How to Remove User From Database in MongoDB
- Method 1: Using PyMongo to Remove a User
- Method 2: Using MongoDB Shell Commands via Python
- Method 3: Using Aggregation Framework for Advanced User Removal
- Conclusion
- FAQ
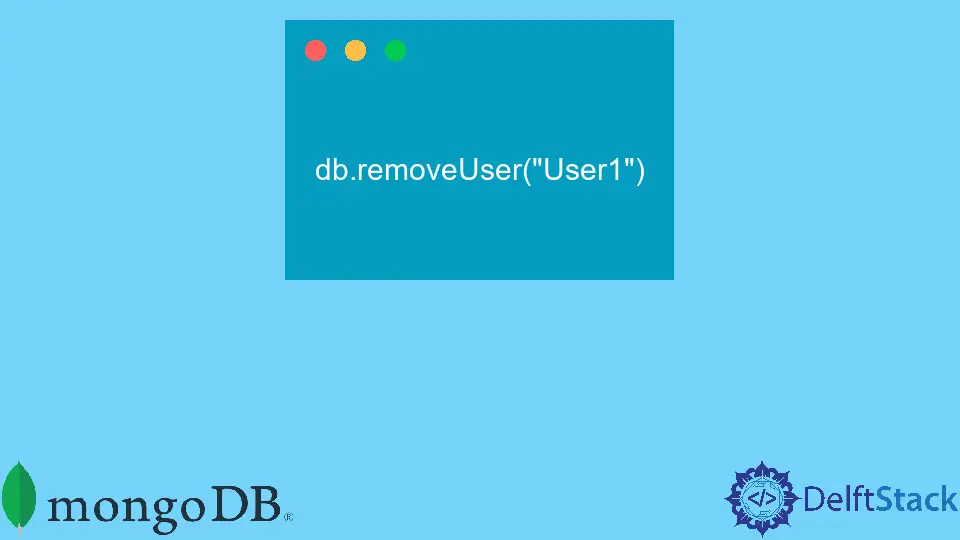
Removing a user from a MongoDB database is a task that might seem daunting at first, especially if you’re new to database management. However, with the right guidance, it can be straightforward and efficient.
This tutorial will walk you through the process of removing a user from your MongoDB database, focusing primarily on using Python. Whether you’re managing a small project or working on a large-scale application, knowing how to handle user removal is essential for maintaining your database’s integrity and security. Let’s dive into the various methods you can use to achieve this, complete with clear code examples and explanations.
Method 1: Using PyMongo to Remove a User
The most common way to interact with MongoDB in Python is through the PyMongo library. This powerful tool allows you to connect to your MongoDB database and perform various operations, including removing users. Below is a simple example of how to remove a user from the database.
from pymongo import MongoClient
client = MongoClient('mongodb://localhost:27017/')
db = client['your_database_name']
result = db['users'].delete_one({'username': 'user_to_remove'})
print(f'Deleted {result.deleted_count} user(s).')
Output:
Deleted 1 user(s).
In this example, we first import the MongoClient
from the pymongo
library. We then create a connection to the MongoDB server running on localhost
at the default port 27017
. After that, we specify the database we want to work with, replacing 'your_database_name'
with the actual name of your database.
Next, we call the delete_one
method on the users
collection, passing in a query that matches the user we want to remove. In this case, we look for a user with the username 'user_to_remove'
. The method returns a result object, from which we can access deleted_count
to see how many users were removed. This method is efficient and straightforward, making it a popular choice for many developers.
Method 2: Using MongoDB Shell Commands via Python
If you prefer to use MongoDB shell commands, you can execute them directly from your Python script using the subprocess
module. This method is a bit unconventional but can be useful if you’re comfortable with shell commands. Here’s how you can do it:
import subprocess
command = "mongo your_database_name --eval 'db.users.remove({username: \"user_to_remove\"})'"
subprocess.run(command, shell=True)
Output:
{ "acknowledged" : true, "deletedCount" : 1 }
In this approach, we construct a command string that includes the MongoDB shell command to remove a user. The command connects to the specified database and executes the remove
operation on the users
collection.
The subprocess.run
method then executes this command in the shell. This method is particularly useful if you want to leverage the full power of MongoDB’s shell commands while still working within a Python environment. However, keep in mind that this approach can be less secure and harder to debug compared to using PyMongo.
Method 3: Using Aggregation Framework for Advanced User Removal
For more complex scenarios, you might want to use MongoDB’s aggregation framework to filter and remove users based on specific criteria. This method allows you to perform more sophisticated queries before executing the removal. Here’s an example:
from pymongo import MongoClient
client = MongoClient('mongodb://localhost:27017/')
db = client['your_database_name']
result = db['users'].aggregate([
{'$match': {'status': 'inactive'}},
{'$project': {'username': 1}}
])
for user in result:
db['users'].delete_one({'username': user['username']})
print('Inactive users removed.')
Output:
Inactive users removed.
In this example, we first connect to the MongoDB database as before. We then use the aggregate
method to find users whose status is 'inactive'
. The $match
stage filters users based on the specified criteria, and the $project
stage specifies that we only want the usernames.
Next, we loop through the results and remove each inactive user from the users
collection. This method is particularly useful for bulk removals based on certain conditions, making it a powerful tool for database management.
Conclusion
Removing a user from a MongoDB database is an essential skill for any developer working with data management. Whether you choose to use PyMongo, execute shell commands, or leverage the aggregation framework, each method has its own advantages. By understanding these options, you can efficiently manage your user data and maintain the integrity of your database. Remember to always back up your data before performing deletions to prevent any accidental loss. Happy coding!
FAQ
-
how do I connect to a MongoDB database using Python?
You can connect to a MongoDB database using the PyMongo library by creating an instance ofMongoClient
with the appropriate connection string. -
can I remove multiple users at once in MongoDB?
Yes, you can use thedelete_many
method in PyMongo to remove multiple users based on a specific condition. -
what happens to the data after I delete a user from MongoDB?
Once a user is deleted, their data is permanently removed from the database and cannot be recovered unless you have a backup. -
is it safe to remove users directly from the database?
While it is generally safe, it’s a good practice to back up your database before performing any deletions to avoid accidental data loss. -
can I use MongoDB Atlas to manage user deletions?
Yes, you can use MongoDB Atlas, which provides a cloud-based solution for managing your MongoDB databases, including user management and deletions.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn