How to Kill Connections in MySQL
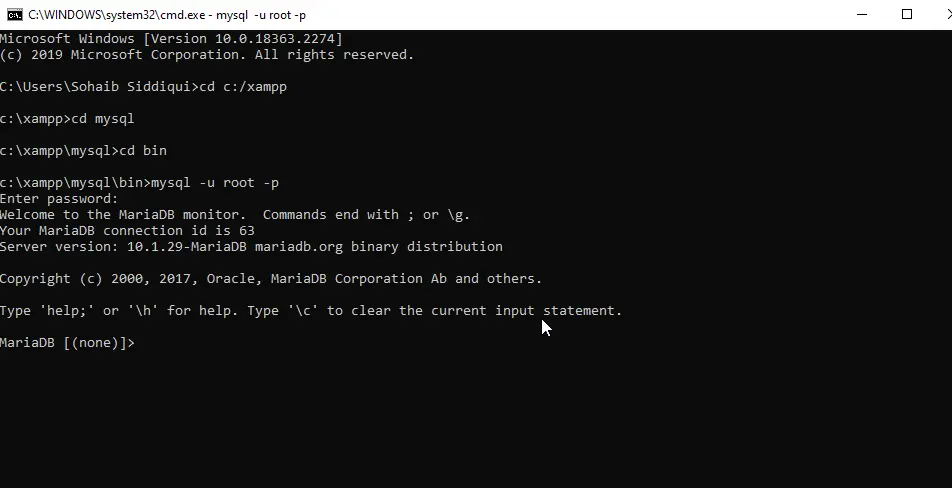
This small article will discuss the problem with MySQL inactive processes and how we can kill the unused processes in the MySQL server.
Kill Connections in MySQL
While using the MySQL database in your software, desktop, or web, there can be a problem that you lose the connection with the database, and it states an error of Can't connect to the database
. Or it can be Too many connections
.
Such errors are caused when there are too many users of that database, or you are doing something wrong in your development, for example, not closing the previously opened connections. Any software tool for MySQL, such as TOAD, does not provide any utility to delete the inactive connections to the database.
This is done only using the command prompt.
For that, you first need to open MySQL in the command prompt. Go to the location of your mysql/bin
folder and type in the following command to connect to MySQL:
mysql -u root -p
This is shown in the following image:
By this step, we have connected to our database. We can use the following command to show the list of processes:
SHOW PROCESSLIST
This will give the list of all the currently active processes in the database server with their process IDs and all other details.
Now, if you need to kill any process, you can use the command:
KILL [process_ID]
In place of [process_ID]
, you need to provide the ID of that process you need to kill. By this, you can kill all the unused processes and retain your database connection.
The above image shows that when we killed the connection with ID 3
, it shows us the message that the connection was killed. Because this killed connection was the only connection to the database, it recreated a connection.