How to Pretty Print in MongoDB
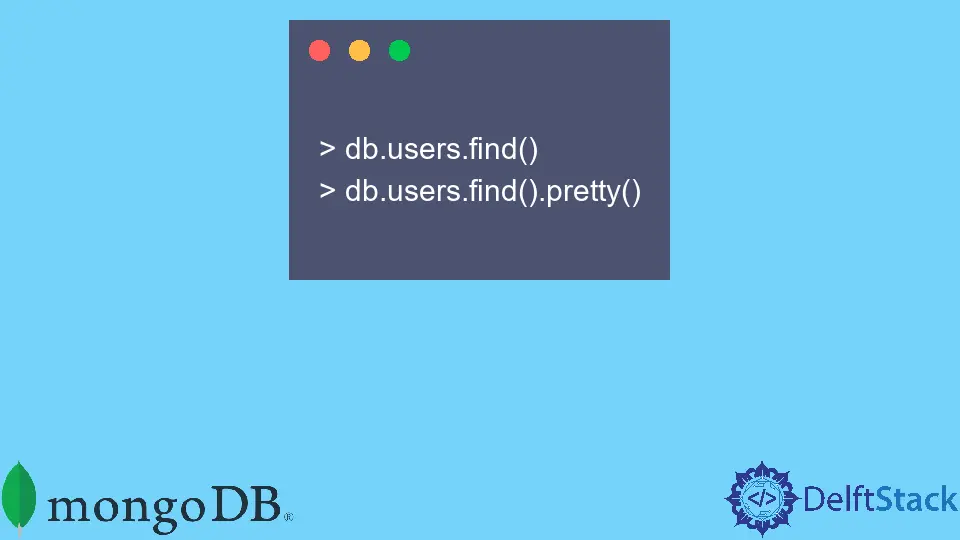
This article will discuss how to display formatted results using pretty print in MongoDB.
Pretty Print in MongoDB
A cursor
is an object that lets programmers iterate through the documents in a Mongo collection in the world of Mongo. Although developers can explicitly traverse through the items returned in the cursor
object, the functionality of the cursor
permits an automatic iteration across the query results.
The pretty()
method in the Mongo universe defines the cursor
object to show the Mongo query results in a readable, attractive layout. This strategy is quite helpful if our database is large.
Syntax:
> db.collectionName.find(queryString).pretty()
The queryString
is a choice input argument that may be used to get documents from a collection according to given selection criteria.
The JSON documents or collections inside the Mongo Shell can be more attractive using the cursor.pretty()
method. The Mongo Shell does not always like this approach.
You can find more information about the .pretty() documentation in the .pretty()
. Let’s use the following example to understand the mentioned idea.
Code:
> db.users.find()
> db.users.find().pretty()
In the preceding sample, we are locating all of the users, pretty approach or not. The main distinction between the two queries is that the latter returns data in a more readable style while the former delivers data in a denser format.
Run the above line of code in MongoShell, which is compatible with MongoDB. It will display the following outcome.
Output:
{ "_id" : ObjectId("54f612b6029b47909a90cesd"), "email" : "johndoe@example.com", "comment" : "This is the first user in the collection.", "country" : "United Kingdom" }
{
"_id" : ObjectId("54f612b6029b47909a90cesd"),
"email" : "johndoe@example.com",
"comment" : "This is the first user in the collection.",
"country" : "United Kingdom"
}
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn