How to Get the Size of a Database in MongoDB
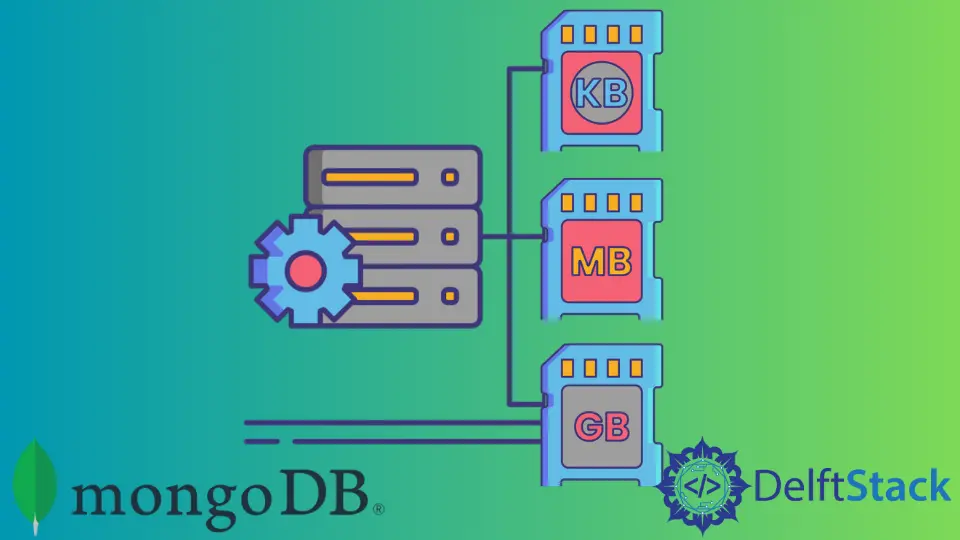
Do you know the size of your database while working in MongoDB? Today, we will use the show dbs
command and db.stats()
method to understand how to get the size of a database in MongoDB.
Get the Size of a Database in MongoDB
We can use the show dbs;
command on the mongo shell to get the storage size of all the databases in MongoDB as follows.
Example Code:
> show dbs;
OUTPUT:
admin 0.000GB
config 0.000GB
local 0.000GB
test 0.001GB
The above output shows the database name with their size in GB. We use the db.stats()
method to get detailed statistics about a database.
It has various fields that we need to understand first. Following is a brief explanation for each of them, but you can find more details about every field here.
db
- the name of the current databasecollections
- number of tables/collections in the current databaseviews
- number of views in the current databaseobjects
- objects’ number in the current database across all the tables (collections)avgObjSize
- average size of every document (a record) in bytesdataSize
- total size of uncompressed data contained by the database. ThedataSize
decreases when we remove a document.storageSize
- is the data’s size stored on thefilesystem
. ThedataSize
can be bigger than thestorageSize
if the compression is used.indexes
- number of indexes in the database across all collectionsindexSize
- the sum of free index space and allocated space to all indexes in the databasetotalSize
- the sum of allocated space for indexes and documents for all collections in a database. It includes free and used storage space.scaleFactor
- a value that is used by the commandfsUsedSize
andfsTotalSize
- these are all about thefilesystem
where the database is stored. These are used to get an idea of how much a database can grow.ok
- the value 1 means the query is executed successfully
Remember that we must select a database before using the db.stats()
function.
Example Code:
> use test
> db.stats()
OUTPUT:
{
"db" : "test",
"collections" : 5,
"views" : 0,
"objects" : 15,
"avgObjSize" : 99,
"dataSize" : 1485,
"storageSize" : 135168,
"indexes" : 5,
"indexSize" : 102400,
"totalSize" : 237568,
"scaleFactor" : 1,
"fsUsedSize" : 176960913408,
"fsTotalSize" : 208499617792,
"ok" : 1
}
We can also pass the scaling factor according to the project requirements.
- No scaling factor is passed if we want the statistics in bytes.
- Pass
1024
to thestats()
method to get statistics in Kilobytes (KB). - Pass
1024 * 1024
to thestats()
function to retrieve the statistics in Megabytes (MB). - Pass
1024 * 1024 * 1024
to thestats()
method to see the statistics in Gigabytes (GB).
See the following examples as a demonstration.
Get Statistics in bytes:
>db.stats()
OUTPUT:
{
"db" : "test",
"collections" : 5,
"views" : 0,
"objects" : 15,
"avgObjSize" : 99,
"dataSize" : 1485,
"storageSize" : 135168,
"indexes" : 5,
"indexSize" : 102400,
"totalSize" : 237568,
"scaleFactor" : 1,
"fsUsedSize" : 176986320896,
"fsTotalSize" : 208499617792,
"ok" : 1
}
The database takes up 0.1352 MB
on filesystem
considering the storageSize
given above. Similarly, we can get the size of a database in the following examples.
Get Statistics in KB:
> db.stats(1024)
OUTPUT:
{
"db" : "test",
"collections" : 5,
"views" : 0,
"objects" : 15,
"avgObjSize" : 99,
"dataSize" : 1.4501953125,
"storageSize" : 132,
"indexes" : 5,
"indexSize" : 100,
"totalSize" : 232,
"scaleFactor" : 1024,
"fsUsedSize" : 172839080,
"fsTotalSize" : 203612908,
"ok" : 1
}
Get statistics in MB:
> db.stats(1024*1024)
OUTPUT:
{
"db" : "test",
"collections" : 5,
"views" : 0,
"objects" : 15,
"avgObjSize" : 99,
"dataSize" : 0.0014162063598632812,
"storageSize" : 0.12890625,
"indexes" : 5,
"indexSize" : 0.09765625,
"totalSize" : 0.2265625,
"scaleFactor" : 1048576,
"fsUsedSize" : 168788.66796875,
"fsTotalSize" : 198840.73046875,
"ok" : 1
}
Get statistics in GB:
> db.stats(1024*1024*1024)
OUTPUT:
{
"db" : "test",
"collections" : 5,
"views" : 0,
"objects" : 15,
"avgObjSize" : 99,
"dataSize" : 0.0000013830140233039856,
"storageSize" : 0.000125885009765625,
"indexes" : 5,
"indexSize" : 0.000095367431640625,
"totalSize" : 0.00022125244140625,
"scaleFactor" : 1073741824,
"fsUsedSize" : 164.83288955688477,
"fsTotalSize" : 194.18040084838867,
"ok" : 1
}