How to Insert Date in MySQL
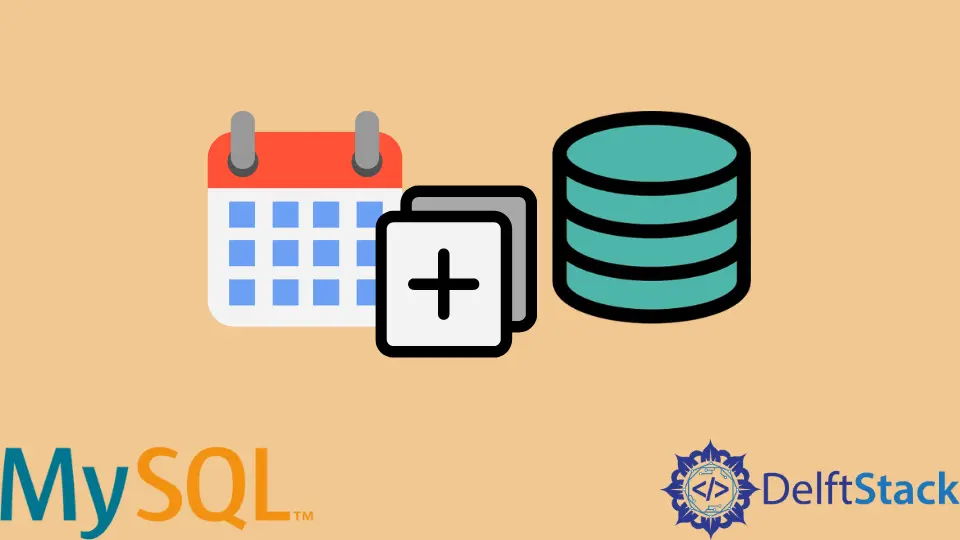
In this tutorial, we aim at exploring how to insert date values in a MySQL table.
Businesses and organizations using SQL services generally add dates to their records. These dates are extremely useful as they help write efficient queries with the date filter, keep track of different users based on their date of registration and exit from the system, etc.
These dates can be put up in any suitable format for the user. The most general format employed by programmers and senior data analysts in their SQL queries is yyyy-mm-dd
.
Let us try to understand how to insert a date column in MySQL. It can be done using the INSERT INTO
method.
Before we begin, we will create a table and insert a row with the date of registration for a student.
-- create the table student_dates
CREATE TABLE student_dates(
stu_id int,
stu_firstName varchar(255) DEFAULT NULL,
stu_date date,
primary key(stu_id)
);
-- insert rows to the table student_dates
INSERT INTO student_dates(stu_id,stu_firstName,stu_date)
VALUES(1,"Preet",STR_TO_DATE('24-May-2005', '%d-%M-%Y'));
To view the entries in the data, we use the following code.
SELECT * FROM student_dates;
Output:
stu_id stu_firstName stu_date
1 Preet 2005-05-24
As we can see in the code above block, the date 24-May-2005
has been converted to 2005-05-24
date format. This date is represented as day, month, and year in that order.
mm-dd-yyyy
, we can use the '%M-%d-%Y'
command in the STR_TO_DATE
function.Therefore, with the help of the INSERT INTO
statement and the STR_TO_DATE()
function, we can efficiently update a column of one table based on values from another table in MySQL.