Sparse Index in MongoDB
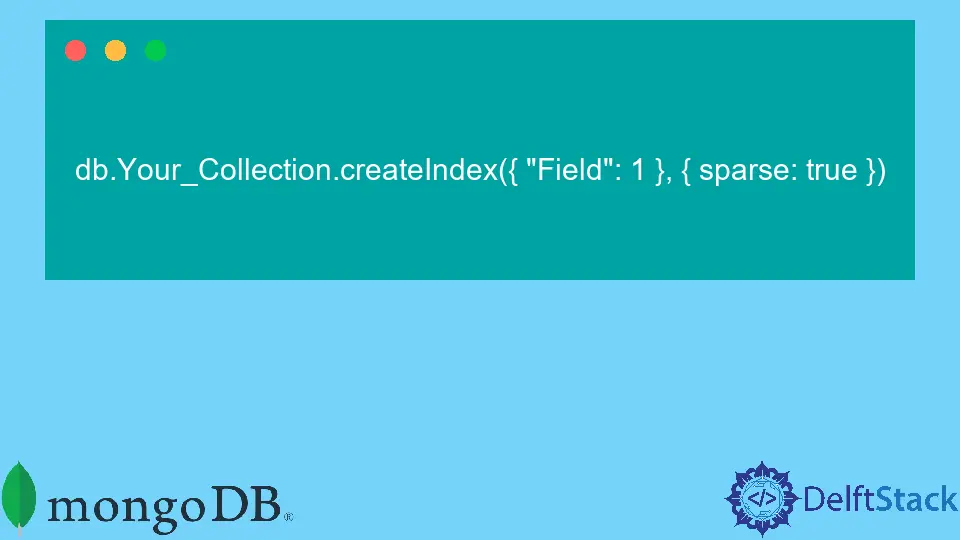
In MongoDB, it is not mandatory that all the documents contain the same fields.
Now, if you want to get all the documents in a MongoDB collection that contain only the specified field, then you may need to specify a common field as an index. This term is known as the Sparse Index.
In this article, we will discuss Sparse Index in MongoDB. Also, we will provide a relevant example with an explanation to make the topic easier.
Sparse Index in MongoDB
The specialty of the Sparse Index is that it only contains documents with the specified indexed field even if the value of the indexed field is null
. If any document doesn’t have the indexed field, it will skip that document.
It is called the Sparse Index because the indexed field doesn’t contain all the documents in a collection.
Create a Sparse Index in a MongoDB Collection
To create a Sparse Index in a MongoDB collection, we need to use the method createIndex()
. The general format for this method is shown below:
db.Your_Collection.createIndex({ "Field": 1 }, { sparse: true })
In our below example command, we will create a Sparse Index with the field Email
in a collection mydata
. The command will be as follows:
db.mydata.createIndex({ "Email": 1 }, { sparse: true })
It is very important to know that if you create a Sparse Index and query based on it, it may skip some of the documents from the collection.
Note: Please check if you are in the right collection. To switch on a specific collection, use the command
use YourDB
; otherwise, it will give an error.
Please note that the commands in this article are for the MongoDB database and need to be run on the MongoDB console.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn