How to Remove Item by ID in MongoDB
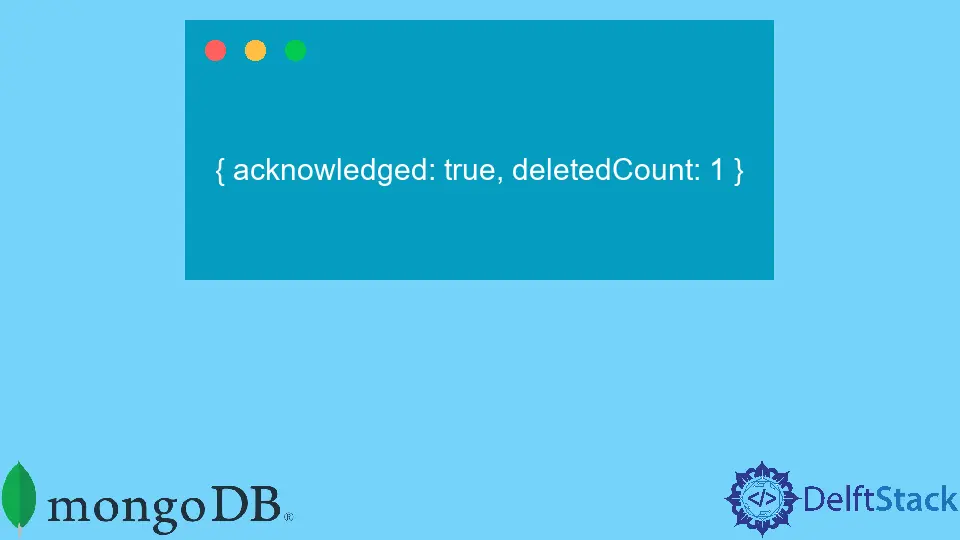
Sometimes we need to delete data in our database based on specified criteria. MongoDB doesn’t contain SQL queries for this purpose, unlike other SQL databases. Instead, it uses commands.
This article will discuss how to delete a document based on specific criteria like ID. Also, we will look at an example with explanations regarding the topic to make it easier.
Delete a Document by ID in MongoDB
MongoDB contains a built-in method named deleteOne()
for performing delete operations. This function will take various criteria as a parameter.
The general syntax for this method is below.
db.Your_Collection.deleteOne({ Your_Criteria_Here })
As we are willing to delete a document based on its ID, we can update the syntax as follows:
db.mycollection.deleteOne( {"_id": ObjectId("Your_ID_Here")});
Now, take a look at an example relevant to this topic.
Remember you need to switch to your database if you are not in your targeted database. To do this, use the command:
use Your_DB
.
In our example below, we will illustrate how we can delete a document based on the specified ID. To do this, let’s create some documents inside our collection first.
{
"_id": ObjectId("6371fd850f19826ee6ca5139")
"sl": 1,
"Name": "Alex"
}
{ _id: ObjectId("6371fd850f19826ee6ca5138"),
sl: 0,
Name: 'Alen'
}
Now, let’s delete a document with the id 6371fd850f19826ee6ca5139
. To do this, we will use the command as follows:
db.mycollection.deleteOne( {"_id": ObjectId("6371fd850f19826ee6ca5139")});
After executing the above command, you will get an output like the one below.
{ acknowledged: true, deletedCount: 1 }
{ _id: ObjectId("6371fd850f19826ee6ca5138"),
sl: 0,
Name: 'Alen'
}
Please note that the commands shown in this article are for the MongoDB database and the command needs to be run on the MongoDB console.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn