How to Query With String Length in MongoDB
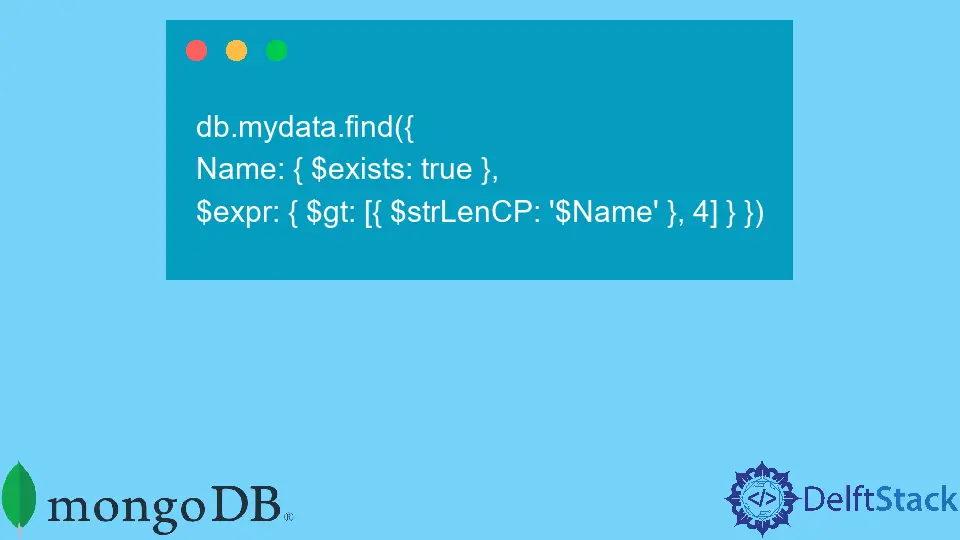
Sometimes, we need to find a specific document using exceptional properties like string length. In this article, we are going to see how we can find specific documents using the string length in MongoDB and also we will see an example regarding the topic to make it easy to understand.
Find a Specific Document in a Collection Based on String Length
To find a specific document from a collection, we use a very common built-in method of MongoDB named find()
. But we need to provide some exceptional criteria when we are willing to find a document based on the string length.
In our next example, we will see two ways to find a specific document based on string length.
In our example below, we will see how we can find a document based on string length.
But first, let’s check the documents available in our collection. For this purpose, we are going to use the below command:
db.mydata.find()
The above command will show you all the documents in the mydata
collection.
{ _id: ObjectId("63713371117701ff3d627b56"),
Name: 'Alen',
Email: 'abc@gmail.com',
Year: 2018 }
{ _id: ObjectId("63713371117701ff3d627b57"),
Name: 'Max',
Email: 'max@gmail.com',
Year: 2017 }
{ _id: ObjectId("63713371117701ff3d627b58"),
Name: 'Ethen',
Email: 'ethen@gmail.com',
Year: 2019 }
{ _id: ObjectId("63713371117701ff3d627b59"),
Name: 'Alex',
Email: 'alex@gmail.com',
Year: 2020 }
Above are the documents available in our collection. Let’s go to our next step.
Now let’s see the methods we can use to find the document based on string length. For this purpose, we will take the length of the Name
field.
Use the $where
Keyword
We can use the keyword $where
for this purpose. The command will be as follows:
db.mydata.find({ $where: 'this.Name.length > 4' })
Use the Aggregation
If you want to use the aggregation, then you can follow the below command:
db.mydata.find({
Name: { $exists: true },
$expr: { $gt: [{ $strLenCP: '$Name' }, 4] } })
On the command above, the keyword $gt
means Greater Than. Now, after executing both of the commands in the MongoDB console, you will get the same output as follows:
{ _id: ObjectId("63713371117701ff3d627b58"),
Name: 'Ethen',
Email: 'ethen@gmail.com',
Year: 2019 }
Please note that the commands shown in this article are for the MongoDB database and the command needs to be run on the MongoDB console.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn