names with Additional Information
-- Retrieving table names with additional information
SELECT table_name, engine, table_rows
FROM information_schema.tables
WHERE table_schema = 'library_db';
This example enriches the result set by including additional information about each table, expanding beyond the basic query.
The statement SELECT table_name, engine, table_rows FROM information_schema.tables WHERE table_schema = 'library_db';
fetches not only the table names but also details such as the storage engine (engine
) and the number of rows in each table (table_rows
).
Output:
+---------------------------------------+--------+------------+
| TABLE_NAME | ENGINE | TABLE_ROWS |
+---------------------------------------+--------+------------+
| authors | InnoDB | 3 |
| books | InnoDB | 3 |
| publishers | InnoDB | 2 |
+---------------------------------------+--------+------------+
Customizing the selected columns enables a more comprehensive overview of the tables, providing insights into their structure and content.
Code Example 4: Sorting Tables Alphabetically
-- Sorting tables alphabetically
SELECT table_name
FROM information_schema.tables
WHERE table_schema = 'your_database_name'
ORDER BY table_name;
Sorting the retrieved table names alphabetically is the focus of this example.
The query SELECT table_name FROM information_schema.tables WHERE table_schema = 'your_database_name' ORDER BY table_name;
adds an ORDER BY
clause to the basic query. This clause arranges the results alphabetically based on the table names.
Output:
+---------------------------------------+
| TABLE_NAME |
+---------------------------------------+
| authors |
| books |
| publishers |
+---------------------------------------+
The ability to sort tables facilitates better organization and readability, especially when dealing with a large number of tables within a database.
Code Example 5: Excluding System Tables
-- Excluding system tables
SELECT table_name
FROM information_schema.tables
WHERE table_schema = 'your_database_name' AND table_type = 'BASE TABLE';
In certain scenarios, it may be necessary to exclude system tables from the result set.
The query SELECT table_name FROM information_schema.tables WHERE table_schema = 'your_database_name' AND table_type = 'BASE TABLE';
achieves this by incorporating an additional condition. The table_type = 'BASE TABLE'
condition ensures that only base tables, excluding system tables, are included in the output.
Output:
+------------+
| TABLE_NAME |
+------------+
| authors |
| publishers |
| books |
+------------+
This example showcases how to tailor the query to filter out specific types of tables, focusing on those relevant to user data rather than system operations.
Use the SHOW TABLES
Statement to Select All Tables in a MySQL Database
When it comes to retrieving a list of all tables within a MySQL database, another approach is to use the SHOW TABLES
statement. This statement is concise and offers a quick way to fetch table names without delving into the intricacies of the Information Schema.
The syntax for the SHOW TABLES
statement is straightforward:
SHOW TABLES;
Let’s consider a scenario where we have a database named exampledb
with three sample tables: table1
, table2
, and table3
. Below are multiple code examples demonstrating the use of the SHOW TABLES
statement:
Code Example 1: Basic Usage
-- Show all tables in the current database
SHOW TABLES;
Here, we present the basic usage of the SHOW TABLES
statement in MySQL. This straightforward command is designed to provide a quick overview of all tables within the current database.
When executed, it returns a simple list of table names, making it a convenient option for users who seek rapid insight into the existing tables without delving into more detailed metadata.
Output:
+----------------------------------+
| Tables_in_exampledb |
+----------------------------------+
| table1 |
| table2 |
| table3 |
+----------------------------------+
Code Example 2: Using LIKE
for Pattern Matching
-- Show tables that match a pattern (e.g., tables starting with 'table')
SHOW TABLES LIKE 'table%';
This example introduces a more advanced use of the SHOW TABLES
statement by incorporating the LIKE
keyword for pattern matching. In this case, we aim to retrieve tables that match a specific pattern.
The query SHOW TABLES LIKE 'table%';
is tailored to showcase tables whose names start with the prefix table
. The %
symbol serves as a wildcard, representing zero or more characters.
Output:
+-------------------------------------------+
| Tables_in_exampledb (table%) |
+-------------------------------------------+
| table1 |
| table2 |
| table3 |
+-------------------------------------------+
This feature is particularly useful for users who want to filter tables based on specific naming conventions or patterns within the database.
Code Example 3: Using SHOW FULL TABLES
-- Show full information about all tables in the current database
SHOW FULL TABLES;
In this example, the SHOW FULL TABLES
statement is employed to display comprehensive details about all tables within the current database.
When using SHOW FULL TABLES
, the result set will include columns like Tables_in_database
(the table name) and Table_type
(the type of the table, e.g., BASE TABLE
). This additional information can be beneficial for users who require a deeper understanding of the database schema and configuration.
Executing this query provides a more detailed overview compared to the basic SHOW TABLES
statement, offering insights into the structure and characteristics of each table.
Output:
+----------------------------------+------------+
| Tables_in_exampledb | Table_type |
+----------------------------------+------------+
| table1 | BASE TABLE |
| table2 | BASE TABLE |
| table3 | BASE TABLE |
+----------------------------------+------------+
Use the mysqlshow
Client to Select All Tables in a MySQL Database
In addition to SQL statements like SHOW TABLES
, MySQL provides a command-line utility called mysqlshow
that allows users to obtain information about databases and tables concisely.
Before we proceed, ensure that the mysqlshow
client is installed on your system. It is typically included with the MySQL client package.
If you don’t have it installed, you can do so by installing the MySQL client using your package manager. For example, on a Linux system, you can use the following command:
sudo apt-get install mysql-client
Once installed, you can use the mysqlshow
command to interact with your MySQL server.
The basic syntax for using mysqlshow
to list tables is as follows:
mysqlshow -u username -p[password] database_name
Replace username
, [password]
, and database_name
with your MySQL username, password (if any), and the name of the database you want to inspect.
Code Example: Using mysqlshow
to List Tables
Let’s assume we have a database named exampledb
with three tables: table1
, table2
, and table3
. Here’s how you can use mysqlshow
to list these tables:
mysqlshow -u your_username -p your_password exampledb
Here, the -u
flag specifies the MySQL username, the -p
flag prompts for the password, and the last argument is the name of the database, exampledb
.
Upon executing the command, the mysqlshow
client fetches and displays a list of tables within the specified database along with additional information such as the table type and the number of rows. This quick and concise output can be beneficial for users who prefer a command-line interface for exploring database structures.
Output:
+------------------+
| Tables |
+------------------+
| table1 |
| table2 |
| table3 |
+------------------+
It’s worth noting that the mysqlshow
client doesn’t require familiarity with SQL syntax, making it an accessible option for users who might be more comfortable with command-line interactions. However, for more detailed metadata and flexibility, SQL queries or the SHOW TABLES
statement might be more suitable.
Conclusion
In conclusion, exploring and selecting all tables in a MySQL database can be approached through various methods, each catering to different preferences and needs. Whether using SQL queries such as SHOW TABLES
and querying the Information Schema, or employing command-line utilities like mysqlshow
, each method has its strengths.
SQL queries offer flexibility and detailed metadata, while command-line tools provide a quick and accessible means for users who are more comfortable with terminal interactions. Understanding these diverse approaches equips database administrators, developers, and analysts with the tools to efficiently navigate and manage MySQL databases.
The choice of method ultimately depends on the user’s familiarity with SQL syntax, the level of detail required, and personal workflow preferences.