How to Drop Multiple Tables in MySQL
-
Use the
INSERT
Statement to Insert Entries in a Table in MySQL -
Use the
DROP TABLE
Statement to Drop Multiple Tables in MySQL
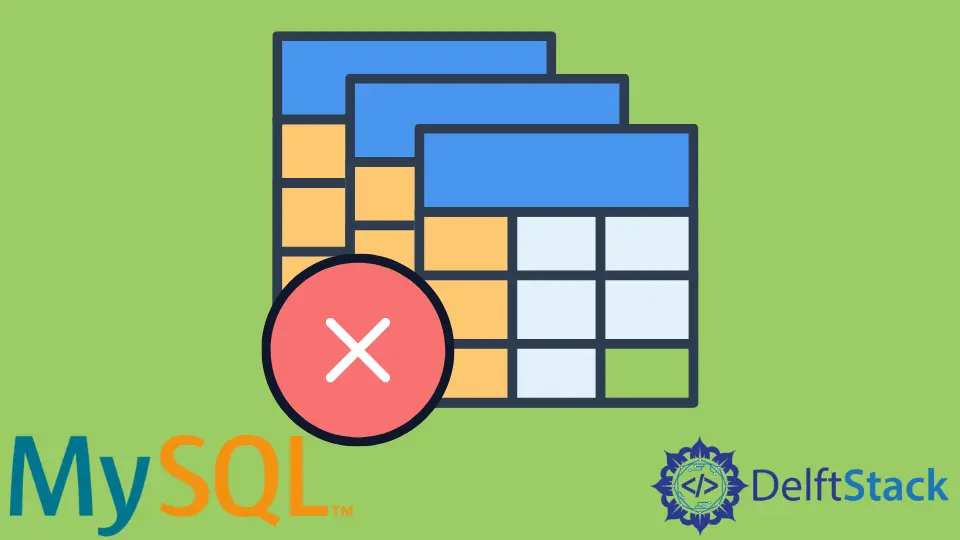
This tutorial aims to understand how to drop multiple tables in MySQL.
Most businesses and organizations that use MySQL to visualize and analyze data have multiple tables to work with. Sometimes, these tables become redundant. In such instances, one needs to get rid of these tables to make space for newer tables or records.
Moreover, it becomes essential to understand how to get rid of multiple tables simultaneously to avoid cluttered queries that run for hours individually. Simultaneously dropping multiple queries can also help increase the readability of the overall code library.
Let us try to understand this in greater depth.
However, we create two dummy tables to work with before we begin. Here we create a table, student_dates
, along with a few rows.
-- create the table student_dates
CREATE TABLE student_dates(
stu_id int,
stu_firstName varchar(255) DEFAULT NULL,
stu_date date,
primary key(stu_id)
);
Similarly, we can create the table student_details
and a few defined rows with the following query.
-- create the table student_details
CREATE TABLE student_details(
stu_id int,
stu_firstName varchar(255) DEFAULT NULL,
stu_lastName varchar(255) DEFAULT NULL,
primary key(stu_id)
);
Use the INSERT
Statement to Insert Entries in a Table in MySQL
The previous query creates a table with the name student_dates
. With the INSERT
statement’s help, let us add data for a few students. This operation can be done as follows.
-- insert rows to the table student_dates
INSERT INTO student_dates(stu_firstName,stu_date)
VALUES("Preet",STR_TO_DATE('24-May-2005', '%d-%M-%Y')),
("Dhruv",STR_TO_DATE('14-June-2001', '%d-%M-%Y')),
("Mathew",STR_TO_DATE('13-December-2020', '%d-%M-%Y')),
("Jeet",STR_TO_DATE('14-May-2003', '%d-%M-%Y')),
("Steyn",STR_TO_DATE('19-July-2002', '%d-%M-%Y'));
The code would enter the student data in the table student_dates
. We can visualize this table with the following command.
SELECT * from student_dates;
The code block would generate the following output.
stu_id stu_firstName stu_date
1 Preet 2005-05-24
2 Dhruv 2001-06-14
3 Mathew 2020-12-13
4 Jeet 2003-05-14
5 Steyn 2002-07-19
Similarly, let us insert values in the student_details
table. We can do this with the following query.
-- insert rows to the table student_details
INSERT INTO student_details(stu_id,stu_firstName,stu_lastName)
VALUES(1,"Preet","Sanghavi"),
(2,"Rich","John"),
(3,"Veron","Brow"),
(4,"Geo","Jos"),
(5,"Hash","Shah"),
(6,"Sachin","Parker"),
(7,"David","Miller");
The student_details
table can be visualized with the help of the following query.
SELECT * from student_details;
Output:
stu_id stu_firstName stu_lastName
1 Preet Sanghavi
2 Rich John
3 Veron Brow
4 Geo Jos
5 Hash Shah
6 Sachin Parker
7 David Miller
Use the DROP TABLE
Statement to Drop Multiple Tables in MySQL
To get rid of a table in MySQL, one can use the DROP TABLE
statement. The syntax of this statement can be illustrated as follows.
DROP TABLE name_of_the_table;
The table named name_of_the_table
will drop in the query.
Now let us aim at dropping multiple tables, namely student_dates
and student_details
. We can do this with the following query.
DROP TABLE IF EXISTS student_dates, student_details;
The query would eliminate the tables student_dates
and student_details
. These names can now be used to name tables with newer records.
Thus with the help of the DROP TABLE
statement, we can efficiently get rid of multiple tables quickly and efficiently with high code readability in MySQL.