How to Sort by Timestamp in MongoDB
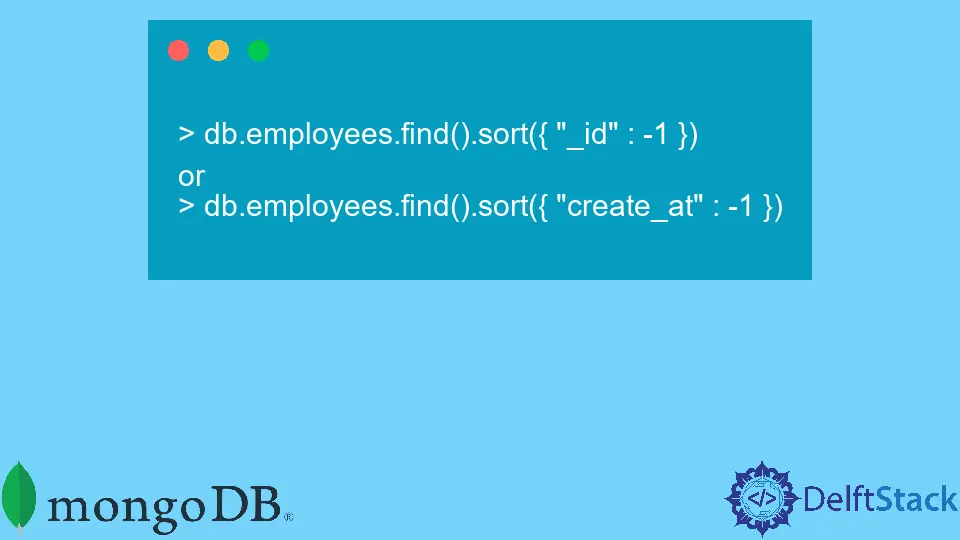
Today’s post will look at many methods to sort timestamps in MongoDB.
Sort by Timestamp in MongoDB
The sort()
method will sort the documents in MongoDB. The method accepts a document with a list of fields and the order in which they should be sorted.
1
and -1
specify the sorting order. For ascending order, use 1
; for descending order, use -1
.
Syntax:
db.collection_name.find().sort({ field_name : 1 | -1 })
In this case, the collection on which you wish to execute sorting is specified by the collection_name
. The field that needs to be sorted in either an ASC
or DESC
order is specified by field_name
.
The sort()
method will display the pages in ascending order if you don’t indicate your preferred sorting order. An unstable sort yields a different result each time it is applied to the same data set.
Utilizing indexes, MongoDB can locate the result of the sort operation. MongoDB performs the top-k
sort algorithm if index scanning cannot determine the sort order.
Consider the following example to help you better understand the prior idea.
> db.employees.find().sort({ "joining_date" : -1 })
According to their joining dates on the employees
collection, we are sorting the employees in the example above. The joining date column keeps track of the employee’s ISO-formatted
joining date.
You can also use _id
because it has a timestamp if you wish to do sorting based on the created_at
field. That will result in the same result.
> db.employees.find().sort({ "_id" : -1 })
or
> db.employees.find().sort({ "create_at" : -1 })
Run the above line of code in MongoShell
, which is compatible with MongoDB. It will display the following outcome:
{
"_id" : ObjectId("54f612b6029b47919a90cesd"),
"email" : "johndoe@exampledomain.com",
"name" : "John Doe",
"create_at" : "ISODate("2020-07-04T00:00:00Z")",
"joining_date" : "ISODate("2020-07-04T00:00:00Z")"
}
{
"_id" : ObjectId("54f612b6029b47919a97cesd"),
"email" : "smithwarn@exampledomain.com",
"name" : "Smith Warn",
"create_at" : "ISODate("2020-04-28T00:00:00Z")",
"joining_date" : "ISODate("2020-04-28T00:00:00Z")"
}
{
"_id" : ObjectId("54f612b6029b47919a91cesd"),
"email" : "jessicawill@exampledomain.com",
"name" : "Jessica Will",
"create_at" : "ISODate("2019-12-14T00:00:00Z")",
"joining_date" : "ISODate("2019-12-14T00:00:00Z")"
}
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn