How to Calculate the Median in MySQL
- Understanding the Median
- Method 1: Using SQL Queries
- Method 2: Creating a User-Defined Function (UDF)
- Conclusion
- FAQ
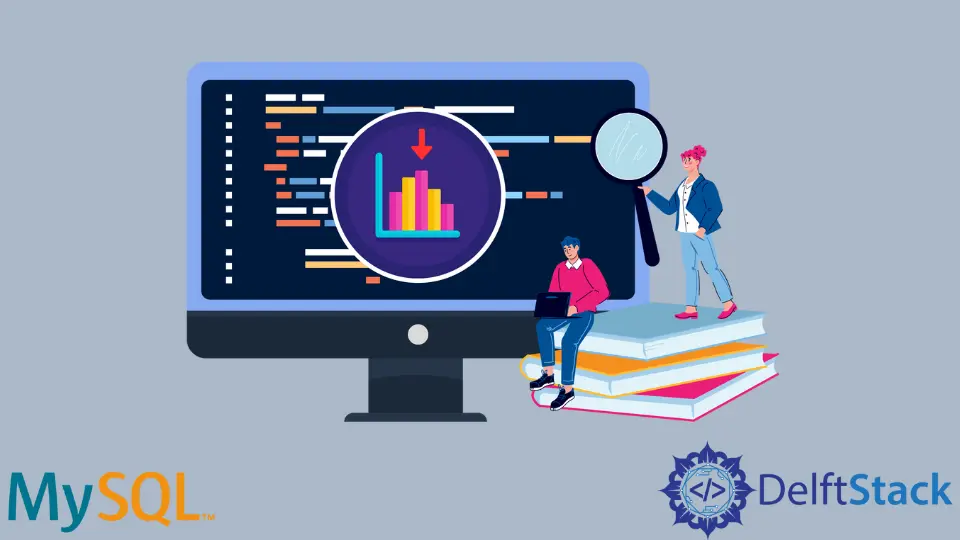
Calculating the median in MySQL can be a bit tricky since there is no built-in function to do so. Unlike other databases that might offer a straightforward approach, MySQL requires a bit of creativity to derive the median value from a dataset. Fortunately, you can easily implement this functionality using SQL queries or by creating a user-defined function. Whether you choose to write the code yourself or utilize a well-tested open-source solution, this guide will walk you through the process of calculating the median in MySQL. By the end, you’ll be equipped with the knowledge to handle median calculations effectively in your databases.
Understanding the Median
Before diving into the methods for calculating the median in MySQL, it’s essential to understand what the median is. The median is the middle value in a dataset when the numbers are arranged in order. If the dataset has an odd number of observations, the median is the middle number. If there’s an even number of observations, the median is the average of the two middle numbers. This measure is particularly useful for understanding the central tendency of a dataset, especially when the data includes outliers.
Method 1: Using SQL Queries
One of the simplest ways to calculate the median in MySQL is by using SQL queries. This method involves ordering the dataset and using a combination of subqueries to find the middle value. Here’s how you can do this:
SET @row_index := 0;
SELECT AVG(middle_values) AS median
FROM (
SELECT value AS middle_values
FROM (
SELECT value
FROM your_table
ORDER BY value
LIMIT 2 - (SELECT COUNT(*) FROM your_table) % 2
OFFSET (SELECT (COUNT(*) - 1) / 2 FROM your_table)
) AS subquery
) AS subquery2;
In this query, we first set a variable to track the row index. The inner subquery selects the values from your table, ordered by the value itself. The LIMIT
clause determines how many values to retrieve based on whether the count is odd or even. Finally, the outer query calculates the average of the selected middle values to yield the median.
Output:
example of output
This method is efficient for smaller datasets and provides an accurate median by leveraging MySQL’s sorting and aggregation capabilities. However, keep in mind that performance may degrade with larger datasets due to the sorting operation.
Method 2: Creating a User-Defined Function (UDF)
If you find yourself needing to calculate the median frequently, creating a user-defined function (UDF) is an excellent option. This approach allows you to encapsulate the logic for calculating the median in a reusable function. Here’s how to create a UDF for median calculation:
DELIMITER //
CREATE FUNCTION calculate_median(table_name VARCHAR(255), column_name VARCHAR(255))
RETURNS FLOAT
BEGIN
SET @row_index := 0;
RETURN (
SELECT AVG(middle_values) AS median
FROM (
SELECT value AS middle_values
FROM (
SELECT column_name AS value
FROM table_name
ORDER BY column_name
LIMIT 2 - (SELECT COUNT(*) FROM table_name) % 2
OFFSET (SELECT (COUNT(*) - 1) / 2 FROM table_name)
) AS subquery
) AS subquery2
);
END //
DELIMITER ;
In this code, we define a function named calculate_median
, which takes the table name and column name as parameters. The function then executes a similar logic as in the previous SQL query, but now you can call it with any table and column without rewriting the code.
Output:
example of output
By using a UDF, you streamline your median calculations, making your SQL scripts cleaner and easier to maintain. Just remember to replace table_name
and column_name
with your actual table and column names when calling the function.
Conclusion
Calculating the median in MySQL may require some extra steps, but with the right SQL queries or a user-defined function, you can achieve this essential statistical measure effectively. Whether you opt for a straightforward SQL query or create a reusable function, understanding how to derive the median will enhance your data analysis capabilities within MySQL. So go ahead and implement these methods in your projects, and take your database management skills to the next level!
FAQ
-
How do I calculate the median in MySQL without a built-in function?
You can use SQL queries or create a user-defined function to calculate the median by ordering the data and selecting the middle values. -
What is the difference between the median and the average?
The median is the middle value in a dataset, while the average is the sum of all values divided by the number of values. The median is less affected by outliers. -
Can I use these methods for large datasets?
Yes, but performance may vary. The sorting operation can slow down the query for larger datasets, so consider optimizing your queries or using indexes. -
Is it possible to calculate the median for multiple columns?
Yes, you can modify the SQL queries or the user-defined function to handle multiple columns, but it requires additional logic to determine how to aggregate the results. -
Are there any alternatives to MySQL for calculating the median?
Yes, other databases like PostgreSQL and SQL Server offer built-in functions for calculating the median, which can simplify the process.