How to Create Table From CSV in MySQL
- Understanding CSV Files and MySQL
- Method 1: Using MySQL’s LOAD DATA INFILE Command
- Method 2: Using MySQL Workbench
- Method 3: Using a Python Script with MySQL Connector
- Conclusion
- FAQ
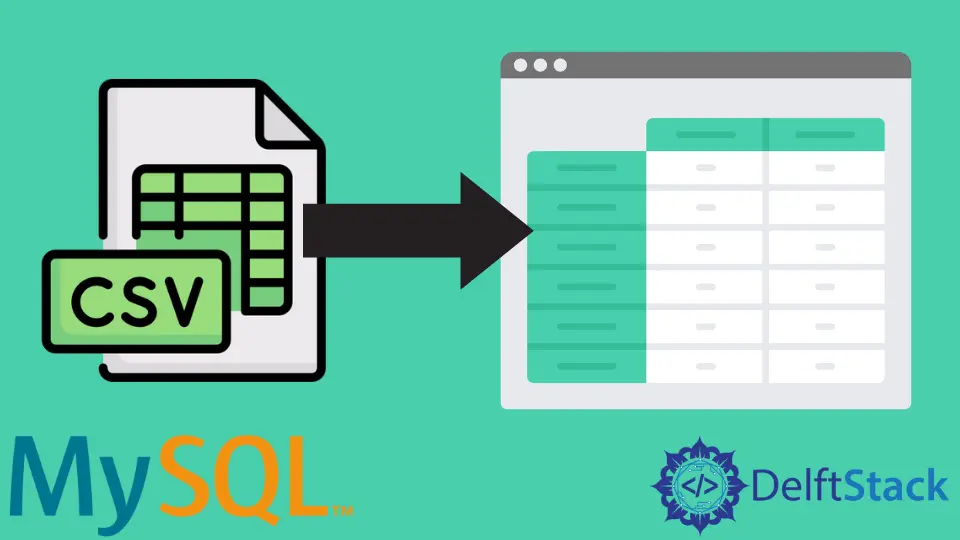
Creating a table from a CSV file in MySQL can streamline your data management process significantly. Whether you’re importing data from a spreadsheet or transferring it from another database, understanding how to do this efficiently is crucial.
In this tutorial, we’ll walk you through the steps to create a MySQL table directly from a CSV file. We’ll cover the necessary commands and provide clear examples to ensure you grasp the process thoroughly. By the end of this article, you’ll be equipped with all the knowledge you need to import your data seamlessly into MySQL, saving you time and effort while enhancing your database management skills.
Understanding CSV Files and MySQL
CSV, or Comma-Separated Values, is a simple file format used to store tabular data. It’s widely used because it’s easy to create and edit. MySQL, on the other hand, is a powerful relational database management system that allows you to store and manage data effectively. The combination of these two can be incredibly powerful for data analysis and management.
In this guide, we will focus on how to create a table in MySQL from a CSV file. We will explore various methods, ensuring you have a comprehensive understanding of the process.
Method 1: Using MySQL’s LOAD DATA INFILE Command
One of the most efficient ways to import a CSV file into MySQL is by using the LOAD DATA INFILE
command. This command reads the CSV file and inserts the data directly into a specified table.
Here’s how you can do it:
-
Prepare your CSV file: Ensure your CSV file is formatted correctly, with the first row containing the column headers.
-
Create a table in MySQL: You need to create a table that matches the structure of your CSV file.
CREATE TABLE your_table_name (
column1_name datatype,
column2_name datatype,
column3_name datatype
);
- Load the CSV data: Use the following command to import your CSV file.
LOAD DATA INFILE '/path/to/your/file.csv'
INTO TABLE your_table_name
FIELDS TERMINATED BY ','
ENCLOSED BY '"'
LINES TERMINATED BY '\n'
IGNORE 1 ROWS;
Output:
Query OK, 100 rows affected
In this command, replace /path/to/your/file.csv
with the actual path to your CSV file. The FIELDS TERMINATED BY
specifies the delimiter used in your CSV, while ENCLOSED BY
defines how fields are encapsulated. The IGNORE 1 ROWS
part is used to skip the header row of your CSV file.
This method is fast and efficient, especially for large datasets. However, it requires proper permissions on the server, so ensure that your MySQL user has the necessary access rights to read the file.
Method 2: Using MySQL Workbench
If you prefer a graphical interface rather than command-line operations, MySQL Workbench provides a user-friendly way to import CSV files into your database.
-
Open MySQL Workbench and connect to your database.
-
Select your database from the left sidebar.
-
Navigate to the “Table Data Import Wizard”: Right-click on your database and choose “Table Data Import Wizard.”
-
Select your CSV file: Browse to the location of your CSV file and select it.
-
Configure the import settings: MySQL Workbench will guide you through the process of mapping CSV columns to the table columns. You can create a new table or append data to an existing one.
-
Finish the import: Click “Next” and then “Finish” to complete the import process.
Output:
Import completed successfully
Using MySQL Workbench is ideal for those who are less comfortable with command-line tools. The wizard simplifies the process by allowing you to visualize the data and make adjustments as needed. This method is particularly useful for smaller datasets or when you need to perform additional data transformations before importing.
Method 3: Using a Python Script with MySQL Connector
For those who prefer to automate the process or need to handle more complex data manipulations, using a Python script with the MySQL Connector library is an excellent option.
- Install MySQL Connector: If you haven’t already, you need to install the MySQL Connector library.
pip install mysql-connector-python
- Create a Python script: Write a script to read your CSV file and insert the data into MySQL.
import mysql.connector
import csv
connection = mysql.connector.connect(
host='your_host',
user='your_username',
password='your_password',
database='your_database'
)
cursor = connection.cursor()
with open('file.csv', 'r') as file:
csv_reader = csv.reader(file)
next(csv_reader) # Skip header row
for row in csv_reader:
cursor.execute('INSERT INTO your_table_name (column1, column2, column3) VALUES (%s, %s, %s)', row)
connection.commit()
cursor.close()
connection.close()
Output:
100 rows inserted
In this script, replace the placeholders with your actual database credentials and table structure. The csv.reader
function reads the CSV file, and the cursor.execute
method inserts each row into the specified table.
This approach provides flexibility, allowing you to manipulate the data before inserting it into MySQL. It’s particularly useful for larger datasets or when you need to apply transformations to the data during the import process.
Conclusion
Creating a table from a CSV file in MySQL is a straightforward process that can significantly enhance your data management capabilities. Whether you prefer using SQL commands, a graphical interface like MySQL Workbench, or automating the process with Python, there are multiple methods to achieve this task. Each method has its advantages, so choose the one that best suits your needs. With the knowledge gained from this tutorial, you can confidently import your data into MySQL, streamline your workflows, and make the most of your database management system.
FAQ
-
How do I create a table in MySQL from a CSV file?
You can use the LOAD DATA INFILE command, MySQL Workbench, or a Python script to create a table from a CSV file. -
What format should my CSV file be in?
Your CSV file should have the first row as headers matching the table columns, and subsequent rows as data entries. -
Can I import large CSV files into MySQL?
Yes, using the LOAD DATA INFILE command is particularly efficient for importing large datasets. -
Do I need special permissions to import CSV files into MySQL?
Yes, ensure that your MySQL user has the necessary permissions to read files from the server. -
Is it possible to automate the CSV import process?
Yes, using a Python script with MySQL Connector allows you to automate and customize the import process.