MongoDB $Set Operator
-
MongoDB
$set
Operator - Set Top-Level Fields
- Set Fields in Embedded Documents
- Set Elements in Arrays
-
$set
(Aggregation) -
Use Two
$set
Stages - Add Fields to an Embedded Document
- Overwrite an Existing Field
- Add Element to an Array
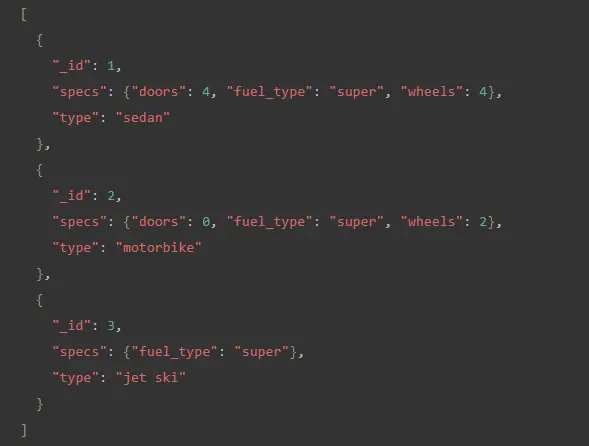
Through the help of this article, you will learn how to use the $set
operator to partially update an object in MongoDB so the new object will overlay/merge with the existing one.
The $set
operator replaces the value of a field with the given value. The $set
operator expression uses the following syntax.
{ $set: { <field1>: <value1>, ... } }
You will specify a <field>
in an embedded document or an array using the dot notation.
MongoDB $set
Operator
If the field does not exist, the $set
operator creates one with the specified value, as long as the new field does not violate any constraint.
For example, if you give a dotted path for a field that does not exist, the $set
operator will generate the embedded documents as needed to complete the dotted path.
In addition, the $set
operator will update or create each field if you specify multiple field-value pairs.
You can create the following products
collection.
db={
"products": [
{
_id: 100,
quantity: 250,
instock: true,
reorder: false,
details: {
model: "PTI",
make: "Breakout"
},
tags: [
"jeans",
"clothing"
],
ratings: [
{
by: "CustomerIK",
rating: 3
}
]
}
]
}
Set Top-Level Fields
For the document matching the criteria _id
equals 100
, the operation below uses the $set
operator to update the value of the details
field, quantity
field, and tags
field. The query for this is given below.
db.products.update(
{ _id: 100 },
{ $set:
{
quantity: 500,
details: { model: "2600", make: "Outfitters" },
tags: [ "coats", "outerwear", "clothing" ]
}
}
)
The operation above updates the following.
- value of
quantity
to500
. details
field with new embedded documenttags
field with a new array
The result for the query above is shown in the screenshot below.
Set Fields in Embedded Documents
You will use dot notation to specify a <field>
in an embedded document or an array. For the document that matches the criteria _id
equal to 100
, the following operation updates the make
field in the details
document.
The query for this is given below.
db.products.update(
{ _id: 100 },
{ $set: { "details.make": "Breakout Kids" } }
)
The result for the query above is shown in the screenshot below.
Set Elements in Arrays
The following action modifies the value in the second element (array index of 1
) of the tags
field and the rating
field in the first element (array index of 0
) of the ratings
array for the document meeting the criteria _id
equal to 100
.
The query for this is given below.
db.products.update(
{ _id: 100 },
{ $set:
{
"tags.1": "wind breaker",
"ratings.0.rating": 2
}
}
)
The result for the query above is shown in the screenshot below.
$set
(Aggregation)
$set
(aggregation) adds new fields to a document. $set
outputs documents containing all existing fields from the input documents and newly added fields.
$set
(aggregation) has the following syntax.
{ $set: { <newField>: <expression>, ... } }
$set
is a command that adds new fields to an existing document. The user can include one or more $set
stages in an aggregation operation.
Use the dot notation to add fields or fields to embedded documents (including documents in arrays). Use $concatArrays
to add an element to an existing array field using $set
.
Use Two $set
Stages
Create a sample grades
collection with the following.
db={
"grades": [
{
_id: 1,
student: "Ali",
homework: [10, 8, 9],
quiz: [9, 8],
extraCredit: 3
},
{
_id: 2,
student: "Manahil",
homework: [3, 8, 5],
quiz: [7, 9],
extraCredit: 6
}
]
}
Two $set
stages are used in the following process to add three new fields to the output documents. The query for this is given below.
db.grades.aggregate( [
{
$set: {
totalHomework: { $sum: "$homework" },
totalQuiz: { $sum: "$quiz" }
}
},
{
$set: {
totalScore: { $add: [ "$totalHomework", "$totalQuiz", "$extraCredit" ] } }
}
] )
The operation returns the following documents, as shown in the screenshot below.
Add Fields to an Embedded Document
Create a sample collection transport
with the following.
db={
"transport": [
{
_id: 1,
type: "sedan",
specs: { doors: 4, wheels: 4}
},
{
_id: 2,
type: "motorbike",
specs: {doors: 0, wheels: 2}
},
{
_id: 3,
type: "jet ski"
}
]
}
The following aggregation operation creates a new field fuel_type
in the embedded document specs
. The query for this is given below.
db.transport.aggregate( [
{ $set: { "specs.fuel_type": "super" } }
] )
The operation returns the following results, as shown in the screenshot below.
Overwrite an Existing Field
When a $set
operation specifies an existing field name, the original field is replaced. Make a pets
sample collection with the following items.
db={
"pets": [
{
_id: 1,
dogs: 10,
cats: 5
}
]
}
The $set
operation that follows, overrides the cats
field. The query for this is given below.
db.pets.aggregate( [
{ $set: { "cats": 20 } }
] )
The operation returns the following document, as shown in the screenshot below.
Add Element to an Array
Create a sample grades
collection with the following records.
db={
"grades": [
{
_id: 1,
student: "Ali",
homework: [10, 8, 9],
quiz: [9, 8],
extraCredit: 3
},
{
_id: 2,
student: "Manahil",
homework: [3, 8, 5],
quiz: [7, 9],
extraCredit: 6
}
]
}
The user can use the $set
operator with a $concatArrays
expression. This adds an element to an existing array field.
The following operation uses $set
to replace the homework
field with a new array whose elements are the current homework
array concatenated with another array containing a new score [ 7 ]
. The query for this is given below.
db.grades.aggregate([
{ $match: { _id: 2 } },
{ $set: { homework: { $concatArrays: [ "$homework", [ 7 ] ] } } }
])
The operation returns the following document, as shown in the screenshot below.
In this article, you have learned how to use the $set
operator to partially update an object in MongoDB so that the new object will overlay/merge with the existing one.