How to Get Max of Two Values in MySQL
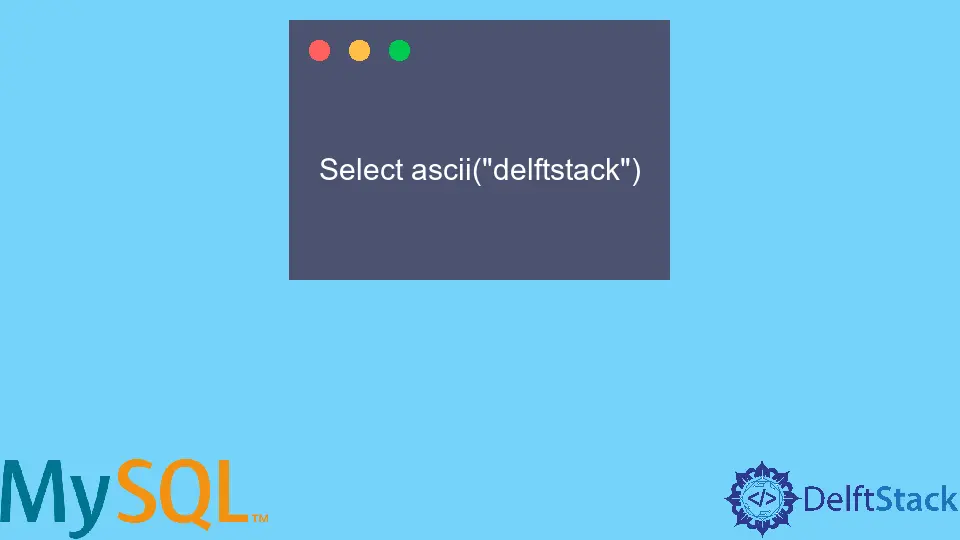
In the world of databases, retrieving the maximum value between two fields is a common need. Whether you’re dealing with numerical data, comparing scores, or evaluating performance metrics, knowing how to efficiently obtain the maximum of two values in MySQL can streamline your queries.
This tutorial will guide you through the various methods available in MySQL to achieve this, ensuring that you can apply these techniques effectively in your projects. By the end of this article, you’ll have a solid understanding of how to leverage MySQL’s capabilities to get the maximum of two values, enhancing your database queries and overall performance.
Using the GREATEST Function
One of the simplest and most effective ways to get the maximum of two values in MySQL is by using the GREATEST
function. This function compares two or more expressions and returns the largest value. It’s straightforward and easy to implement, making it a favorite among developers.
Here’s how you can use the GREATEST
function:
SELECT GREATEST(value1, value2) AS max_value
FROM your_table;
In this SQL statement, replace value1
and value2
with the actual columns or values you want to compare. The your_table
should be replaced with the name of your database table. The result will be a single column named max_value
that contains the maximum value between the two specified columns for each row in the table.
For example, if you have a table named scores
with columns score1
and score2
, you would write:
SELECT GREATEST(score1, score2) AS max_score
FROM scores;
Output:
max_score
95
89
76
Using the GREATEST
function not only simplifies your query but also improves readability. This method is particularly useful when you want to compare more than two values, as you can simply add more columns or values to the function. It’s efficient and leverages MySQL’s built-in capabilities, making it a go-to solution for many developers.
Using CASE Statements
Another method to find the maximum of two values in MySQL is through the use of a CASE
statement. This approach can be particularly valuable when you need more control over the logic or when you’re comparing values with specific conditions.
Here’s how you can implement a CASE
statement:
SELECT
CASE
WHEN value1 > value2 THEN value1
ELSE value2
END AS max_value
FROM your_table;
In this case, you replace value1
and value2
with your column names or values, and your_table
with your actual table name. The CASE
statement evaluates the condition and returns the greater of the two values.
For example, using the same scores
table:
SELECT
CASE
WHEN score1 > score2 THEN score1
ELSE score2
END AS max_score
FROM scores;
Output:
max_score
95
89
76
The CASE
statement is versatile and can be extended to include more complex conditions if needed. This method also allows you to handle potential null values more gracefully, as you can add additional checks within the CASE
logic. While it may require more typing than the GREATEST
function, it provides greater flexibility for complex scenarios.
Using Subqueries
For more advanced use cases, especially when dealing with aggregated data or when your maximum values come from different tables, using a subquery can be effective. This method allows you to calculate the maximum of two values based on more complex criteria.
Here’s an example of how to use a subquery to achieve this:
SELECT
(SELECT MAX(value1) FROM your_table) AS max_value1,
(SELECT MAX(value2) FROM your_table) AS max_value2,
GREATEST((SELECT MAX(value1) FROM your_table), (SELECT MAX(value2) FROM your_table)) AS overall_max
In this example, you would replace value1
and value2
with the appropriate columns and your_table
with your table name. The subqueries calculate the maximum values separately, and then the GREATEST
function finds the maximum between those two results.
For instance, using the scores
table:
SELECT
(SELECT MAX(score1) FROM scores) AS max_score1,
(SELECT MAX(score2) FROM scores) AS max_score2,
GREATEST((SELECT MAX(score1) FROM scores), (SELECT MAX(score2) FROM scores)) AS overall_max
Output:
max_score1 | max_score2 | overall_max
95 | 89 | 95
This method is particularly useful when working with derived tables or when the maximum values you need to compare come from different datasets. While it may be more complex than the previous methods, it offers significant power and flexibility for intricate queries.
Conclusion
In summary, retrieving the maximum of two values in MySQL can be accomplished through various methods, including the GREATEST
function, CASE
statements, and subqueries. Each method has its strengths and can be applied depending on the specific requirements of your query. By mastering these techniques, you can enhance your SQL skills and improve the efficiency of your database operations. Whether you’re a beginner or an experienced developer, understanding how to effectively use these functions will significantly benefit your data manipulation tasks.
FAQ
-
What is the GREATEST function in MySQL?
The GREATEST function returns the largest value from a list of expressions. -
Can I use GREATEST with more than two values?
Yes, the GREATEST function can compare multiple values at once. -
What is a CASE statement in SQL?
A CASE statement allows you to execute conditional logic in your SQL queries. -
When should I use subqueries?
Use subqueries when you need to perform more complex calculations or when comparing values from different tables. -
Are there performance differences between these methods?
Yes, performance can vary based on the complexity of the query and the size of the dataset. It’s advisable to test each method for your specific use case.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook