How to List All Collections in the MongoDB Shell
-
Use the
show collections
Command to List All Collections in the MongoDB Shell -
Use the
listCollections
Command to List All Collections in the MongoDB Shell -
Use the
db.getCollectionNames()
Method to List All Collections in the MongoDB Shell -
Use the
db.getCollectionInfos()
Method to List All Collections in the MongoDB Shell
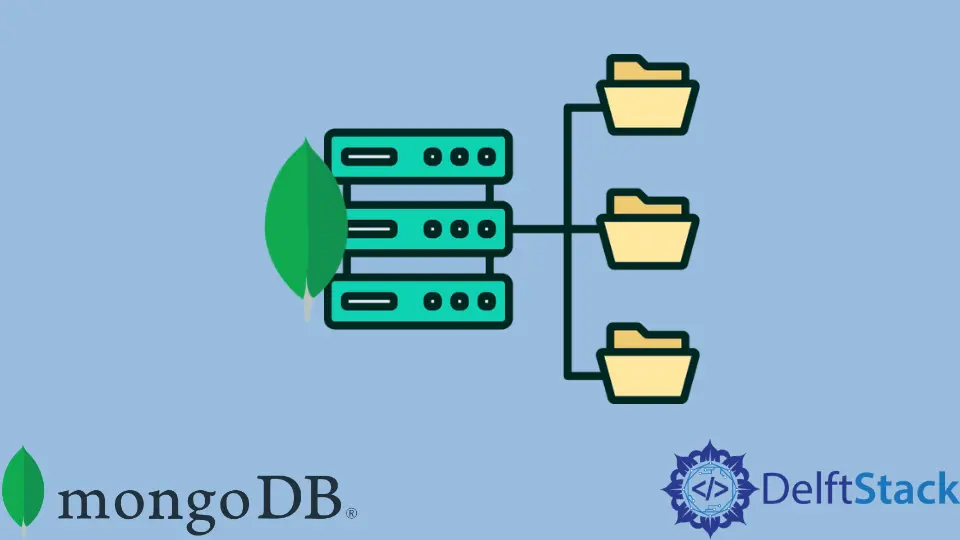
There are several methods for listing collections in a database when using MongoDB. This article will discuss four different methods to get a list of collections in a MongoDB database.
These methods are as follows:
- The
show collections
Command - The
listCollections
Command - The
db.getCollectionNames()
Method - The
db.getCollectionInfos()
Method
Use the show collections
Command to List All Collections in the MongoDB Shell
You will use the Mongo shell command show collections
to get a list of MongoDB collections. This command produces a list of all collections you create in a MongoDB database.
It would be best if you first chose a database in which at least one collection is present to use the command.
Let’s use the use
command to choose a database.
use <BlocTAKdatabase>
After that, you can run the command using the show collections
query.
show collections
The result for the query above is given below.
employees
clients
clienttype
services
system.views
In this instance, there are five outcomes. clienttype
is a view, albeit you can’t tell by looking at it.
The remaining items are collections. system.views
is a system collection that contains information about each database view.
Your access level will determine the output of the collection returned.
- For users with the required access,
show collections
list the database’s non-system collections. - For users without the required access,
show collections
lists only the collections for which the users have privileges.
Use the listCollections
Command to List All Collections in the MongoDB Shell
The administrator command listCollections
returns the name and options of all collections and views in the database. The produced data is in the form of a document.
The query for this command is given below.
db.runCommand( { listCollections: 1, authorizedCollections: true, nameOnly: true } )
The result for the above query is given in the screenshot below.
The document contains information to create a cursor for the collection of information. You can also run the command like this:
db.runCommand( { listCollections: 1.0 } )
Using this provides a lot of information about the collections. Check out the db.getCollectionInfos()
example below to see the data returned when running it like that.
Use the db.getCollectionNames()
Method to List All Collections in the MongoDB Shell
The function db.getCollectionNames()
returns an array with the names of all collections and views in the current database. Besides, if running with access control, the names of the collections are according to the user’s privilege.
The query for this method is given below.
db.getCollectionNames()
The result for the query above is given below.
[ "employees", "clients", "clienttype", "services", "system.views" ]
Use the db.getCollectionInfos()
Method to List All Collections in the MongoDB Shell
The db.getCollectionInfos()
method produces an array of documents containing information about the current database’s collection of views, such as name and options. Again, it determines the outcome by the user’s level of privilege.
Here you have a query for calling it without any arguments.
db.getCollectionInfos()
The result for the above query is given in the screenshot below.
The real definition of db.getCollectionInfos()
is as follows:
db.getCollectionInfos(filter, nameOnly, authorizedCollections)
As a result, you can use the filter
argument to narrow down the collection list using a query phrase. You use this on any field that the method returns.
The nameOnly
argument can specify that the method should only return the names of the collections.
While used with nameOnly: true
, the authorizedCollections
argument allows users to apply without the required privilege to run the command with access control. In this scenario, the command only returns collections to which the user has access.
Here’s a query of using db.getCollectionInfos()
with these parameters.
db.getCollectionInfos( {}, true, true )
The result for the above query is given in the screenshot below.
Here’s an example of a query where you can filter it to just a specific name.
db.getCollectionInfos( { name: "clients" }, true, true )
The result for the query above is given below.
[ { "name" : "clients", "type" : "collection" } ]
And here’s what happens when you remove the last two arguments.
db.getCollectionInfos( { name: "clients" } )
The result for the query above is given below.
[
{
"name" : "clients",
"type" : "collection",
"options" : {
},
"info" : {
"readOnly" : false,
"uuid" : UUID("91d1c6d8-8516-455d-a3c2-b157e1998f8c")
},
"idIndex" : {
"v" : 2,
"key" : {
"_id" : 1
},
"name" : "_id_"
}
}
]
So, using this MongoDB article, you can now use four different methods to list all the collections present in a MongoDB database. These methods are the show collections
command, the listCollections
command, the db.getCollectionNames()
method, and the db.getCollectionInfos()
method.