How to Query Nested Object in MongoDB
- Understanding Nested Objects in MongoDB
- Querying Nested Objects Using Dot Notation
- Using the $elemMatch Operator for Arrays
- Querying Nested Objects with the Aggregation Framework
- Conclusion
- FAQ
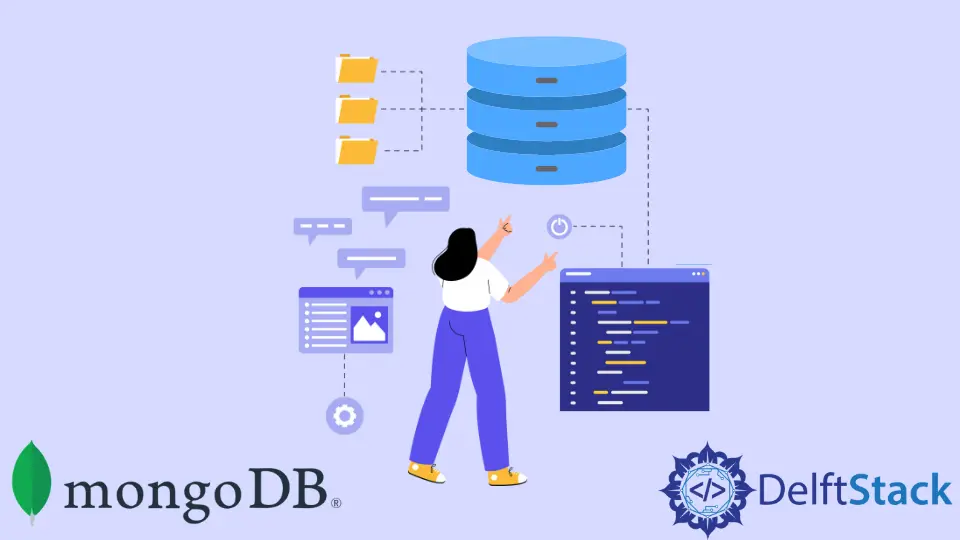
MongoDB is a powerful NoSQL database that allows you to store data in flexible, JSON-like documents. One of its standout features is the ability to handle complex data structures, including nested objects. Querying these nested objects can initially seem daunting, but with the right techniques, it becomes a straightforward task.
In this article, we’ll explore various methods to query nested objects in MongoDB, focusing on practical examples that you can easily replicate in your projects. Whether you’re a beginner or an experienced developer, understanding how to work with nested objects will enhance your MongoDB skills and improve your data retrieval capabilities.
Understanding Nested Objects in MongoDB
Before diving into querying, it’s essential to grasp what nested objects are. In MongoDB, a nested object is a document within a document. This structure allows for a hierarchical organization of data, making it easier to represent complex relationships. For example, consider a collection of users where each user has an address object containing street, city, and zip code. To query such structures effectively, you need to understand how to access and filter these nested fields.
Querying Nested Objects Using Dot Notation
MongoDB allows you to query nested objects using dot notation, which is a straightforward way to access the properties of a nested document. For instance, if you have a collection named users
and you want to find users living in a specific city, you can write a query like this:
db.users.find({"address.city": "New York"})
Output:
{ "_id": 1, "name": "John Doe", "address": { "street": "123 Main St", "city": "New York", "zip": "10001" } }
{ "_id": 2, "name": "Jane Smith", "address": { "street": "456 Elm St", "city": "New York", "zip": "10001" } }
This command retrieves all users whose address.city
field matches “New York”. The use of dot notation allows you to specify the path to the nested field directly, making your queries concise and readable.
By leveraging dot notation, you can easily filter documents based on nested properties. This approach is particularly useful in applications where data is structured hierarchically, enabling you to access specific information without retrieving unnecessary data.
Using the $elemMatch Operator for Arrays
When dealing with nested objects that contain arrays, the $elemMatch
operator comes in handy. It allows you to query for documents that contain an array of objects and filter based on the properties of those objects. Suppose you have a collection of products, each with an array of reviews. To find products with a review rating of 5 stars, you can use the following query:
db.products.find({
"reviews": {
"$elemMatch": {
"rating": 5
}
}
})
Output:
{ "_id": 1, "name": "Product A", "reviews": [{ "rating": 5, "comment": "Excellent!" }, { "rating": 4, "comment": "Very good." }] }
{ "_id": 2, "name": "Product B", "reviews": [{ "rating": 5, "comment": "Loved it!" }] }
This query retrieves all products that have at least one review with a rating of 5. The $elemMatch
operator ensures that you only get documents that meet your specified criteria within the array. This is particularly useful in scenarios where you need to filter based on multiple criteria within an array of objects.
Querying Nested Objects with the Aggregation Framework
For more complex queries involving nested objects, the MongoDB Aggregation Framework is a powerful tool. It allows you to perform operations like filtering, grouping, and projecting data in a more sophisticated manner. Let’s say you want to calculate the average rating of products based on their reviews. You can achieve this with the following aggregation pipeline:
db.products.aggregate([
{
"$unwind": "$reviews"
},
{
"$group": {
"_id": "$name",
"averageRating": { "$avg": "$reviews.rating" }
}
}
])
Output:
{ "_id": "Product A", "averageRating": 4.5 }
{ "_id": "Product B", "averageRating": 5.0 }
In this example, the $unwind
stage deconstructs the reviews
array, creating a separate document for each review. Then, the $group
stage calculates the average rating for each product. This method is particularly useful when you need to perform calculations or aggregations on nested data, enabling you to extract meaningful insights from your MongoDB collections.
Conclusion
Querying nested objects in MongoDB may seem complex at first, but with the right techniques, it can be a seamless process. Whether you’re using dot notation for simple queries, the $elemMatch
operator for arrays, or the Aggregation Framework for more complex operations, you have the tools at your disposal to retrieve the data you need efficiently. As you continue to work with MongoDB, mastering these querying methods will significantly enhance your ability to manage and analyze your data effectively.
FAQ
-
What is a nested object in MongoDB?
A nested object in MongoDB is a document that contains another document as a field, allowing for hierarchical data representation. -
How can I access nested fields in MongoDB?
You can access nested fields using dot notation, allowing you to specify the path to the desired field.
-
What is the purpose of the $elemMatch operator?
The $elemMatch operator is used to query arrays of objects, filtering documents based on specific criteria within the array elements. -
How does the Aggregation Framework work in MongoDB?
The Aggregation Framework processes data records and returns computed results, allowing for complex queries, transformations, and calculations on your data. -
Can I update nested objects in MongoDB?
Yes, you can update nested objects using the dot notation in your update queries, allowing you to modify specific fields within nested documents.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn