How to Escape Single Quote in MySQL
-
Using the Character
'
Within a Word With''
to Escape Single Quote in MySQL -
Using the Character
"
Within a Word With""
to Escape Double Quote in MySQL -
Using the
\
Character to Escape Double Quote in MySQL
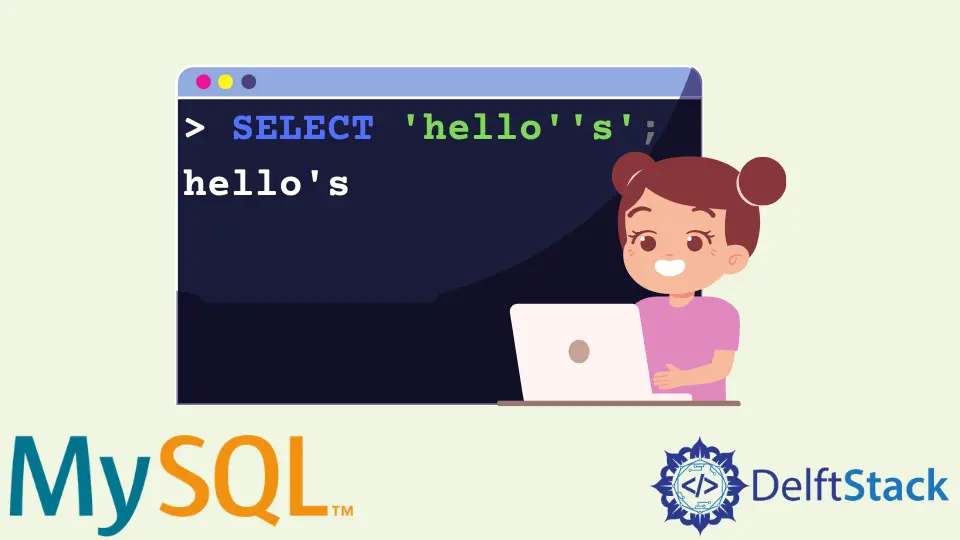
In this tutorial, we aim at exploring different methods to understand how to escape the single quote in MySQL.
Generally, businesses and enterprises that use MySQL for database management tend to include single case characters. Including these characters can be a cumbersome task as these quotes are often involved in creating the base syntax for the MySQL query. We must understand how to escape single quotes.
There are three main approaches that can help us with this task. They can be illustrated as follows.
- Character as
'
within a sentence or word with'
can be illustrated as''
. - Character
"
within a sentence or word with"
can be illustrated as""
. - We can also put the character
\
to indicate a quote in MySQL.
Let us understand how this method works in greater depth.
Using the Character '
Within a Word With ''
to Escape Single Quote in MySQL
Suppose we need to print the word Hello's
in MySQL using single inverted commas. This can be achieved with the following query.
SELECT 'hello''s';
The query above in MySQL would generate the following result.
hello's
Using the Character "
Within a Word With ""
to Escape Double Quote in MySQL
Suppose we need to print the word Hello"s
in MySQL with the word covered with double inverted commas. This can be achieved with the following query.
SELECT "hello""s";
The aforementioned query in MySQL would generate the following result.
hello"s
Using the \
Character to Escape Double Quote in MySQL
An alternative to the '
indication can be the use of the backslash symbol in MySQL. Let us assume we wish to print the word 'hello
. This can be understood with the following query.
SELECT '\'hello';
The aforementioned query in MySQL would generate the following result.
'hello
Therefore, with the help of the three aforementioned techniques, we can escape a single quote in MySQL.