How to Remove Duplicates in MongoDB
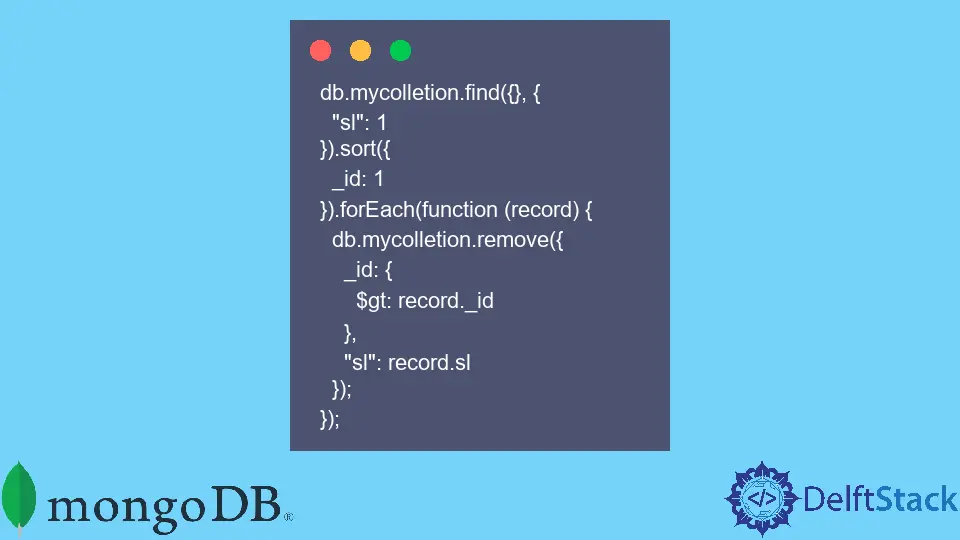
You may be familiar with duplicate entries if you are a database administrator. Duplicate entries are a common problem in database management.
In this article, we are going to see how we can remove duplicate entries in MongoDB, and also we are going to see an example with a proper explanation to make the topic easier.
Remove Duplicate Documents From the Collection in MongoDB
We need to divide the task into two parts to remove duplicate entries from the database. First of all, we need to sort the documents of a collection based on specific criteria, and after that, we need to define a function for each document using the method forEach()
.
Inside the function, we need to use a method named remove()
, which will remove the duplicate document. Now let’s take a look at an example to make it clear.
In our example below, we will demonstrate how we can remove duplicate entries from our collections. Now before we start, let’s create some duplicate documents intensionally like the below:
{
"sl": 1,
"Name": "Alex"
}
{
"sl": 1,
"Name": "Alex"
}
Now we are going to execute the below command in the MongoDB console:
db.mycolletion.find({}, {
"sl": 1
}).sort({
_id: 1
}).forEach(function (record) {
db.mycolletion.remove({
_id: {
$gt: record._id
},
"sl": record.sl
});
});
We already described the working mechanism of the above command in our introduction part. After executing the command successfully, you will get the below output in your console window.
{
"sl": 1,
"Name": "Alex"
}
Remember, you need to switch to your database if you are not in your targeted database. To do this, use the command below:
use Your_DB
Please note that the commands shown in this article are for the MongoDB database and the command needs to be run on the MongoDB console.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn